__init__ in Python
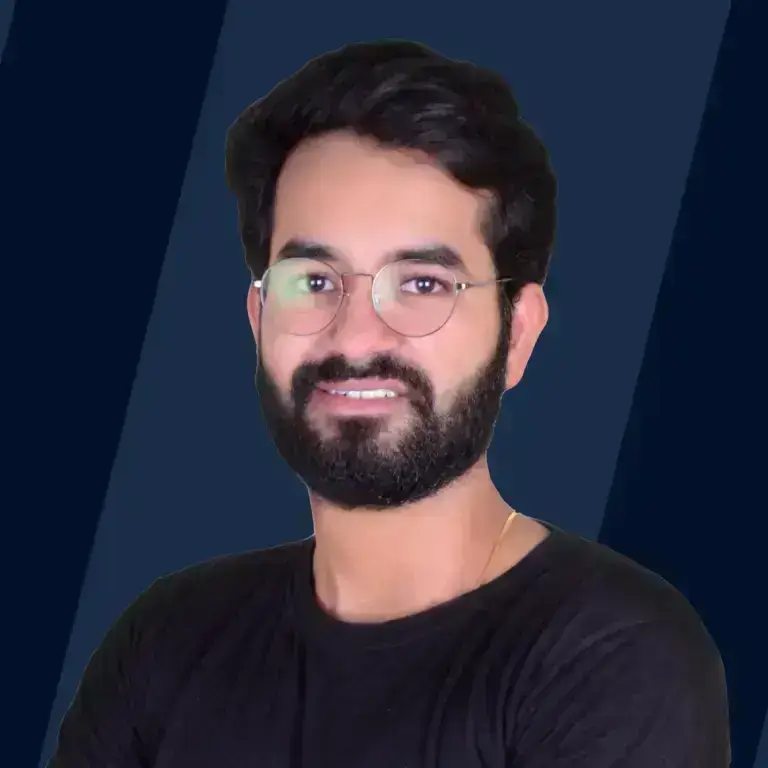
Overview
In an object-oriented approach, the __init__ method is the Python equivalent of the C++ constructor. Every time an object is created from a class, the __init__function is called. The __init__ method only allows the class to initialize the object's attributes. It is only used within classes.
What is init in Python?
Python is an object-oriented language. Whenever we create the object of our class, the the constructor of our class is called first. There is a method called __init__() for this task in Python. This method is called automatically whenever a new object of a class is created.
__init__ is a magic method called Dunder Methods (These are special methods whose invocation happens internally, and they start and end with double underscores). Other examples for magic methods are: __add__, __len__, __str__, etc.
How Does the init Method Work?
The init method in python is a special method used in the process of initializing an object. When you create a new instance of a class, the init method is automatically called. It simply initialize the class object.
Examples of init in Python
1. The Default init Constructor
Here is an example of a class Car that will clarify the functioning of __init__().
Code:
Output:
Explanation:
You can see in the output the order of print statements that __init__ is called first.
2. init with inheritance
Inheritance is a fundamental concept in OOPs. it is the way by which a class inherits the properties of another class. Here, the first class are called the base class or parent class, and the second class is called the derived class or children class.
The derived class can access the properties and methods of their parent class. In other words, the child class inherits all the methods and properties of their parent class and can add more methods and properties that are unique to them(child class) only. Inside a child class, __init__() of the parent class can be called to create objects and assign values. Here is an example:
Code:
Output:
Explanation:
In the above example, when the object of the Child class is created, __init__() of the Child class is called, which first calls the __init__() of the ParentClass. __init__() of the ParentClass prints out ‘Parent Class’ then print inside the Child class is executed.
Syntax of init in Python
Syntax:
__init__() function is defined just like a regular Python function. Self must be the first argument. After that, you can pass arguments of your requirements. The logic of the function comes in the function definition part.
Parameters in init in Python
It can take any number of arguments in it while the first parameter that will be passed in it will always be self.
Code:
Output:
Explanation:
In the above example, a class Trip is created, and an object ‘T’ is created to invoke the methods and attributes. As soon as the object is created, the__init__ method is initialized with values India and London, which are assigned to to_city and from_city, respectively. The self keyword represents the current instance of the object. So when the holiday() method is run, it prints out the values of from_city and to_city, which are London and India, respectively.
Return Value of init in Python
The init method of a class is used to initialize new objects, not create them. As such, it should not return any value. Returning None is correct in the sense that no runtime error will occur, but it suggests that the returned value is meaningful, which it is not.
Code:
Output:
Exceptions of init in Python
The init method in Python is the constructor for object initialization. It lacks a return value and is prone to TypeError if arguments are missing or exceed expectations. Default parameter values can be set to mitigate this. Attempting to access undefined attributes leads to an AttributeError. The init method is not mandatory, but if defined, it's crucial for proper object setup.
Conclusion
This article had a lot of information about the init method in Python. Here is a quick summary
- __init__() is a special Python method that runs when an object of a class is created.
- __init__() function is mostly used for assigning values to newly created objects.
- __init__() is a magic method, which means it is called automatically by Python
- __init__() can also be invoked manually.
- __init__() also supports inheritance.