Priority of a Thread in Java
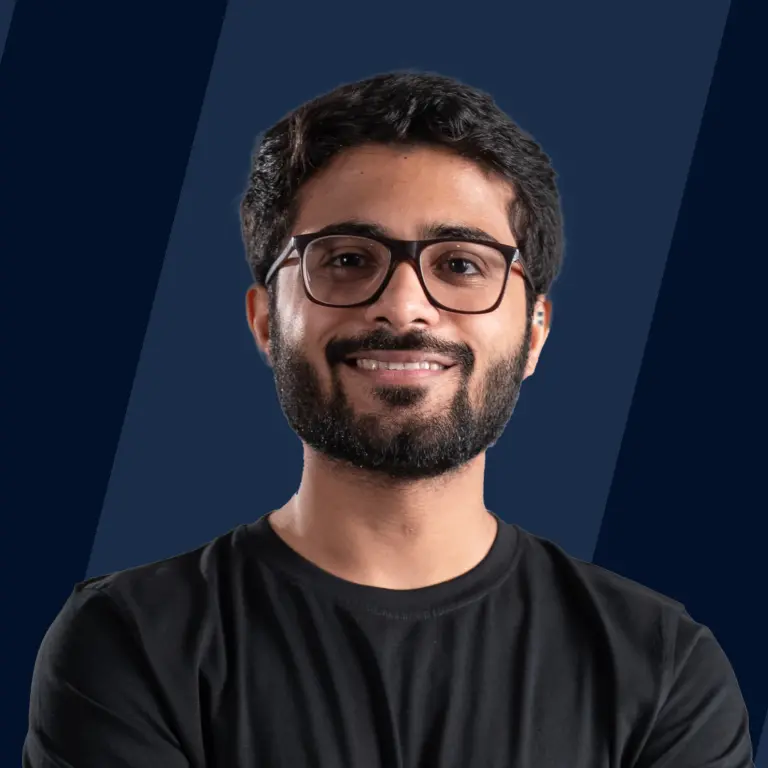
Overview
A thread is defined as the smallest sub-process unit of processing that is independent and utilizes a shared memory area. Now, to avoid any clash, as the memory area is common for all the threads, the priority in which the processing of these multiple threads will occur is called the Thread Priority in Java.
Setter & Getter Method of Thread Priority
We shall now discuss the setter and getter methods for the thread priority in Java.
public final int getPriority(): In the java.lang.Thread.getPriority() method, the output is always the priority of the specified given thread. It is also referred to as the Getter or Get method as we get the priority of the thread
public final void setPriority(int newPriority): In the java.lang.Thread.setPriority() method, the output is to update or assign the priority to newPriority of the specified thread. We also get an error such as IllegalArgumentException when the value of the newPriority comes out of the range. It can be 1 (for minimum) to 10 (for maximum). It is also referred to as the Setter or Set method, as we can update or assign the priority to a newPriority.
3 Constants Defined in Thread class
So far, we have learned that each thread created in JVM is assigned a priority. These thread priorities in java can be understood as the number of resources allocated to a particular thread where the priority range lies between 1 and 10.
Now we shall learn the three constant variables that are static and used for fetching the priority of a thread. These are described below:
-
public static int MIN_PRIORITY: This can be elaborated as the minimum priority that can be given to the thread, and the value this MIN_PRIORITY variable holds is 1.
-
public static int NORM_PRIORITY: This can be understood as the default priority, which is given to the thread if nothing is predefined. The default priority of the main thread is 5.
-
public static int MAX_PRIORITY: This can be described as the maximum priority given to the thread with a value of 10.
We must also note that the child thread will be assigned a priority that is equal to its parent thread priority. It is also possible to change the selection preference of any thread while evaluating the processing, even though it is the main thread or user-defined thread. It is advised that we always change the priority by using constants available in the Thread class as follows:
- Thread.MIN_PRIORITY;
- Thread.NORM_PRIORITY;
- Thread.MAX_PRIORITY;
Example of Priority of a Thread in Java
Code:
Output:
Explanation: From the above example of code for the scenario - Java Thread Priority, we have started by creating three threads with the help of JavaThreadPriorityExample class. We can observe here that as we stated the getPriority() method and did not define any value to it, so by default, it will give the output as 5 as can be observed from the output of the code. Then we utilized the setPriority() as 7, 4, and 9 respectively to each thread, and hence we get the same in the output by calling the getPriority() method.
Note: Threads with high priority will always get preference over the lower priority threads whenever it comes to the execution of the threads.
Example to Create Custom Thread with User-defined Name and Priority
Code:
Output:
Explanation: The above example discusses the creation of a custom thread with a user-defined name and Priority. Here we started with two threads (thr1, thr2). Then we let the first thread not touch and manipulated the name of the second thread as child2. Then, when we printed the name and priority of both the threads, we saw the expected output as above, where the first thread was named Thread-0 and child2 for the second.
As the priority was not explicitly mentioned, we got the output as 5 for both threads. Eventually, we started manipulating the first thread's name and priority and only with the second thread's priority.
Conclusion
-
A thread can be explained as the smallest sub-process unit of processing, that is, independent of each other and utilizes a shared memory area.
-
The priority in which the processing of the multiple threads occurs is called the Thread Priority in Java.
-
The Setter & Getter Method of Thread Priority in Java:
- public final int getPriority(): Returns the priority of the specified given thread.
- public final void setPriority(int newPriority): Returns the update or assigns the priority to newPriority of the specified thread.
-
The three constant variables that are static and used for fetching the priority of a thread are described as below:
- public static int MIN_PRIORITY: The minimum priority holds the value as 1.
- public static int NORM_PRIORITY: The default priority holds the value as 5.
- public static int MAX_PRIORITY: The maximum priority holds a value of 10.