What is Default Constructor in C++?
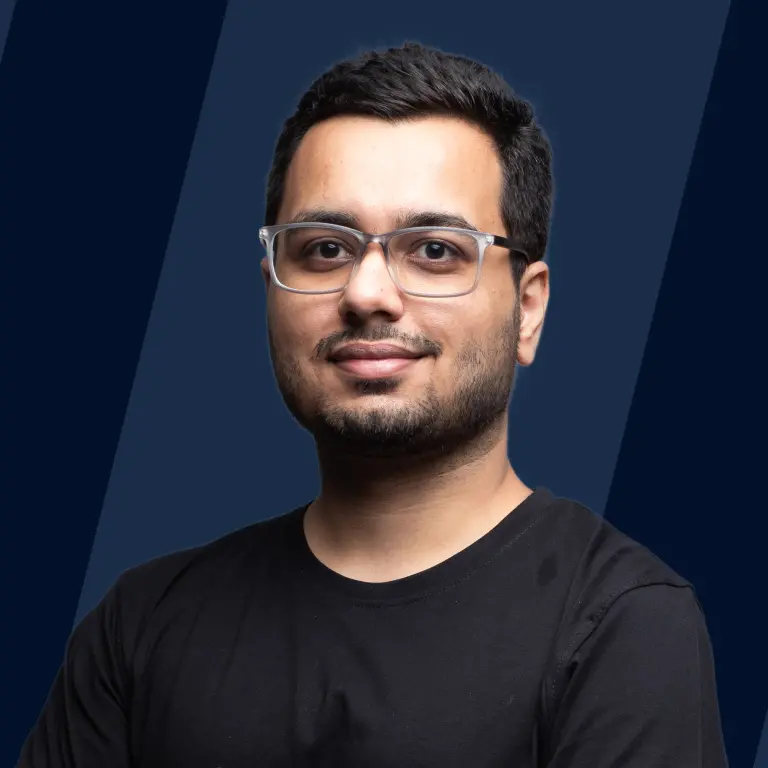
Constructors are special member functions that are invoked implicitly when a class object is created. They share the same name as the class and have no return type. A default constructor in C++ is a type of constructor that is implicitly created by the compiler when no constructor is created explicitly. Default constructors have no parameters or arguments. That’s why they are also called no-argument constructors, and if they contain any parameter, then they should be default parameters with default values.
Syntax
Let's see the syntax used for the declaration of default constructors in C++ inside the class and outside the class, which is as follows:
Syntax for Declaring Default Constructor Inside the class
Code:
Syntax for Declaring Default Constructor Outside the class
Code:
Explanation
The declaration of the default constructor inside the class is made the same way as done for any other member function. The name of the default constructor is the same as the name of its corresponding class, so the compiler can differentiate between basic member functions and a default constructor. The declaration of the default constructor outside the scope of its class is made in the same way as done for any member function by using the scope resolution operator(::).
Features of Default Constructors in C++
Features of the default constructor in C++ are as follows:
- Default constructor in C++ has the same name as its class.
- Default constructor in C++ is implicitly invoked when any object is created.
- Default constructor is used to initialize the values to the data members of an object.
- Default constructors have zero arguments or default parameters.
- They are created by the compiler when no constructors are declared explicitly inside the class.
Types
Let's discuss the types of default constructors in C++. There are a total of five types which are as follows:
Implicitly-Declared Default Constructor
As we discussed earlier in the article, when a constructor is not created explicitly by the user, the compiler creates a constructor, which will be declared as the inline public member of the same class. That constructor is known as an implicitly declared default constructor.
Even if an explicitly declared constructor is present, a user can force the compiler to create a default constructor, which may also be called an implicitly declared default constructor.
Implicitly-Defined Default Constructor
When an implicitly-defined default constructor is not defined, the compiler does its function body creation and compilation. In short, the compiler defines the implicitly-defined default constructor during creation. It works the same way as a user-defined constructor with an empty body. The class that contains empty explicitly defined constructors may work differently than the classes with an implicitly defined default constructor during the initialization of the values.
Deleted Implicitly-Declared Default Constructor
The implicitly declared default can be declared as deleted for a class if any of the conditions listed below are true:
- The class has a member who is of reference type.
- The class has a member that contains a deleted default constructor, or its default constructor may act ambiguous and inaccessible.
- The class has a virtual base or direct base that contains a deleted default constructor, or it may act ambiguous and inaccessible.
- The class has a non-static member or virtual base that contains a deleted destructor, which might also be inaccessible.
Trivial default constructor
A default constructor of a class is said to be trivial if all the conditions listed below are true:
- The constructor of the class is not an explicitly created constructor or user-defined constructor.
- The class has no virtual base class and virtual member functions.
- Each direct base of the class should contain the trivial default constructor.
- Trivial default constructor is present in every non-static member of the class.
Note: A trivial default constructor performs no actions.
Eligible default constructor
A default constructor in C++ is said to be eligible if:
- The constructor is either explicitly defined or implicitly defined.
- The constructor must not be deleted.
- There should be no default constructor, which will have more constraints than it.
- If any constraints are associated with the constructor, then the constraint should be satisfied.
Examples
Now, Let's see some basic examples that will help us understand the default constructor in C++ more clearly.
Example 1:
In the following example, we will see the basic calling of the default constructor in C++ when an object of the same class is created.
Code:
Output:
Example Explanation: Here, we have defined a default constructor, Base(), of the Base class. Then inside the main() method, we have declared or created an object obj1 of the Base class. The default constructor will be called implicitly when the class object is created. The message inside the default constructor Base() will be printed as seen in the output.
Example 2:
In the following example, we will have a default constructor in C++ with a default parameter. As discussed above in the article, default parameters can be used in default constructors.
Code:
Output:
Example Explanation: Here, we have defined a default constructor, Base(), with a default parameter having a default value, i.e., int x = 5. Inside the main() method, we have initialized an object of the class, i.e., b, to invoke the default constructor. The default constructor Base(int x=5) will return or calculate the square of the variable x as seen in the output.
Example 3:
In the following example, we will be using the default constructor in C++ to set or initialize the values of the private data members of a class.
Code:
Output:
Example Explanation: Here, Inside the Base class, we have declared two data members, a and b of integer type. Then we have defined the default constructor Base(), which will initialize the value of the data members. Whenever an object of the Base class has been created, the values of the data members will be initialized implicitly. Inside the main() method, we have created an object obj1 of the Base class. Then we will call the function printValue() to print the value of a and b as shown in the output.
Learn more
Constructors and Destructors: Constructor is the special member function invoked implicitly when a class object is created. While Destructor is a special member function invoked implicitly when an object's scope ends, it is used to free up the resources occupied by an object.
To learn about Constructor and Destructor in detail, refer to Constructor and Destructor in C++ To learn more about classes, objects, and more object-oriented programming concepts, refer to Object Oriented Programming
Conclusion
- Constructor is mainly of three types:**
- Default Constructor
- Parameterized Constructor
- Copy Constructor
- Default constructor in C++ is a type of constructor that is implicitly created by the compiler when no constructor is created explicitly.
- Default constructor has no parameters or arguments. That's why they are also called no-argument constructor
- Default Constructors in C++ are created by the compiler when no constructors are declared explicitly inside the class.
- There are a total of five types of default constructors in C++, which are as follows:
- Implicitly-declared default constructor
- Implicitly-defined default constructor
- Deleted implicitly-declared default constructor
- Trivial default constructor
- Eligible default constructor