Delete a Directory in Python
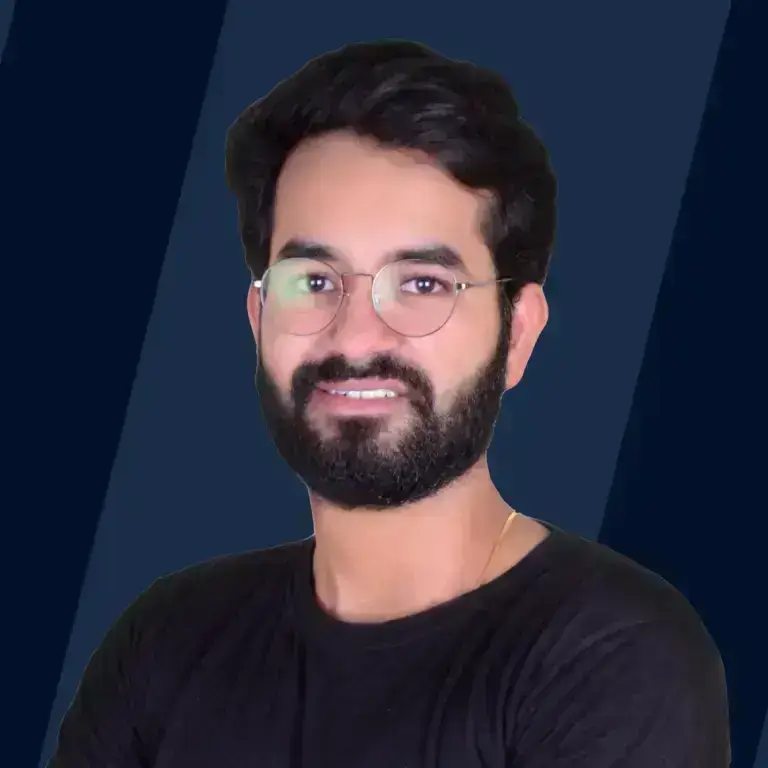
Overview
Python offers various built-in modules and functions that allow us to delete files and directories from our local computers. Deleting files and directories is a critical operation because you cannot easily retrieve directory content once you have deleted the directory. We have various built-in functions by which a user can easily delete a directory in Python. We can delete the directories and files using some functions of the modules os, shutil, and pathlib.
Delete Directory in Python
Python has many built-in modules, such as os, shutil, and pathlib, which are used to delete files and directories from your local drive. If we want to delete empty directories, then we can use the os.rmdir() function because os.rmdir() is used to delete empty directories. The path.rmdir() also helps in removing the empty directory, but we have to create a path object pointing to the directory. Using the shutil.rmtree() function, we can delete the entire directory(including subdirectories under it) from our local system.
Imagine a tree like this:
Say we want to delete the User directory, just do shutil.rmtree('User'). This will delete all the subdirectories (Developer/Tester & Programmer/Bug).
Say we want to delete Tester or Bug directory, just do path.rmdir('Tester') or os.rmdir('Bug'). We can't delete directories that are not empty, like User, Developer, and Programmer.
Methods to Delete Directories in Python
Let’s have a look at the methods to delete directory in Python.
Using os.rmdir() Function:
Python uses the os.rmdir() function to delete an empty directory from your local system, and if the directory is not found, then a FileNotFoundError is raised. If we use the os.rmdir() function, the directory must be empty, otherwise, an OSError will be thrown.
Example:
In the below code, we are deleting the directory 'Scaler' from our local system using the os.rmdir() function. Since the directory with the name 'Scaler' is present in our system and it is also empty , it will be deleted from our system. We just have to pass the absolute path of the directory that we want to delete as an input to the function, and the directory will be deleted from our system. Code:
Output:
But listen, what happens when we want to delete any directory and that directory is not in our local system? It will throw a FileNotFoundError.
Code:
Output:
In the above example, we are trying to access the directory (i.e., the Dummy directory), but since we don't have any directories with this name in our local system, it will throw an error.
Now, what happens if our directory contains some content (which may be files or images)? It will throw an OSError because if we have the os.rmdir() function, the directory should be empty.
Code:
Output:
As we can see in the output, our directory(i.e., the Scaler directory) is not empty, it has some content. That's why our code is throwing an error.
Using pathlib Module:
To delete a file with the pathlib module, create a path object pointing to the file and call the rmdir() method on the object. The pathlib.rmdir() function of the pathlib module is also used to delete an empty directory. We just have to pass the absolute path of the directory that we want to delete as an input to the function, and the directory will be deleted from our system.
Tip: The pathlib module is available in Python 3.4 or above, and if you want to use this module in Python 2, then just install it by using the command: pip install pathlib.
Example: As we have already seen above in the os.rmdir() function, if we want to delete directory in Python and that directory is not in our local system, then it will throw a FileNotFoundError. Also, if our directory contains some content (which may be files or images), then it will throw an OSError.
The below example will show the demonstration of the pathlib module and the pathlib.rmdir() function.
Code:
Output:
As you can see in the above outputs when we run the script for the first time, it will delete the directory(Scaler), and if we run it again, it will throw an error that the directory is not present in the path specified.
Using shutil.rmtree():
The shutil module is used to perform high-level operations on files and directories. Using the shutil.rmtree() function, we can delete the entire directory (including subdirectories under it) from our local system.
Let's say we have a folder and inside that folder, we have some other folders and files also. Then, using the shutil.rmtree() function, we can delete the entire folder and its contents.
Example: We have created a directory named Scaler, and inside this directory, we have some other folders like Content and Topics. When we run the below code, we will see that the entire folder will be deleted with its entire content.
Code:
Output: Before you run the code:
After you run the code:
More Examples for Understanding
Let's have a look at some examples for more understanding of the functions os.rmdir(), pathlib.rmdir(), and shutil.rmtree() and to see how we can easily delete directory in Python.
Example 1: Delete a Directory from a List of Directories.
Suppose we have a list of directories and we want to delete the Content directory.
In the below code, we are using the os.rmdir() function to delete it from our local system because os.rmdir() can only delete the empty directory, and here the 'Content' directory is empty. So we can use the os.rmdir() function to delete the directory easily.
Code:
Output:
As you can see in the above output, the Content directory has been deleted.
Example 2: Handling Error While Deleting Directory.
In the below image, as you can see, we have two directories, Academy and Topics, but the Academy directory has some files and folders. Now let's see what happens when we try to delete the Academy directory.
Code:
Output:
As you can see in the above code, we are trying to delete the directory(Academy). It is throwing an error because it has some content, so we can not delete it.
Example 3: Delete the Directory that has Some Content.
In the above example, we see that we cannot delete the directory that has some content, and if we try to delete it, it will throw an error. Now we will use the shutil.rmtree() function to delete the directory containing content. Using the shutil.rmtree() function, we can delete the entire directory(including subdirectories under it) from our local system.
Let's look at the below code to understand it better.
Code:
Output: Before:
After:
Conclusion:
Now let's conclude our topic "delete directory Python" by mentioning some key takeaways.
- Deleting files and directories is a critical operation because you cannot easily retrieve directory content once you have deleted the directory.
- We can delete the directories and files using some functions of the modules os, shutil, and pathlib.
- Python uses the os.rmdir() function to delete an empty directory from your local system, and if the directory is not found, then a FileNotFoundError is raised.
- The pathlib.rmdir() function of the pathlib module is also used to delete an empty directory.
- The shutil module is used to perform high-level operations on files and directories. With the help of the shutil.rmtree().