What are Getter and Setter in Java?
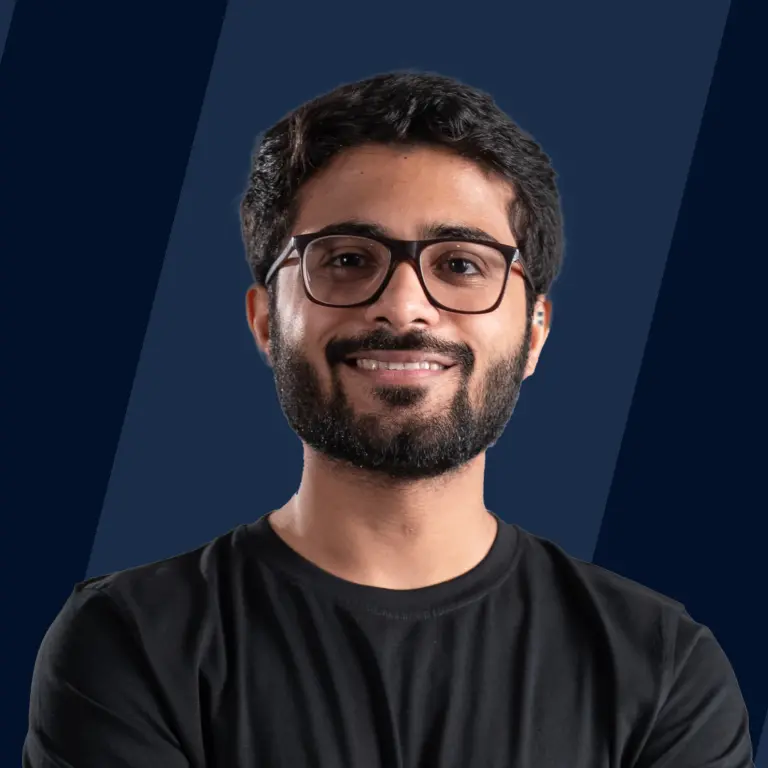
Getter and Setter are those methods in java that are used to retrieve the value of a data member and update or set the value of a data member respectively. Getters are also called accessors and Setters are also called mutators.
Getter and setter in java help in providing data hiding to prevent direct unauthorized access to the variables. By using getter and setter in java we are indirectly accessing the private variables. Once defined, getters and setters can be accessed inside the main() method.
Refer to the 'Example' section of the article for detailed implemented examples.
Example
Example: 1
Let us take an example to demonstrate the actual use of getter and setter in java.
Code:
Output:
Explanation:
- In the above program, inside the "Student" class there are two private variables that are 'name' and 'rollno'.
- Directly we cannot access those variables outside the class which is an implementation of encapsulation or data hiding.
- So we are using getters as getName() and getRollno() methods to retrieve the values and setters as setName() and setRollno() methods to update or set the values.
- Inside setters, we used "this pointer" to access the data members of the current object it is pointing to.
- Here without directly accessing the variables, we are easily changing their values by just calling the setter functions and printing it by calling the getter functions.
Example: 2
Let us take another example using the same program but this we will add some validation inside the setRollno() function. This program will make your concepts clear regarding getter and setter in java.
Code :
Output :
Explanation:
- It's the same program we've used in the previous example but here we have added a condition or validation inside the setRollno() method so that when someone sets the rollno it should be under some range.
- Here we used 50 as the maximum possible value of a student's rollno inside a classroom which means the rollno should not be more than 50.
- Since the rollno is set as 51 in the program you will receive a message saying "Roll number is invalid".
The Need for Getter and Setter Method
If we are using access modifiers like public, default, and protected then we are compromising the overall security of the program because no data hiding is implemented.
So we can lose control over the data which means it can be accessed and changed from anywhere in the program, and we will not be able to provide any validation or condition for accessing that data member.
Therefore to provide security for accessing the data member we declare it using a private modifier.
