Event Handling in JavaScript
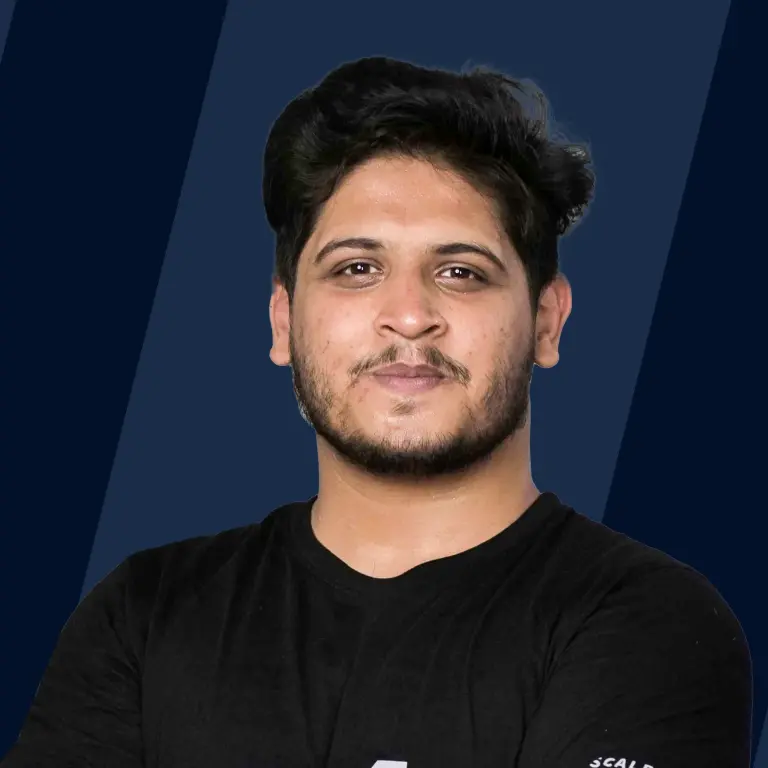
Overview
Event Handling in JavaScript is a software routine that processes actions that happen when a user interacts with the page, such as mouse movements and keystrokes. Event handlers in javascript are when an event is received by an event handler from an event producer and the processes that follow. Events are handled similarly in a browser. When the browser notices a change, it notifies an event handler (a function) that is listening to a specific event. The actions are then carried out as defined by these functions.
What are Event Handlers in JavaScript?
When an event, consider pressing a keyboard key or clicking an element, occurs on a DOM or an HTML element, we can call the specific functions based on these events. Now, how does the HTML element know when to call the mentioned JavaScript code or JavaScript function? This is taken care of by the event handlers. The properties of DOM or HTML elements are called event handlers to control how an element should respond to a given event. The concept and operation of event handlers are summarised in the figure below:
As shown in the above figure, when a user clicks a specific mouse button or types a specific keyword into the browser, that action activates the corresponding event handler for that HTML element. The browser then shows the end users the effects of the actions that were carried out on the webpage by the JavaScript code that was executed by the event handler.
Event handlers can be assigned directly using the equal (=) operator because they are attributes of HTML/DOM elements as well. The syntax is as follows:
Syntax:
Registering Event Handlers in Javascript
For registering handlers, there are two recommended methods. By assigning the event handler code to the target element's corresponding on event property or by adding the handler as a listener for the element using the addEventListener() method, the event handler code can be made to execute when an event is triggered. The handler will receive an object that complies with the Event interface in either scenario (or a derived interface). The main difference is that using the event listener methods, additional event handlers can be added (or removed).
Different Phases of Event Handling in JavaScript
The lifecycle of a JavaScript event contains three different phases of events:
- Capturing Phase: In the capture phase, generally known as the trickling phase, the event "trickles down" to the element that caused the event.
- Target Phase: It starts with the element and handler at the top level and works its way down to the element. When the event arrives at the target, the capture phase is over.
- Bubbling Phase: The event is "bubbled" up to the DOM tree during the bubble phase. The innermost handler initially captures and handles it (the one that is closest to the element on which the event occurred). After that, it moves up (or bubbles up) to the DOM tree's higher levels, moves up to its parents, and eventually returns to its root.
They follow the same order as listed above. As shown in the diagram below:
In event handling, when an event reaches the element, it enters the capturing phase. Events enter the target phase when they reach the element, and they up from the element during the bubbling phase.
HTML Event Handler Attributes
The names of event handlers typically start with on; for example, onclick is the name of the event handler for the click event. Use an HTML attribute with the name of the event handler to assign an event handler to an event connected to an HTML element. For example, you may use the following to run some code when a button is clicked:
In this example, the alert box appears when the button is clicked.
