How to Do an Email Validation in JavaScript?
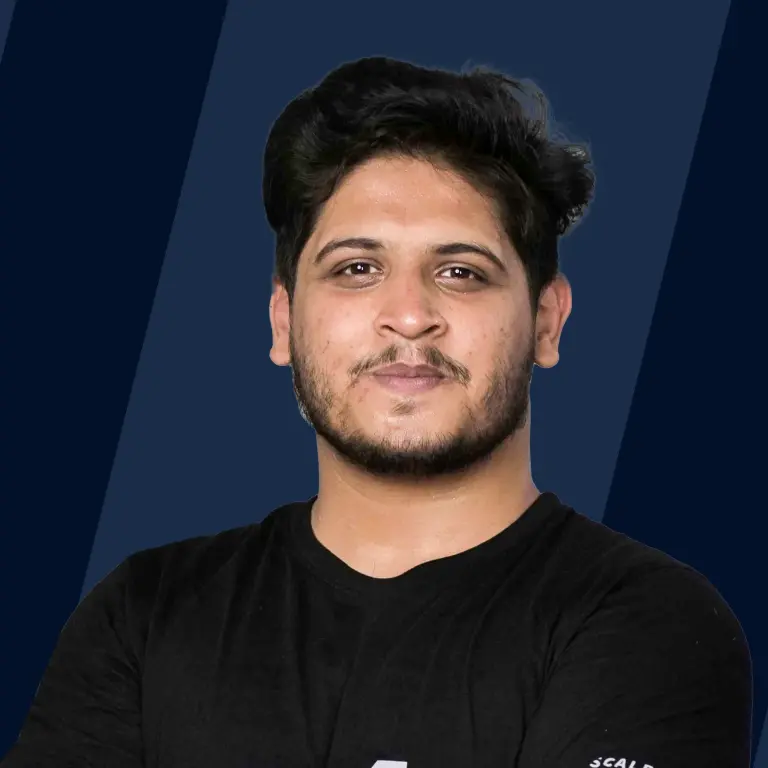
Overview
JavaScript is a modern and widely accepted language that is loved by many developers. Why does everyone love JavaScript? Because it is one of the most popular languages that is used to create Full-Stack applications that have both the Frontend and the Backend and is also used to create beautifully designed web pages.
Apart from creating websites and making them look cool, JavaScript also helps in many ways. It improves the user experience on the website by providing some necessary features like Form Validation in Javascript.
Form Validation in Javascript means JavaScript applies some checks to the form data at the time of entering data so that the wrong type of data is not sent to the server and errors occur.
What is validation?
Validation is a process that makes sure that the data that the user has entered into a form is of the correct type. Simply put, It is the process of checking the values inputted by the user. This is done on the client-side, and its purpose is to save time for the server.
For example, Let's suppose there is a form on a page where you have to enter your phone number. The phone number has some rules that it should be of 10 digits (in India), and it should only have numerals and no other characters. So, we can use JavaScript to check these things before sending data to the server. This will help the user to catch errors and also save the server time. Also, the user will get an immediate response about any errors.
How does Email Validation work in JavaScript
To understand how email validation work first we have to understand what an Email is: An email is a string of ASCII characters (alphabets, numerals, special characters, etc.) which is separated into two parts by the @ (at) symbol.
- First part - personal information
- Second part - domain name at which the email is registered.
Personal information can contain:
- Uppercase and lowercase letters (A-Z and a-z)
- Numeric characters (0-9)
- Special characters - ! # $ % & ' * + - / = ? ^ _ { | } ~`
- Period, dot, or full stop (.) with the condition that it cannot be the first or last letter of the email and cannot repeat one after another.
Domain name contains:
- Letters
- Digits
- Hyphens
- Dots
Now that we know what an email consists of, we can now learn the ways we use it for validation.
- Using Regular Expressions - This is the preferred way as it provides more flexibility and reduces the chances of error.
