HTML Handlebars
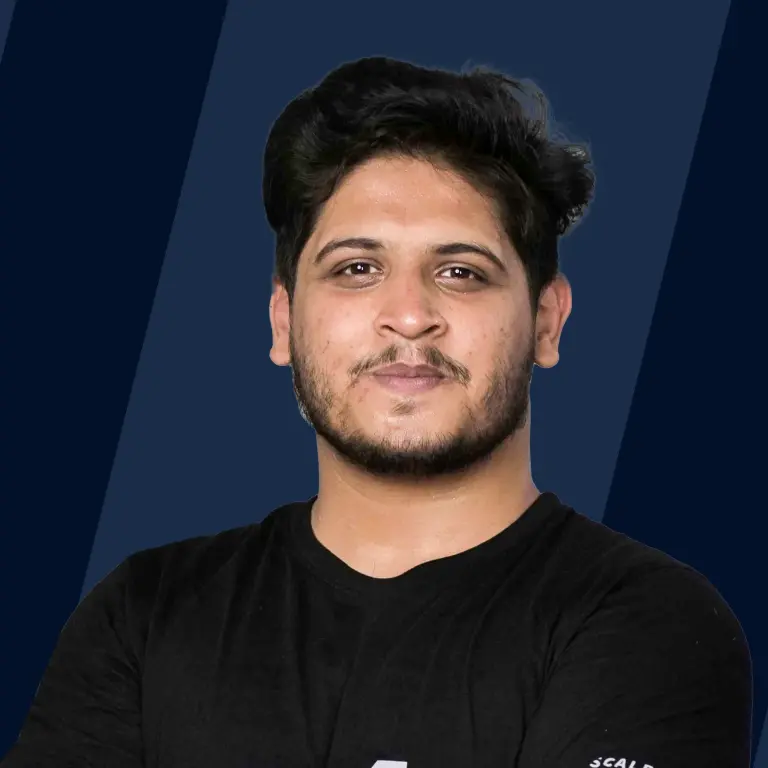
Overview
Handlebars.js is a logicless templating engine that keeps the HTML code and the logic part of the code separate and enables you to write cleaner code. Handlebars simplifies the workload by decreasing the number of loops, arrays, and statements you have to write to achieve what Handlebars can do with the templates.
Handlebars.js is a client-side (though it can be used on the server, too) templating engine for JavaScript. It is a JavaScript library that you include on your page just as you include any other JavaScript file. It is written in JavaScript, and Handlebars.js is a compiler that takes HTML and Handlebars expressions and compiles them into JavaScript functions.
How to Add Handlebars to your project
There are many ways in which you can add Handlebars to your project.
-
Because it is a JavaScript library, you can import using a local path, i.e., Handlebars can be loaded into the browser like any other JavaScript file, as shown below.
-
Handlebars is hosted on free CDNs as well. For example
-
You can also add Handlebars in your project via the npm route or yarn route. You can install it compiling the following command.
You can then use Handlebars using require.
-
Handlebars can be enabled by using other package managers as well. Like,
- Component
- Composer
- jspm
Using npm or yarn is the recommended way of using Handlebars. If you want to use the Handlebars templates in the web browser, you should use a built engine such as Webpac or Parcel because there are multiple plugins that allow you to use the Handlebars in the Webpack environment. Also, there is a Babel plugin that precompiles the Handlebars templates.
You can select any of above-mentioned methods, and once installed, you can dive into writing some templates and make your code cleaner.
How does it work
Handlebars.js is a compiler built with JavaScript. It takes the HTML code and Handlebars expression and compiles them into a JavaScript function. This function then takes one parameter, i.e., an object, and it returns an HTML string. So, in the end, you have a string(HTML) that has the values from the object properties inserted in the relevant places, and you insert this string on a page.
This sounds way more complex than it is, actually, so let’s take a closer look.
As shown in the image above, Handlebars take a template with the variables, and it compiles to a function.
This function is executed by passing a JSON object to it as an argument. This object is known as Context/Argument/Data. This context contains the values of the variables used in the template. This function returns the required HTML after replacing the variables of this template with the corresponding values after execution.
Now, let’s deep dive into the templates, expressions, and context to understand clearly the working of Handlebars.
Templates
Once you set up your library, you can start writing Templates. The recommended way to add Templates to your page is by including them inside the <script> tag, with an id and type attribute. The type attribute is important; otherwise, the browser will try to parse it as JavaScript(these are not JavaScript). Let’s write our first template.
Here {{name}} is called an expression. Expressions are covered later in this article.
Templates need to be compiled into a JavaScript function before use. After this template has been retrieved, then we can compile it by using Handlebars.compile() method, which returns a function. So first, you are going to compile this template which looks like this:
NOTE: In production, you will use a pre-compiler to reduce this extra step of compiling our template.
Here you are using a variable name, so you need to provide Handlebars with the value before it can return the finalized HTML. You can do this by passing a context to this template. Let’s take a look at the Context.
:::
Context
Every template you write has a context. A context is a JavaScript object that you pass to the compiled template. You can also change the context using a block expression which is covered later in this article.
Let’s create a context and pass it to the template created above.
Now, the value of the HTML is set to :
Expressions
You’re already familiar with the expressions as you saw in the examples above. The variables inside the templates are surrounded by double curly braces, i.e., {{}}, which are known as Expressions.
Example:
Here name is an expression that is used inside the template.
HTML Escaping
Handlebars can escape the values returned by the expression. If you don’t want Handlebars to escape the value, you have to surround variable using the triple curly brace, i.e. {{{ variable-name}}}.
Let’s take an example template to understand better.
The context created is
The finalized HTML will be
Output
Helpers
You can't write JavaScript directly within templates. Handlebars provides Helpers. By using Helpers, one can write JavaScript inside the Templates. A Handlebar helper is a function that you can call from your templates. To call a Helper, just call it as an expression, i.e., {{helper-name}}. You can also pass parameters here as well that will be passed to your helper function.
Handlebars let you create a custom helper function to accomplish the task you define. Let's take an example where you want the Handlebars to capitalize a variable. This can be achieved by creating a Helper function.
This is the example of capitalizing a name, but inside a helper function, you can do whatever you want and return a final output. When it is used in a template, it looks like this:
Block Helpers
Block helpers are just like the regular ones, but instead of working on a single variable, they work on a block. These helpers can modify the HTML and the content they are wrapped around.
To create a block helper, you again need to use Handlebars.registerHelper, but this time you are also using the second parameter of the callback function, i.e., options. Let's take a closer look at this.
Here the fn is called, which is the compiled template between the opening and the closing blocks of the helper, and then it is passed to the context, here this.
This is what the template looks like:
Summary
- Handlebars is a very powerful templating engine and can do wonders with your code if used correctly.
- There are multiple ways to include Handlebars in your project, but the recommended way is to use npm or yarn.
- It is important to include the type attribute inside your template; else, the browser will parse them as JavaScript, which it isn't.
- One cannot write JavaScript directly inside the Templates. You will need to use Helpers.
- Helpers are the functions that you can call from your templates.
- If you want more functionality with the helpers, then you can use Block Helpers.