The HTML characters like the ampersand (&), double quotes ("), less than ("), and other special characters must be escaped when using JavaScript code as the value of the onclick attribute. Otherwise, a syntax error will occur.
A function defined in a script can be called by an event handler defined in HTML. For example:
When a button is clicked in this example, the myAlert() function is called. A separate <script> element defines the function "myAlert()" which could be included in a separate JavaScript file.
When using event handlers as attributes of an HTML element, the following are some important aspects:
First, without explicitly defining the event, the event object can be accessed by the code in the event handler:
Second, the event's target element is represented by the this value inside the event handler:
Third, the element's properties can be accessed by the event handler, as shown below:
Disadvantages of Using HTML Event Handler Attributes in JavaScript
Due to the following reasons, assigning event handlers using HTML event handler attributes is not regarded as a good practice and should be avoided as much as possible in event handling:
- The event handler code is first mixed in with the HTML code, making it more difficult to extend and maintain.
- Timing issue, Users may start interacting with the element on the webpage, which will result in an error if the element fully loads before the JavaScript code.
- Consider the following myAlert() function, which is defined in a separate JavaScript file:
Besides this, the myAlert() function returns an unknown value when the page has fully loaded but JavaScript has not. Users will experience an error if they press the button at this time.
Event Objects
Event handler functions accept an argument, the event object, even though we haven't used it up to this point. Additional information about the event is also included in this object. For example, we can check the event object's button property to verify which mouse button was pressed.
Various Types of Event Handlers in JavaScript
JavaScript offers a variety of event handlers that are activated in response to specific actions taken on the HTML elements. A few of the event handlers in javascript are:
Event Handler | Description |
---|---|
onclick | When a click action occurs on an HTML element, this event handler runs a JavaScript script. For example, the onClick event handler may be called when a button is clicked, a link is clicked, a checkbox is checked, or an image map is selected. |
onmouseover | When the mouse is over a particular link or object, this event handler runs a JavaScript script. |
onload | When a window or image has fully loaded, this event handler executes some JavaScript code. |
onkeypress | When a user presses a key, this event handler executes JavaScript code. |
onmouseout | When the mouse leaves a specific link or object, this event handler runs a JavaScript script. |
onkeyup | When a key is released during a keyboard action, this event handler executes JavaScript code. |
onkeydown | When a key is pressed on the keyboard during an action, this event handler runs a JavaScript script. |
Propagation
For the majority of event types, the handlers registered on nodes with the children will get children-level events. If the button within a paragraph is clicked, the paragraph's event handlers can also see that click event. If both the button and the paragraph have a handler, the handler on the button, which is more specific, will execute first.
The event is said to propagate outward, from the node where it occurred to the parent node of that node and then to the document's root. After every handlers registered on the particular node has had their chance to respond to the event, the handlers that registered on the entire window are given the chance to do so.
A handler for an event can invoke the stopPropagation method on the event object at any time to prevent successive handlers from getting the event. This may be useful in event handling in javascript if, for example, we have a button inside another clickable element, and we do not want button clicks to activate the outer click behavior of an element.
Default Actions
For the majority of event types, the JavaScript event handlers are called prior to the default behavior. If the handler does not want this normal behavior to occur, typically because it has already handled the event, it can call the event object's preventDefault method.
This allows you to implement custom keyboard shortcuts and context menus. It may also be used to interfere obnoxiously with the expected behavior of users. For example, the following link cannot be followed:
JavaScript Key Events
The key events occur each time a user interacts with the keyboard. There are three main key event types in javascript that involves keyup, keydown, and keypress.
Event | Description |
---|---|
Onkeydown | This event is triggered when the user is pressing a key. |
Onkeypress | This event is triggered when the user presses the key. |
Onkeyup | This event is triggered when the user releases the key. |
Despite its name, "keydown" is not only triggered when a key is physically pushed. When a key is pushed and held, the event is triggered each time the key is repeated. Or sometimes, you must take extra care in this case. For instance, If we add a button to the Document Object Model when a key is pushed and delete it when the key is released. Now, if the key is held down longer you may accidentally add hundreds of buttons.
Pointer Events
Pointer events are the DOM (Document Object Model) events that are triggered for a pointing device. There are some common methods for pointing at objects on a screen such as mouse (including devices that act like mice, such as trackballs and touchpads), stylus/pen, and touchscreens. The pointer is a device that is hardware-independent and can target a specific set of screen coordinates.
Mouse Clicks
When any mouse button is pressed, a number of events are triggered. Similar to the "keydown" and "keyup", the "mouseup" and "mousedown" events are also fired when the mouse button is pushed and released. And, these occur on the DOM nodes directly under the mouse pointer at the time the event happens.
