set() in Python
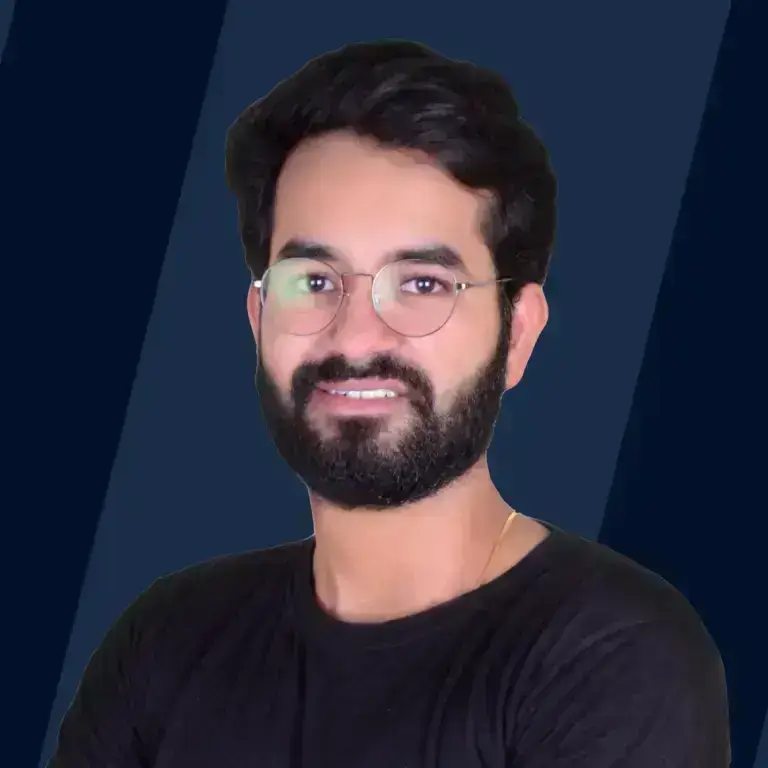
Overview
A set is a collection of unordered values or items. The set in Python has similar properties to the set in mathematics. The set in python takes one parameter. It is an optional parameter that contains values to be inserted into the set. The set in python creates and returns a set using the provided iterable values in the parameter of the set() function.
Syntax of set() function in Python
The syntax of set in python is as follows:
or
Note:
- We can put heterogeneous type of values inside the sets.
- In the second syntax, we need to pass values in the form of an iterable object or collection or sequence like list, tuple, string, set, dictionary, etc.
- If we do not provide any value in the {} in the first syntax, then an empty dictionary is created in the place of the empty set.
Parameters of set() function in Python
The set() function is used to create a set in python. The set in python takes one parameter. It is an optional parameter that contains values to be inserted into the set. The values in the parameter should be a b (string, tuple) or collection(set, dictionary), or an iterator object.
Return Values of set() function in Python
The set in python creates and returns a set using the provided iterable values in the parameter of the set() function. If we do not provide any parameter into the set function, the set() function returns an empty set.
Exceptions of set() function in Python
The set() usually does not raise errors and exceptions if we use proper syntax.
If we try to delete an element that is not present in the set, then the method will raise a KeyError.
Example of set() in Python
Let us try to see an example of set creation using both the syntax mentioned above.
Code:
Output:
What is a set in Python?
A set is a collection of unordered values or items. The set in python has similar properties to the set in mathematics. Let us learn some of the most important features of set in python:
- A set in python is an iterable object.
- A set in python does not contain duplicate values.
- A set in Python is an unordered collection of items.
- A set in python is an elastic object (mutable) object but the elements of the set in non-mutable.
- We cannot use indexing on the sets as no index is attached to any element in the python set.
- Slicing operation cannot be performed on the set in python because sets do not have indexing.
Since the set in python is inspired by mathematics sets, we can perform various mathematic-based operations on sets like intersection, union, set difference, complement, etc. Let us learn about these operations and other operations of the set in python.
Methods of Python Set
Let us learn about some of the most commonly used important methods of set in python.