- When a variable is encapsulated using a private access modifier it can be accessed only through the getter and setter.
- Getter and setter in java are used to achieve encapsulation and data hiding.
- They help the programmer control the access of important variables and how they will be updated in a proper manner using conditions.
- The getter and setter method provides functionalities like validation, and error handling using which we can change the behavior of the data member.
Naming Convention for Getter and Setter
As per the convention, the getter function starts with "get" followed by the variable name where the first letter of the variable is in the upper case. The same goes with setters, it starts with "set" followed by the variable name where the first letter is in uppercase.
Syntax Used for Getters :
Code:
Syntax Used for Setters :
Code:
Bad Practices in Getter and Setter
There are some usual bad practices done by the programmers when they work with getter and setter in java.
These bad practices include:
Using Getter and Setter for Less Restricted Variables
Let us take an example to understand:
There is class 'Laptop' which contains public data member 'price'. Two methods are defined that is setPrice() and getPrice() which are used to set the laptop's price and then retrieve it or display it respectively.
Code:
Output:
Explanation:
- In the above code, it is useless to define getter(getPrice) and setter(setPrice) as the variable is already declared in public scope which can be accessed directly using the (.)dot operator.
We can write the above program with a better approach so that no getters and setters will be used to access the data members or variables of the class :
Code:
Output:
Using Reference of an Object Directly in a Setter
Let us take an example to understand:
Inside the 'Array' class we have a private array 'element'. We have written a setElement() setter function in which values of one array are copied into the array declared inside the class. So the 'element' array values are set which is the same as the 'Arr' array which is declared inside the main() method.
Code:
Output:
Explanation:
- Here when we display the values of the element array it will be the same as that of the Arr array. We're changing the value in the Arr array only but changes take place in both the arrays.
- This should not happen because the element array is declared as private and any entity outside the class cannot make changes to it. This is happening because of the reference.
- The setElement() method is taking reference of Arr[] as the argument, and that reference is copied to the element array which means element[] is storing the reference of Arr[] and therefore whenever we make changes in Arr[] that change also reflects in element[]. This is the concept of shallow copy.
This violates the purpose of the setter as changes can be made even from the main method.
We can use a better approach to write this program:
Code:
Output:
Explanation:
- In the above code, we will declare a completely new array whose size is the same as the size of a[], so that element[] no longer refers to a[].
- Now, when we update a value in Arr[] it won't get reflected in element[] which can be seen in the output of the program.
- Here we are doing deep copy of the values from a[] to element[]. This program takes care of the purpose of the setter which allows any change in the private array only through setters that maintain encapsulation.
Returning of Object References from the Getter
Inside the 'Array' class we have a private array that is already declared with its values. We have written a getElement() getter function which returns the reference of the array when called from the main method.
Code:
Output:
Explanation:
- As we have seen earlier that if the reference of an array is passed as an argument then it can be changed from outside the class also.
- Similarly, if the getter returns the reference of the array directly, anyone from the outside code can use this reference to make changes in the private entity.
We can use a better approach to deal with this practice:
Code:
Output:
Explanation:
- Inside the getter function, a new array is created, having the same size as the element array, whose reference is sent to the main method.
- Hence, when the value at the 0th index gets updated, that change creates an impact on the new array that is the temp[] array, not the private array that is an element[].
Thus, it does not expose the reference of the private array to the outside world which maintains encapsulation.
Conclusion
-
Data hiding is a concept implemented to hide some essential details from object members which can be achieved using access modifiers like private and protected.
-
Getter is a method in java used to retrieve the value of the data members or variables and Setter is a method in java used to update or set the value of the data members or variables.
-
The getter method starts with 'get' followed by the variable name and similarly setter method starts with 'set' followed by the variable name.
-
Common bad practices done by programmers when they deal with getter and setter in java are as follows:
- Usage of getter and setter method for less restricted variables.
- Usage of object reference directly in a setter.
- Returning an object reference in the getter.