After the event, "mouseup", the "click" event is fired on the most specified node which contains both the button press and release. For example, if we hold down the mouse button on one paragraph, and move the pointer to another paragraph, then release the button, the event "click" will occur on the element containing both paragraphs.
Events and the Event Loop
Event handlers function similarly to other asynchronous notifications. They are scheduled to run when the event occurs but must wait for other scripts to complete before they can execute.
The event loop is the key to asynchronous programming in JavaScript. JS executes all operations on a single thread, but through the use of a few smart data structures, it gives the illusion of multithreading. Let's analyze what occurs behind the scenes (at the backend).
The call stack is responsible for keeping track of all operations in the execution queue. A function is popped from the stack whenever it completes.
The event queue is responsible for sending new functions for processing to the stack. It complies with the queue data structure in order to maintain the correct execution order of all operations.
The event loop facilitates this process by continuously checking whether the call stack is empty. If it is empty, the event queue is used to add new functions. If not, the current function call is then executed.
Timers
A timer is created in JavaScript to execute a task or function at a specified time. Mainly in event handling in javascript, the timer is used to delay program execution or to execute JavaScript code at regular intervals. The timer allows us to delay the execution of the code. Thus, the code does not finish executing simultaneously when an event triggers or a page loads.
Sometimes it is necessary to cancel a previously scheduled event. This is actually done by storing the value returned by setTimeout and then calling clearTimeout on the value.
Debouncing
Debouncing in JavaScript is a technique used to enhance the performance of web browsers. A web page may contain functionality that requires time-consuming computations. As JavaScript is a single-threaded programming language, frequent implementation of such a method could negatively impact browser performance.
Debouncing is a programming method for preventing time-consuming tasks from firing so frequently that they slow down the performance of a web page. In other words, it limits the frequency of function calls.
No matter how many times you click the debounce button, it gets executed once every 3 seconds!!
DOM Level 0 Event Handlers
Every element has properties for event handlers, like onclick. As shown in the example, we set the property to a function to assign an event handler in javascript.
In this example, the function here becomes the method of the element button. And, the this value is therefore similar to the element. therefore within the event handler, we can access the property of the element:
Output:
In the event handler, you can access the properties of the element and methods by using the this value. And set the event handler property's value to null to remove the event handler as shown below:
The DOM Level 0 event handlers are still widely used due to their simplicity and browser compatibility.
DOM Level 2 Event Handlers
DOM Level 2 Event Handlers provides two primary methods for registering and deregistering event listeners:
- addEventListener() – this method in javascript attaches an event handler to an element without overriding the existing event handlers.
- removeEventListener() – It is an inbuilt function that removes an event handler from an element for an attached event.
The addEventListener() Method
This method takes three arguments: an event handler function, an event name, and a Boolean value instructing the method to invoke the event handler during the bubble phase (false) or the capture phase (true). As shown below:
Multiple event handlers can be added to handle a single event, as shown below:
The removeEventListener() Method
The removeEventListener() function removes an event listener added with the addEventListener() function (). However, you must pass the same arguments passed to addEventListener(). For example:
Using an anonymous event listener will not work for the following:
Which is Better - an Inline Event or addEventListener?
Before considering which one is better let's understand the differences first.
- addEventListener allows you to register an unlimited number of event handlers.
- removeEventListener can be used to delete the event handlers.
- Inline events can be overwritten.
- The useCapture flag indicates whether an event should be processed during the bundle phase or the capture phase.
These are the major differences. To ensure that HTML tags are easily readable, it is advisable to use the javascript environment for event handling. Also, use addEventListeners if multiple functions or expressions are to be applied to an event; otherwise, use inline events.
Examples
Conclusion
- Event Handling in JavaScript is a software routine that processes actions that happen when a user interacts with the page, such as mouse movements and keystrokes.
- Events are handled similarly in a browser. When the browser notices a change, it notifies an event handler (a function) that is listening to a specific event. The actions are then carried out as defined by these functions.
- When an event, such as clicking an element or pressing a keyboard key, occurs on an HTML or DOM element, we can invoke specific functions based on these events.
- Event handlers can be assigned directly using the equal (=) operator because they are attributes of HTML/DOM elements as well.
- When an event reaches the element, it enters the capturing phase. Events enter the target phase when they reach the element, they up from the element during the bubbling phase.
- Event handler functions accept an argument, the event object, even though we haven't used it up to this point. Additional information about the event is also included in this object.
- The event loop is the key to asynchronous programming in JavaScript. JS executes all operations on a single thread, but through the use of a few smart data structures, it gives the illusion of multithreading.
- To ensure that HTML tags are easily readable, it is advisable to use the javascript environment for event handling. Also, use addEventListeners if multiple functions or expressions are to be applied to an event; otherwise, use inline events.