What is Event Listener in JavaScript?
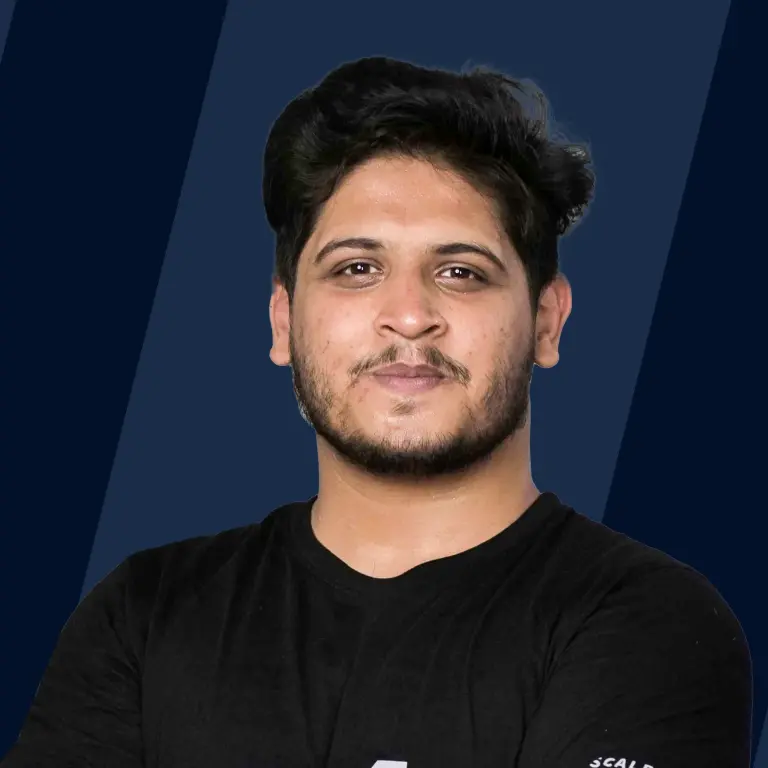
Event Listener in JavaScript is a procedure or method executed when any event occurs like a click event. An event is something that occurs in HTML elements.
For example: When a button is clicked, when a key is pressed, etc.
Event Listeners listen to those occurring events and execute the function performing any task.
Understanding Event Listeners in JavaScript
An event listener adds a responsive functionality to an element, allowing that element to wait and "listen" for the event to occur. Event listeners are similar to event handlers, but in event listeners, you can add multiple events on a single element.
Syntax :
We can add an Event Listener in JavaScript using the inbuilt addEventListener() method, the syntax is pretty simple :
Parameters :
The addEventListener() method takes the following parameters :
- event :
it is a valid JavaScript-defined event to be listened to. - listener :
it is a listener function containing the lines of code to be executed after listening to that particular event.
Example :
In this example, we are simply applying the eventListener for clicking the event on the button using the addEventListener() method.
Output :
- Before Button Click :
- After Button Click :
Adding Event Listener in JavaScript for Different Event Types
On the same element, we can add multiple event listeners example for keyup, click event. So let's take an example in which we are adding keyup, keypress, and change event on an input field.
Example :
Output :
We can see that the eventListener method is listening to all the 3 events going on.
Adding Event Listeners to Window Object
We can also add eventListeners to the "window object". So this will help us to track changes in the window object. So let's take an example in which we are listening to window size change.
Example :
Output :
We can see that the eventListener method is listening to the window resize event.
Removing Event Listeners
Removing event listeners is important when we don't wanna use event listeners anymore, so the removeEventListener() method can be used.
Example :
In this example, we are adding an event listener and then removing it so that input change will not get responded to as the event is removed from the listener.
Output :
We can see here that nothing has happened as the event is removed.
The Value of "this" within the Handler
We can get the information about the element from "this", So by using "this" in the function we can get information on an element like "id","class", and etc. value of this is the same as the currentTarget property of the current event argument.
Example :
In this example, we are getting the id of a current element using this.
Output :
We got the id of the input element on keyup event.
Getting Data Into and Out of an Event Listener
The Event Object, which is automatically supplied to the listener, is the only argument for event listeners, and the return value is overlooked. So, how can we get information into and out of them? There are several viable options for doing so :
Getting Data Into an Event Listener Using "this"
You could use Function.prototype.bind() to pass a value to an event listener via this reference variable, as explained before. In this example, we are giving a string variable containing the value "My string value".
Example :
Output :
we are using the bind function to bind the string value to the listener.
Getting Data Into an Event Listener Using the Outer Scope Property
When an outer scope declares a variable (with const, let), that variable is accessible to all inner functions declared in that scope. As a result, making data accessible to the scope wherein the event listener is defined. It is one of the easiest ways to access data from across an event listener. In this example, we are using the string variable in our function.
Example :
In this example, we are accessing the string value inside the event listener function.
Output :
On the button click the value of the string value is accessed inside the event listener.
Getting Data Into and Out of an Event Listener Using Objects
When an outer scope declares an object (with const, let), that variable is accessible to all inner functions declared in that scope. As a result, making the object accessible to the scope wherein the event listener is defined. It is one of the easiest ways to access its data from across an event listener.
Example :
In this example, we are accessing the property value of the object inside the event listener function.
Output :
On button click the property value is accessed from inside the event listener.
Browser Compatibility
Compatible Browsers are :
- Google Chrome
- Internet Explorer
- Firefox
- Opera
- Safari
- Edge
Conclusion
- Event Listener in JavaScript is a procedure or method executed when any event occurs.
- An event is a thing that is happening to HTML elements, for example: when a button is clicked, when a key is pressed, etc.
- Event Listener in JavaScript are similar to event handlers, with the exception that you can attach as many as you want to a single event on a single element.
- Syntax :
- addEventListener() method takes the following parameters :
- event
- listener_function
- We can use event listeners for different purposes.