What Does Endl Mean in C++?
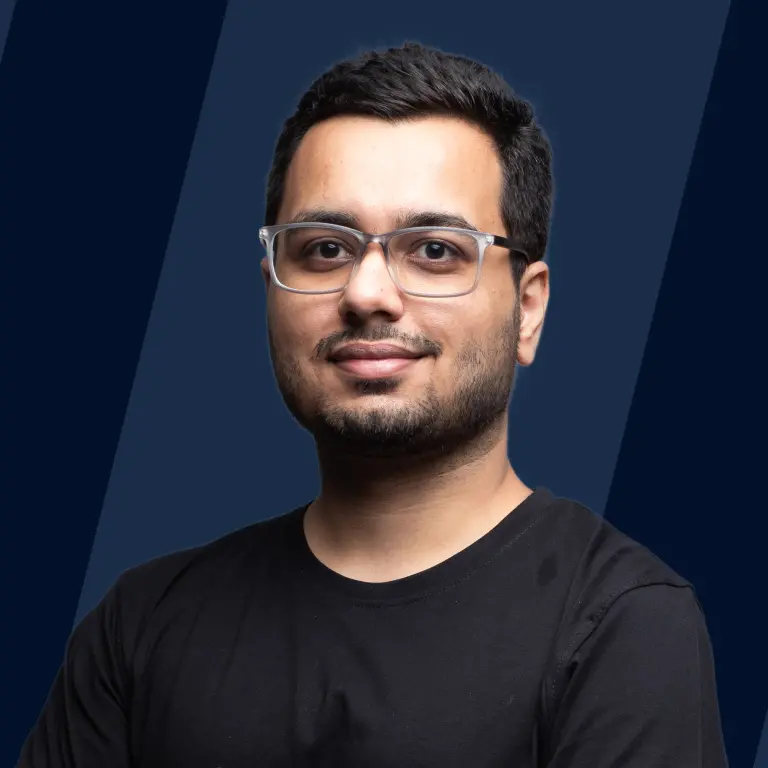
The endl in C++ is one of the manipulator functions used to modify the input and output stream in C++. The manipulators are used to format the data that is displayed to the user and are used along with the insertion(<<) and extraction(>>) operators. The functions in manipulators vary as functions with parameters and without parameters, whereas endl is a manipulator function without parameters.
Syntax of endl
The syntax of the endl function is as follows,
- The basic_ostream implements output operations on character output streams. It is used to insert characters into the output stream and format them.
- The std represents the standard namespace of C++.
- The CharT represents the type of character that is stored.
- The Traits are all objects that allow us to get information about a data type during the completion of the program. In our case, the Traits class provides information about characters used in the stream.
- The os refers to the output stream and is used to interact with the output stream.
- The ampersand symbol(&) represents that the parameter passed and the value returned will be an reference to the original variable.
Note on CharT
In C++, a character array is usually represented at a basic level as std::basic_string<char>, which has the CharT parameter as char, which helps to represent the character array as a string using the std::string. Therefore, CharT is also used to map different data representations. Similarly, the sdt::basic_string<wchar_t> is the basic level representation of a wide character array and has the CharT as wchar_t(wide character) and represents the std::wstring type. A wide character is larger than a normal character and is used to hold characters in languages like Chinese, Korean, etc...
Note on template
A template is used to create a generic class that can modify the code according to the data type passed during the code compilation. Most of the internal programs in C++. have been written using templates. Learn more about templates in C++
Is It endl or end1 in C++?
The manipulator used to create a new line is called endl in C++, and there is nothing such as end1 in C++. The end1 is a typo that beginners often make during programming in C++.
Where is endl Defined?
The manipulator function, endl in C++, is defined in the std::basic_ostream class, defined in the ostream header. So the header #include<ostream> must be included in the code to use the endl function. In most of the programs in C++, we can find the iostream header and not the ostream header, and the reason for this is that the iostream header includes both the istream(input stream) which is used for input formatting and reading operations and the ostream(output stream) headers.
