How to Clear Screen in Python?
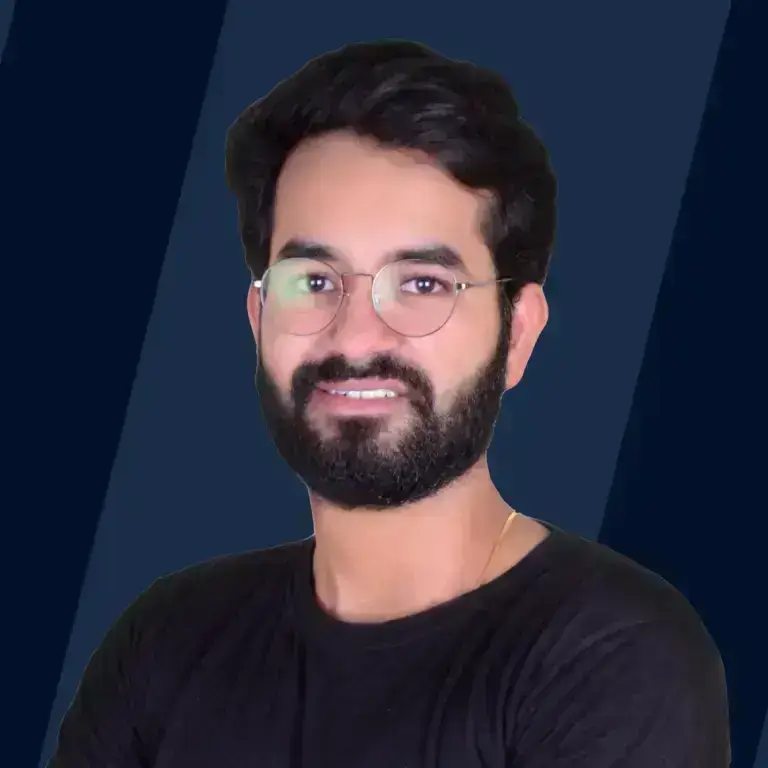
Overview
Python os module is imported to clear the console screen in any operating system. The system() method of the os module with the string cls or clear as a parameter is used to clear the screen in windows and macOS/Linux, respectively.
Introduction to Python Clear Screen
Suppose there is a case where you have given the information of 3 students, and you have to print their details in a way like first there will be information of student 1, then after some time information of student 2 will be displayed and then finally the information of student 3 will be displayed. So, in that case, we will have to clear the python console each time after displaying information about each student.
Image Explanation of the above Example
In an interactive shell/terminal, to clear the python console, we can use the ctrl+l command, but in most cases, we have to clear the screen while running the python script, so we have to do it programmatically.
We can clear screen programmatically, and it helps us to format the output in the way we want. We can also clear the output whenever we want many numbers of times.
How to clear the python console screen?
Clearing the console in python has different methods for different Operating Systems. These are stated below:
- In Windows: For clearing the console in the windows operating system, we will use the system() function from the os module with the 'cls' parameter.
Syntax of the system() function: system() function is in the os module, so the os module needs to be imported before using the system() function in Windows. After importing the os module, the string parameter 'cls' is passed inside the system function to clear the screen.
- In Linux and MacOS: For clearing the console in Linux and Mac operating systems, we will use the system() function from the os module with the 'clear' parameter.
Syntax: os module needs to be imported before using the system() function in Linux. After importing the os module string value parameter 'clear' is passed inside the system function to clear the screen.
- Ctrl+l: This method only works for Linux operating system. In an interactive shell/terminal, we can simply use ctrl+l to clear the screen.
Example of Python Clear Screen
Example 1: Clearing Screen in Windows Operating System
Let's look at an example of the clear screen in python to clarify our understanding.
We will use the above-stated system() function for clearing the python console.
First, we will print some Output; then we will wait for 4 seconds using the sleep() function to halt the program for 4 seconds, and after that, we will apply os.system('cls') to clear the screen.
Code:
Output:
The output window will print the given text first, then the program will sleep for 4 seconds, then the screen will be cleared, and program execution will be stopped.
Example 2: Clearing the screen, then printing some more information in Windows Operating System.
First we will print some Output, then we will wait for 1 second using the sleep() function, and after that, we will apply os.system('cls') to clear the screen.
After that, we will print some more information.
Code:
Output:
The output window will print the first information, then the program will sleep for 1 second, and the screen will be cleared, and then it will print the second information. Again the program will sleep for 1 second, and at last, the program will be stopped after printing the final batch of information.
Example 3: Clearing Screen in Linux Operating System
We will use the above-stated system() method for clearing the python console.
First, we will print some Output; then we will wait for 5 seconds using the sleep() function, and after that, we will apply os.system('clear') to clear the screen.
Code:
Output:
- After 5 seconds, the screen is cleared.
The output window will print the given text first, then the program will sleep for 5 seconds, then the screen will be cleared, and program execution will be stopped.
Example 4: What if we don't know what OS we are working on?
There can be a case where we must first determine what OS we are working on. So, we will first determine whether the os is Windows, Linux, or Mac.
For Windows, the os name is "nt" and for Linux or mac, the OS name is "posix".
So we will check the os name and then accordingly apply the function.
Code:
Output:
For this case, the os name is nt, so windows console will be cleared after 2 seconds of program sleep, and then it will be terminated.
Conclusion
Now that we have seen various examples of how to clear the screen in python let us note down a few points.
- Clearing Screen can be done programmatically, and also it helps us to format the output the way we want.
- For clearing the console in the windows operating system, we will use the system() method from the os module with the ‘cls’ parameter.
- For clearing the console in Linux operating system, we will use the system() method from the os module with the ‘clear’ parameter.
- For windows, the os name is nt, and for Linux, it is posix, so we can also first determine the os and then clear the screen.
Read More
1- What is clear() in Python?
2 - How to install python in linux?