What is the Difference between Append and Extend in Python?
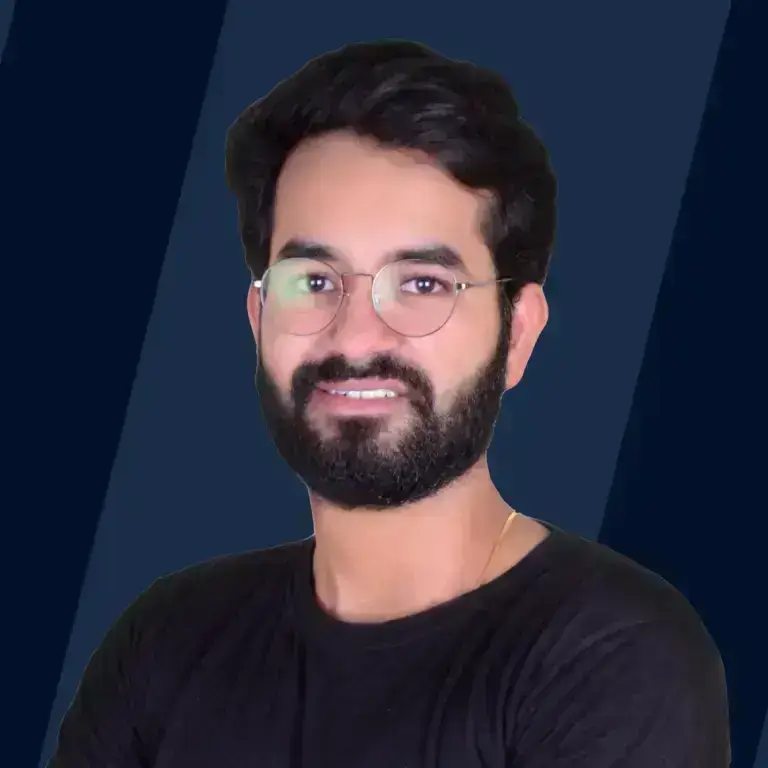
Overview
Append and Extend are two built-in methods that are available in the list data structure of Python. Before starting with append and extend, let's cover some points about list. The list is one of the data structures which is most commonly used in Python along with characteristics. Since a list is mutable so adding, removing, deleting, and updating the elements of lists are easy and very common.
There are many methods that are available in list like:
Append: Append is like adding an element to the existing list.
Extend: Extend is like iterating over an argument and adding each element to the list.
Append in Python
The Append method of the list is used to add an element at the end of the list. It will add an element to an existing list, and the length of the list will be increased by one.
Syntax:
Parameter:
element: It can be any object like a number, a string, or another list that will be considered as a single element and added at the end of the existing list.
Return: It will insert an element at the end of the list which is passed as an argument in the existing list.
Code:
Output:
extend() in Python
The Extend method accepts an iterable whose elements will be appended to the list. For example, if the input to extend() is a list, it will concatenate the first list with the other list by iterating over the elements of the input list. This means that the resulting list’s length will be increased with the size of the iterable passed to extend().
Syntax:
Parameter:
Iterable: It can be a list or any iterable which can be appended to the list.
Return: It will insert an iterable object at the end of the list which is passed as an argument in the existing list.
Code:
Output:
Note: A string object is an iterable object, so when we pass the string in the extend() method, it will append each character as if iterating over a string.
Difference Between Append and Extend in Python
Append | Extend |
---|---|
The element passed in the append() argument is added at the end of the list. | Iterable is passed as an argument and added at the end of the list. |
It will add an element to the list without any changes. | Iterable Object will append each of the elements at the end of the list. |
The length of the list will increase by 1. | The length of the list using extend() will increase by the length of the iterable object. |
The time complexity of the append() method is O(1). | The time complexity of the extend() method is O(k), where k is the length of the iterable. |
Learn more
Conclusion
- append() is used to add an element at the end of the list.
- extend() is used to add an iterable object at the end of the list within the list.
- The time complexity of the append() method is O(1), and the extend() method is O(k), where k is the length of an iterable object.