The following images show the basic architecture of the ostream header,
- The class in std::ios_base ensures that the input and output streams are properly created during the program and destroyed after the program.
- Six global basic_ostream objects are provided by the standard library to work with the output stream.
What is the Type of std::endl?
- The std::endl is a function that takes a reference to the output stream as an input from the std::cout function and adds a new line followed by the flushing of the output stream.
- The std::endl returns the modified output stream of type std::basic_ostream.
- Therefore, there is no type for std::endl as it is a function, and both the parameter and return type of the function is std::basic_ostream.
Endl vs “\n”
The \n is called the new line character and is used to create a new line in C++. The backslash(\) is called the escape sequence and indicates that the n is a special character.
Is “\n” Faster than endl?
The new line character (\n) is faster than the endl function. The reason for this is as follows,
- The endl manipulator creates a new line and flushes the output buffer every time the endl manipulator is used. The \n character does not flush the buffer.
Therefore, when an endl manipulator is used, two system calls are made, and in the case of \n, only one system call is being made.
Code: The endl manipulator
The above code is equivalent to the following code,
The speed difference between \n and endl becomes insignificant in line-buffered standard outputs in which the buffer is flushed for every line. In such cases, the \n will also cause a flush of the buffer.
The flushing of the stream by \n can be prevented using the C++ function, std::cout.sync_with_stdio(false), which unties or prevents the synchronization of cout and cin streams. In such cases, the \n will not cause flushing of the stream, and only manual flushing can flush the buffer. This is often used in competitive programming to lessen the time complexity of programs.
Flushing of Buffer A flush of buffer implies cleaning the buffer, a temporary storage area for data used in the code, and sending the data to permanent memory. For example, flushing a buffer is similar to sending data from the program's local memory to the computer's terminal on which the program is executed and also cleaning the local memory.
In C++, the cin and cout buffers are usually synced, which means that when data is requested from cin, the cout buffer will be cleared and vice versa. Consider the following code snippet,
When the buffer is untied, the program may ask the user for a name before displaying the text Enter name: to the user.
What Is the Advantage of endl Over “\n”?
- The endl manipulator doesn't need any memory, whereas the \n character occupies 1 bit of memory.
- endl manipulator performs the functions of creating a new line and carriage return, whereas \n only creates a new line. Carriage return is the process used to move the cursor to the beginning of the line. The carriage can also be done using the \r character.
- The endl can be used when a flush of buffer and a new line is required.
What is the Difference between Endl and N in C++ programs?
- The endl is a manipulator defined in the C++ library, whereas \n is a special character.
- The C++ endl function doesn't work in libraries such as boost::lambda and QtCore.
- The endl manipulator is only supported by C++, whereas C and C++ programming languages support the new line character.
Learn More
Learn more about the major differences between endl and the new line character in this article, endl vs. \n.
Is Endl a keyword in C++?
Endl is not a keyword in C++. The endl is a function declared in the ostream library of C++.
How Does std::endl Work?
The std::endl manipulator calls the functions from the output stream passed from the std::cout as a parameter to the std::endl function.
The functions used from the output stream when the std::endl function is used provided in the following code snippet,
The os in the above code references the output stream. After the changes in the stream are made, the output stream is returned as a reference.
The std::basic_ostream(output stream) widen() function is used to obtain the character relative to the ostream from characters passed to the function. In our case,\n is passed to make sense of the new line character and return a new line.
Disadvantages of Using std::endl
- The std::endl function flushes the buffer when used, which increases the time of execution of the program
- The C++ endl function doesn't work in libraries such as boost::lambda and QtCore.
- Due to the time complexity, the use of endl in some programs may result in less performance.
Conclusion
- The C++ endl manipulator creates a new line followed by flushing the stream.
- The std::endl manipulator is declared in the std::basic_ostream class in the ostream library of C++.
- The endl function is slower than \n in normal cases but can become significantly faster when using the std::sync_with_stdio(false); option.
- Certain performance degradation is found with endl in certain cases.
- The endl function works with functions from the output stream.