intersection() in Python
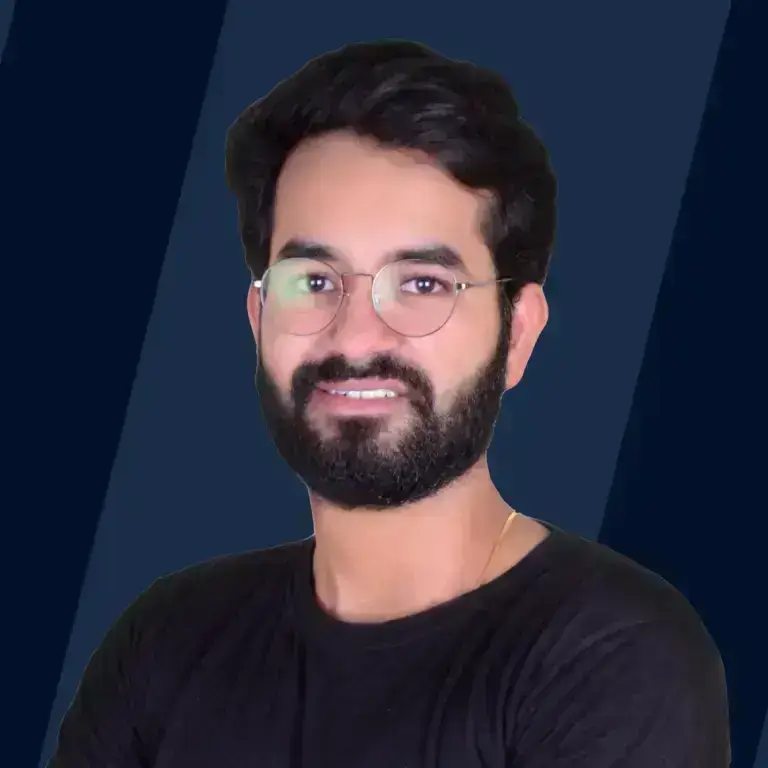
Overview
We can use a method called intersection in python and set intersection operator, i.e. &, to get the intersection of two or more sets. The set intersection operator only works with sets, but the set intersection() method can be used with any iterable, like strings, lists, and dictionaries.
Syntax for intersection() in Python
The syntax of the intersection method is quite simple. The intersection method takes sets as parameters.
set1 is required as set1 will be searched for the equal items. The other sets, such as set2, set3... are optional.
:::{.tip} Note: The syntax of & or intersection() operator in Python is:
Parameters for intersection() in Python
As discussed above, the intersection() method takes sets in the parameters. The intersection method can take any arbitrary number of sets (two or more) as parameters. Using these parameters, the intersection will generate a set of elements present in all the given sets.
Return Values for intersection() in Python
Using the sets passed as parameters to the intersection method, the intersection will generate a set and returns the generated set. The returned set consists of the elements present in all the given sets.
Exceptions for intersection() in Python
Usually, the intersection() method in Python does not raise an error if we use the correct syntax.
The intersection() function returns a set, which has the intersection of all sets(set_1, set_2, set_3 …) with set_1. If we do not pass any set in the parameter of the intersection() method, the intersection() method returns a copy of the calling set itself, i.e. set_1.
Example of intersection() in Python
Let’s take an example of two sets and try to find their intersection using intersection in python.
- Finding intersection in python using intersection() method:
Output:
Intersection of set-one and set-two is: {3, 6}
- Finding intersection in python using intersection operator(&):
Output:
Intersection of set-one and set-two is: {3, 6}
What is intersection() in Python?
The intersection of two sets is nothing but a set consisting of all the common elements of the two sets. To find intersection in python, we have two ways:
- using intersection() method for finding intersections in python
- using & operator for finding intersection in python
The intersection() method and & operator have the same performance. The only difference between the operator and method is that the set intersection operator only works with sets. In contrast, the set intersection() method can be used with any iterable, like strings, lists, and dictionaries.
Working of Set intersection() in Python
Suppose we are provided with two sets of values. So, the intersection of both sets is nothing but a set consisting of all the common elements of the two given sets.
Refer to the diagram given below to have a better understanding. In the example below, the common elements of both sets are 4 and 6. So, our answer is a set of {4, 6}.
As discussed above, for finding intersection in python, we have two ways:
- using intersection() method for finding intersection in python
- using & operator for finding intersection in python
Both the intersection() method and & operator have the same performance. The only difference between the operator and method is that the set intersection operator only works with sets, while the set intersection() method can be used with any iterable, like strings, lists, and dictionaries.
We will be learning about the intersection() method using examples and code snippets in this article.
More Examples
Let us take an example of more than two sets to better understand syntax and work on the intersection() method in python and the intersection operator in python.
Using python intersection() method:
Output:
Using Python intersection() operator:
Output:
We can see in the code above, the set_1 and set_2 have 'a' and 'c'. The set_2 and set_3 have only 'c' in common. So, When the intersection of set_1, set_2, and set_3 will be done, only 'c' will be returned.
Conclusion
- The intersection() method in python and set intersection operator, i.e. & is used to get the intersection of two or more sets.
- The syntax of intersection() method is:
- The set intersection() method can be used with any iterable, like strings, lists, and dictionaries.
- The set intersection operator only works with sets. The syntax of & or intersection operator is:
- The intersection() method can take any arbitrary number of sets (two or more sets) as parameters.
- The intersection() method returns a set consisting of the elements present in all the given sets.