Method | Description |
---|---|
union() | We can use a method called union in python and set union operator i.e. / to get the union of two or more sets. The set union operator only works with sets, but the set union() method can be used with any iterable, like strings, lists, and dictionaries. |
intersection() | We can use a method called intersection() in python and set intersection operator i.e. & to get the intersection of two or more sets. The set intersection operator only works with sets, but the set intersection() method can be used with any iterable, like strings, lists, and dictionaries. |
difference() | The set difference in python can be calculated using the python set difference() method and python set difference operator i.e. -. Both the python set difference method and operator returns a set containing elements that exist only in the first set, and not in both the sets. |
add() | The add() method in set is used to add some particular values or items into the set. The add() method adds a single element to the set. |
update() | The update() method in set is used to add some particular values or items into the set. The update() method adds a single element to the set. |
discard() | The discard() set method is used to some particular values or items from the set. If an element is not present in the set, then the discard() method does not change the set. |
remove() | The remove() method in the set is used to remove some particular values or items from the set. If an element is not present in the set, then the remove() method will raise an error. |
clear() | The clear() method is used to remove all the items from a set. |
pop() | The pop() method is used to remove an item from the set. The pop() method also returns the removed element. |
copy() | As the name suggests, the copy() method is used to return a copy of the set. |
More Examples
Now, let us take some examples to learn the working of the methods of set in python.
Modifying a set in Python
As we have learned, we can use update() and add() methods to modify a set. The add() and update() methods in the set are used to add some particular values or items into the set.
add() method
Code:
Output:
update() method
Code:
Output:
Note: Since 4 was already in the set, another Code: did not get inserted as sets do not contain duplicate values.
Removing elements from a set
We can use discard() and remove() methods for removing elements from a set.
discard() method
If an element is not present in the set, then the discard() method does not change the set.
Code:
Output:
Note: There was no nilesh present in the set, so no exception was raised using the discard() method.
remove() method
If an element is not present in the set, then the remove() method will raise an error.
Code:
Output:
Note: 11 was not present in the set, so an exception was raised using the remove() method.
Python Set Operations
Union of Sets
Let’s take an example of two sets and try to find their union using the union() method in python.
Finding union in python using union() method Code:
Output:
Finding union in python using union operator(|)
Code:
Output:
Intersection of Sets
Let’s take an example of two sets and try to find their intersection using intersection in python.
Finding intersection in python using intersection() method
Code:
Output:
Finding intersection in python using intersection operator(&)
Code:
Output:
Difference of Sets
Let us take the example of two sets namely set_1, and set_2. We will try to find their difference using the python set difference() method and python set difference operator.
Code:
Output:
Compare Sets
We can use comparison operators i.e. <, >, <=, >= , == to compare sets. By comparison of the set, we can find if a set is a subset, superset, or an equivalent set of the other or not. The comparison operators return true or false based on the provided sets.
Code:
Output:
Python Frozenset
The frozensets are used as keys in the dictionary data type because frozensets are immutable (i.e. items of a frozen set cannot be changed).
Lets us learn a few properties and example of frozenset in python:
- The elements of frozenset cannot be changed once created.
- Element(s) cannot be appended or added into a frozenset.
- The frozenset() method can be used to create frozensets in python.
Example:
Code:
Output:
Since frozenset is immutable so, the add() method cannot be used to add elements in a frozen set.
Other Set Operations in Python
Let us now learn a few more set operations of set in python.
Set Membership Test
The membership operator i.e. in and not in can be used to test set membership. If an element is present in the set, the set membership test returns true else returns false.
Code:
Output:
Iterating Through a Set
We can use loops for and while to iterate over sets in python.
Code:
Output:
Conclusion
- A set is a collection of unordered values or items. The set in python has similar properties to the set in mathematics.
- Syntax of set in python:my_set = set(iterable) or my_set = {value1, value2, value3, ...}
- The set in python takes one parameter. It is an optional parameter that contains values to be inserted into the set.
- The set in python creates and returns a set using the provided iterable values in the parameter of the set() function.
- If we do not provide any parameter into the set function, the set() function returns an empty set.
- If we try to delete an element that is not present in the set, then the method will return an error.
- A set in python is an unordered iterable object, which does not contain duplicate values.
- Slicing operation cannot be performed on the set in python because sets do not have indexing.