Python sleep()
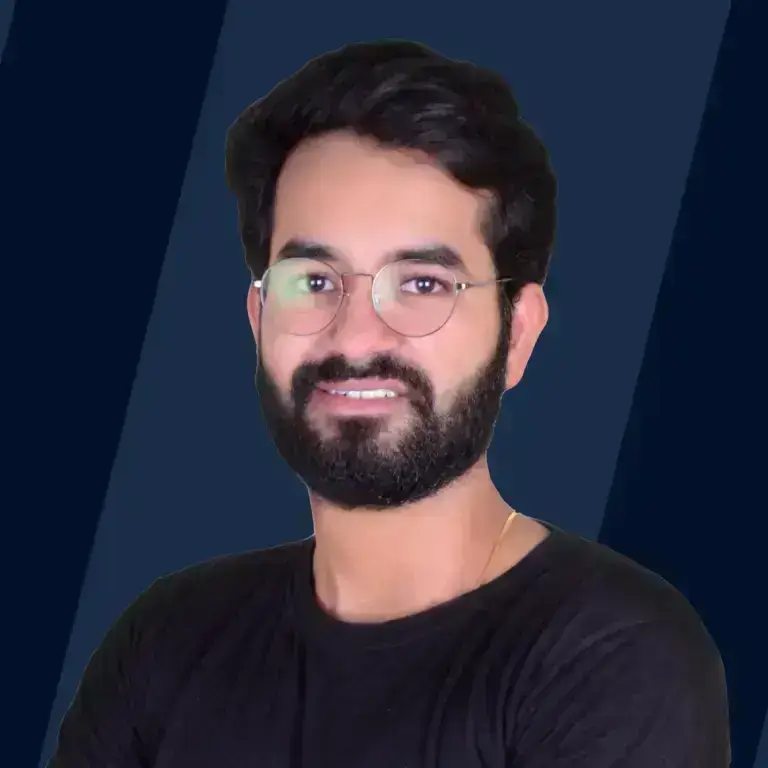
The time.sleep() in Python, part of the time module, allows for delaying program execution by pausing the current thread's operation for a specified number of seconds. This built-in function is essential for controlling execution flow and timing in Python applications.
Syntax of time.sleep() in Python
Parameters of time.sleep() in Python
The time.sleep() in Python has only one parameter, that is the number of seconds. It represents the delay in seconds, that we can specify for our code. The number of seconds can be either an integer or even a float.
Return Value of time.sleep() in Python
The sleep in python does not return anything in general.
Examples of Python sleep()
Let us see our first, very basic example of the time.sleep() function in Python.
Creating a Time Delay in seconds
In the below code, I have added a delay of 3 seconds before the execution of the two print statements.
Code:
Output:
Creating a Time Delay in minutes
Creating a time delay in minutes using Python's time.sleep() in python is a straightforward process, similar to creating a delay in seconds. The sleep in python takes the number of seconds as an argument, so to introduce a delay in minutes, you'll need to convert minutes into seconds.
Here’s a simple example that introduces a delay of 2 minutes between two print statements:
Code:
Output:
Applications of time.sleep()
The time.sleep() in python finds its utility in a variety of scenarios within Python programming. One significant application is in the management of background tasks which require execution at fixed intervals; by incorporating sleep in python, developers can efficiently schedule these tasks without overburdening system resources.
Time Delay in the Python loop
To introduce a time delay in a Python loop, allowing each loop iteration to pause for a specified duration, you can use the time.sleep() function. This is particularly useful for creating effects such as displaying text one character at a time.
Code:
Output: The output simulates the text "Loading..." appearing one character at a time, with each character displayed every 0.2 seconds, creating a typing or loading effect.
Time Delay in Python List
Creating the time delay in lists is very straightforward because we can put all our elements into the list and then iterate over the list to output their values. Each of the values in the list will be printed after the amount of delay we have provided with our sleep in python.
Let us look into the code for a better understanding :
Code:
Output:
Explanation: In the above code, we have imported the sleep() function from Python's time module. Here, all the messages will be prompted after a delay of 0.7 seconds. So, we can see that creating a delay in a list is a very straightforward task.
Creating Time Delay in Python Tuple
Creating the time delay in a tuple is very similar to that of creating the delay in a Python list. The only difference will be that, we would be using a tuple for this purpose. Apart from that, we would iterate over the tuple and specify the amount of delay that is expected into our time.sleep() function.
Code:
Output:
Explanation: As you saw in the above code, we did everything similar to what we did in the list. Every name will be printed with the specified delay inside the loop. Also, we have added one more delay before our loop. So, everything goes in order, as per the delay specified.
Creating Multiple Time Delays
Let us now learn how to create multiple time delays using the Python's time.sleep() function. We already saw an example above, where we created multiple time delays. But here we will be adding more delays and creating a suspence game.
Code:
Output:
Explanation: In the above code, we added multiple delays into our code. We created a suspense game where you had to guess something. But the main point is, that we can use time.sleep() any number of times in our code, and inside loops, with print statements, while calling functions, making API calls, etc. There is no limit to which we can create delays in our code using the time.sleep() function in Python.
Create a Digital Clock in Python
In this example, we will be creating a digital clock with the help of time.sleep() in Python. Let us look into our code, and then we will understand the operations we performed to create that digital clock.
Code:
Output:
Explanation: In the above code, we created our beautiful digital clock using time.sleep() in Python. So, let us discuss some important imports we have made in this code :
- time : As we already know, to use the sleep() function in Python, this is a mandatory import to be made.
- strftime : The strftime() method in Python returns a string representing the date and time using date, time or datetime object. We can import it from the time module in Python, similar to how we imported the sleep method.
Python thread sleep
The time.sleep() function in Python plays a crucial role in multithreading environments by pausing the execution of the current thread. This allows for simulating delays, controlling execution timing, or reducing CPU usage in threaded applications without affecting other threads' execution.
Code:
Output:
Explanation:
In this multithreaded program, we have two custom threads: Worker and Waiter. Upon starting, the main thread initiates both and immediately prints "Done," demonstrating non-blocking behavior typical of multithreading.
- The Worker thread prints numbers from 0 to 10, pausing for 1 second between each print. This simulates a task that periodically updates or checks for changes.
- The Waiter thread prints numbers from 100 to 102 but takes a longer pause of 5 seconds between prints, mimicking a task that requires less frequent updates or checks.
Conclusion
- The time.sleep() in Python halts the current thread, enabling precise control over program execution timing.
- Essential for diverse applications, time.sleep() supports tasks ranging from background operations to user interactions, across data structures and multithreading.
- Demonstrated through varied examples, time.sleep() can introduce delays in loops, lists, and digital clock simulations, enhancing functionality and user experience.
- In multithreaded programming, time.sleep() proves vital for managing execution flow and resource utilization without impacting parallel threads.