- Without Regular Expressions - This is a simpler method but less flexible
Let's learn about both ways.
A regular expression is a sequence of characters that specifies a search pattern in the text. Let's look at a code example to see how RegEx can be used for validation.
First, we have to make an HTML form, and we will link a JavaScript file to it.
Now we have to write the JavaScript code using Regular Expressions that will validate the emails.
Here are some of the simple steps that you have to follow:
First, we make a variable that will store regular expression literal, which consists of a pattern enclosed between slashes: const myRegEx = /ab+c/; After this, we use the match() to match the values of the inputted text with the pattern that we have created. There are also other functions that can be used with regular expressions like exec(), test(), matchAll(), search(), replace(), split().
Email Validation Methods in JavaScript
Email validation is an important feature in web development as it ensures that the user inputs a valid email address. This is crucial for various reasons such as preventing spam and ensuring that communication channels are secure. In JavaScript, there are different methods that developers can use to validate email addresses. This article will explore two of these methods: the regular expression method and the index values method.
- Regular Expression Method: The regular expression method involves using a pattern to match the email address. This pattern is a string of characters that identifies the email format. Developers can use the RegExp object in JavaScript to implement this method. The following code snippet shows how this can be done:
In the above code, the regular expression pattern is stored in the regex variable. The pattern matches the following format:
- The email address should start with one or more word characters.
- It can be followed by zero or more occurrences of the dot or dash characters, which should then be followed by one or more word characters.
- The domain part should start with one or more word characters.
- It can be followed by zero or more occurrences of the dot or dash characters, which should then be followed by either two or three word characters. The test() method is used to check whether the email address matches the pattern. It returns true if it does, and false otherwise.
- Index Values Method: The index values method involves checking whether the email address contains certain characters in specific positions. Developers can use the indexOf() method in JavaScript to implement this method. The following code snippet shows how this can be done:
In the above code, the indexOf() method is used to find the position of the @ and . characters in the email address. The lastIndexOf() method is used to find the position of the last . character in the email address. The method returns true if the email address satisfies the following conditions:
- The @ character should be present in the email address, and its position should be greater than zero.
- The . character should be present in the email address, and its position should be greater than the @ character's position plus one.
- The last . character's position should be less than the length of the email address minus one.
Examples
Simple Email Validation Check
Output
Email Validation with characters, digits, special characters, @, and dot
Output
Email Validation with @ and dot.
This we can do without the use of Regular Expressions
Output
Email Validation with Alphabets, Numbers, and Special Characters
Output
Regular Expressions may look very scary and like someone has written complete garbage at first look, but if you understand them, then they can help you in many ways.
Want to become a JavaScript ninja? Scaler Topics certification course provides the training and recognition you need to succeed.
FAQs
Q: What is form validation in JavaScript?
A: Form validation in JavaScript means applying some checks to the form data at the time of entering data so that the wrong type of data is not sent to the server and errors occur. This is done on the client-side, and its purpose is to save time for the server.
Q: What is email validation?
A: Email validation is the process of verifying whether an email address is valid and can receive emails. This is an important feature in web development as it ensures that the user inputs a valid email address.
Q: What are regular expressions?
A: Regular expressions are a sequence of characters that specify a search pattern in text. They are often used for validating data input, including email validation.
Q: What is the index values method for email validation in JavaScript?
A: The index values method involves using the indexOf() and lastIndexOf() methods to check the presence and position of specific characters in the email address, such as the @ symbol and period. If these characters are present in the correct positions, the email is considered valid.
Conclusion
- Form Validation in Javascript on a website improves user experience and makes the website more attractive.
- Validation is the process of checking the data inputted by the user against some rules.
- Form Validation in Javascript is preferred on the client-side as it saves the server time.
- For validating an Email with JavaScript, we use Regular Expressions.
- Regular Expression is a sequence of characters that specifies a search pattern in the text.