Difference Between List and Tuple in Python
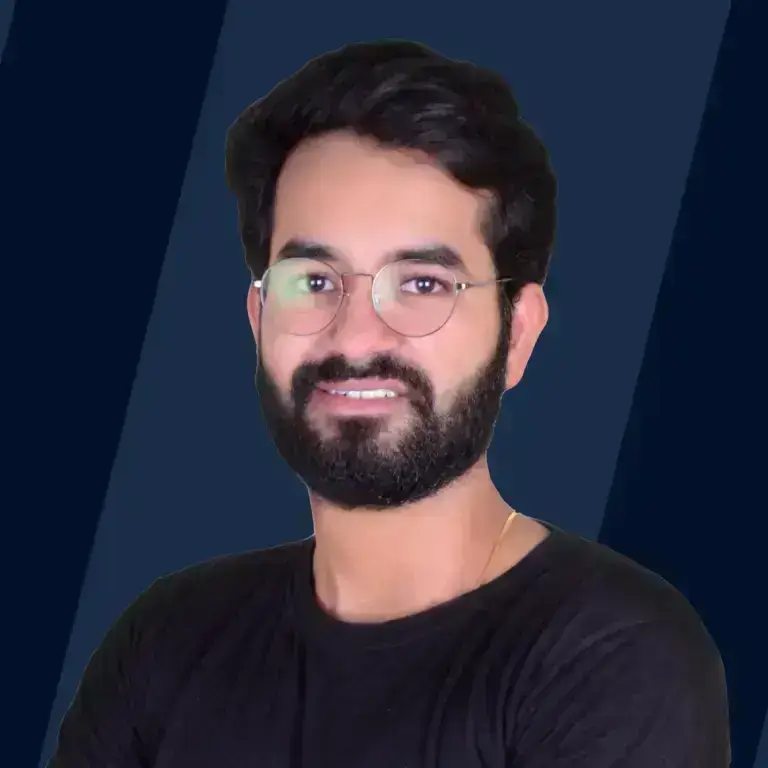
Overview
In Python, List is a type of container in data structures, which is used to store multiple data types at the same time. Lists are a useful tool for preserving a sequence of data and further iterating over it. While, a Tuple is an immutable collection of Python objects separated by commas i.e a tuple can’t be changed or modified after its creation.
What is List in Python?
A list is an essential data structure in Python for organising and storing collections of objects. The Python programming language depends on lists and they are highly flexible. They are made by enclosing a list of objects inside of square brackets[]. You can access objects in lists using their indices because they are sorted, which means they keep a particular sequence. Python lists are highly adaptable for managing dynamic data since they may be modified, added to, or removed from. Lists provide the useful ability to contain items of several data types, making it simple to build composite data structures.
A variety of operations are supported by lists, such as indexing to retrieve specific components, slicing to extract sublists, and a number of techniques for adding, deleting, and altering members. Lists are an effective tool for processing data, iterating over collections, and maintaining structured data because of their adaptability. Python lists are essential for quickly organising and manipulating data in Python programmes, whether you're working with straightforward lists of numbers or more intricate data structures.
What is Tuple in Python?
A tuple is a basic data structure in Python that roughly resembles a list but has some unique features of its own. Tuples are arranged groups of components denoted by brackets (). The fact that tuples are immutable—that is, that once you form a tuple, you cannot modify its members or size—is one of the most important distinctions between tuples and lists. Tuples are the best choice in situations when data stability and integrity are crucial. Tuples are frequently used to group similar values together, but they may also contain pieces of different data kinds, making them adaptable for the construction of heterogeneous data structures.
Tuple elements are very helpful in instances when you want to make sure the data stays consistent during the program's execution. You can access tuple elements by indexing and slicing, just like you would with lists. Tuples can be used to represent coordinates, date and time values, configuration options, and function return values in a number of programming scenarios. They are a useful tool for managing and protecting data in Python programmes since their immutability offers a measure of safety by avoiding unintentional changes.
Key Difference Between List and Tuple in Python
The main difference between List and Tuple in Python is Mutability. Lists are changeable, which means you may alter their elements or size after creation, enabling the processing of dynamic data. Tuples, on the other hand, are immutable, which means that once a tuple has been defined, its components or size cannot be changed. Due to their immutability, tuples are appropriate in settings where data stability and integrity are essential, but lists are more adaptable in situations needing frequent updates or revisions.
Both lists and tuples can store any data such as integer, float, string, and dictionary. Lists and Tuples are similar in most factors but in this article we will describe the main difference between them. Consider the nature of your data and the operations you need to perform when deciding between a list vs tuple in Python.
Difference Between List and Tuple in Python
S. No | List | Tuple |
---|---|---|
1 | Lists are mutable. | Tuples are immutable. |
2 | Iteration in lists is time consuming. | Iteration in tuples is faster |
3 | Lists are better for insertion and deletion operations | Tuples are appropriate for accessing the elements |
4 | Lists consume more memory | Tuples consume lesser memory |
5 | Lists have several built-in methods | Tuples have comparatively lesser built-in methods. |
6 | Lists are more prone to unexpected errors | Tuples operations are safe and chances of error are very less |
7 | Creating a list is slower because two memory blocks need to be accessed. | Creating a tuple is faster than creating a list. |
8 | Lists are initialized using square brackets []. | Tuples are initialized using parentheses (). |
9 | List objects cannot be used as keys for dictionaries because keys should be hashable and immutable. | Tuple objects can be used as keys for dictionaries because keys should be hashable and mutable. |
10. | List have order. | Tuple have structure. |
Examples
Mutability
The major difference between list and tuple whereas lists are mutable, and tuples are immutable. The lists are mutable means that the Python object(list) can be modified after creation, whereas tuples can't be modified after creation.
Let us understand this with the help of following example, by creating a list named fruits:
Output:
Now we are changing the first fruit by accessing the 0th index in the fruits list.
Output:
Let's now perform the same operation on a tuple:
Output:
Now we are changing the first fruit by accessing the 0th index in the fruits tuple.
Output:
We encounter an error while changing the first element (at 0th index) of the tuple because of immutability. As tuples does not support item assignment.
Debugging
The tuples are easy to debug in a large scale project because of its immutability. If we have a small scale project having less data, then lists are a good choice for effective programming. Let us understand this with the help of following example,
Output:
In the above program, we did b = a, here this line of code does not mean we are copying the list object from a to a. This b now refers to the address of the list a which implies if we make any change in the list a then that will reflect the same as in list b, and thus debugging becomes easy. But it is hard in case where Python objects may have multiple references, it will be very complicated to track those changes in lists but immutable object tuple can't be changed once created thus making tuples easy to debug.
Functions Support
The tuples support less operation than the list. The inbuilt dir(object) is used to get all the supported functions for the list and tuple.
List Functions
Output:
Tuple Functions
Output:
Efficiency
The tuples are more memory efficient than the list because tuple has less built-in operations. Lists are suitable for the fewer elements whereas tuples are a bit faster than the list when dealing with huge amount of data.
Output:
In the above program, despite the tuple A is having more number of characters as compared to the list B, list is occupying a larger size in computer's memory
Conclusion
- The literal syntax of tuples is shown by parentheses () whereas the literal syntax of lists is shown by square brackets [] .
- Lists and Tuples are data structures in python and are used to store one or more Python objects or data-types sequentially.
- Tuples can used as keys in a dictionary in python because these are hashable and immutable whereas lists can't be used as keys in dictionary.
- Tuples are used for unchangeable data that means data will be write- protected in tuples. Tuples sends an indication to the Python interpreter that the data should not change in the future.