isNaN() in JavaScript
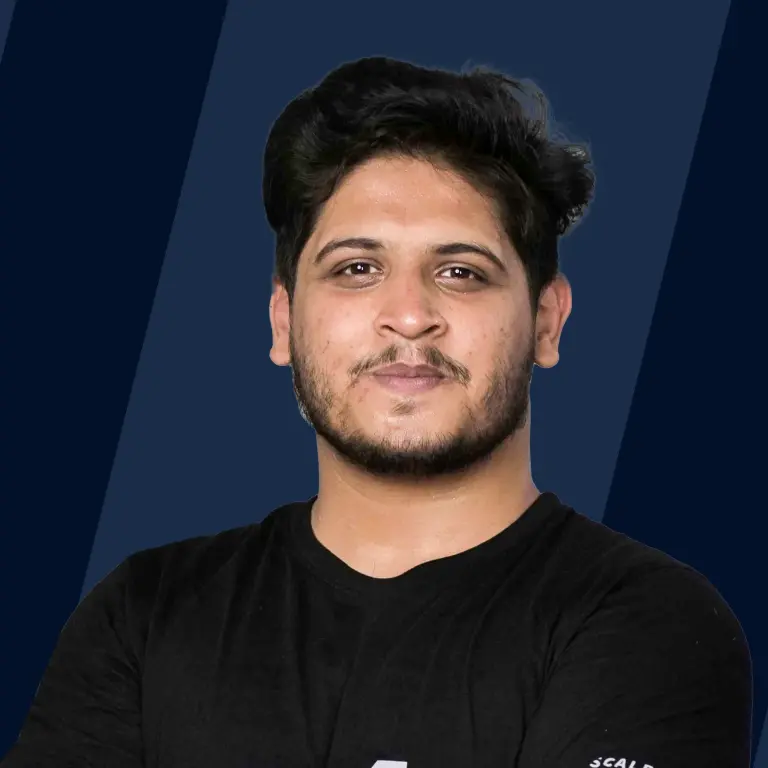
Overview
In Javascript NaN stands for "Not-a-Number". Hence here, isNaN() is a method in Javascript used to check whether a value is a number or not.
The method isNaN in JavaScript returns true if the given value is Not-a-Number, else it returns false.
Syntax of isNaN() in JavaScript
The following is the syntax of the method isNaN in JavaScript:
Parameters of isNaN() in JavaScript
The parameter of isNaN in JavaScript is:
- value: the value to be tested (whether it is a number or not).
Return Value of isNaN() in JavaScript
Return Type: boolean
The method isNaN() in JavaScript returns true if the given value is NaN (not a number). If not, then it returns false.
Example
Here we go through 3 examples, and we will explain their output one by one.
Output:
Explanation:
- In the first function, the argument is NaN directly. So isNaN() function returns true.
- In the second function, 0 is a number. Hence isNaN() returns false, which is printed on the screen.
- In the third function, 0/0 is the argument present. Like in mathematics, 0/0 is not a number at all, so the isNaN() function returns true.
What Is isNaN() in Javascript?
In Javascript isNaN() function is used to check if any value is NaN (which stands for 'Not-a-Number') or not. If the value passed is NaN, the function returns true else, it returns false.
If the parameter to the isNaN() function is not of type Number, it is first converted to a number before testing whether it is NaN or not.
The Convenience of an isNaN Function
Usually, we try to use the two operators, (== and ===) to check for equality.
However, these equality operators cannot be used for evaluating whether a value is NaN or not because NaN == NaN and NaN === NaN returns false, even though both values are Not-a-Number.
Example
Output:
Explanation: Here, we can see that, though both the values being compared against each other are NaN, but still both the if conditions are failing. Hence, equality operators cannot be used in exchange for the isNaN() function.
So we can use the isNaN() function conveniently to check for NaN value instead of using equality operators, which is misleading.
Origin of NaN Values
NaN values are generated when arithmetic operations result in undefined values which are not numbers. For example, dividing zero by zero results in a NaN value.
Confusing Special-Case Behavior
Let us go through an example:
We might expect that the return value to this function call should be true since an empty string ' ' is not a number at all.
However, the output is:
As mentioned before, if the argument is not a number, the given value is coerced to a numeric value.
The function should be interpreted as, "is the given value, when converted to a value of type Number, an IEEE-754 'Not A Number' value?"
Useful Special-Case Behavior
Using NaN in an arithmetic expression leads to the result of the arithmetic expression also being NaN. In order to prevent this, we can use isNaN to determine whether any variable in the expression is NaN or not. If it is NaN, we can use some default value or avoid the evaluation of the expression itself. For example,
Output:
Explanation: Here, b is NaN, so using it for the arithmetic operation of addition leads to c being NaN too.
So, in order to avoid this, we can use the isNaN() function in teh following way. Code:
Output:
Explanation: We see here that by assigning default values (by checking with the isNaN() function), we prevent c from being assigned a NaN value.
Using isNaN() Method with Other Functions
We can use this function to test whether values returned from parseInt and parseFloat functions are NaN or not. We elaborate on this below:
Output:
Explanation: parseInt() and parseFloat() functions convert a string to its corresponding integer and float values. '12' and '12.12' are converted to integer and float values, respectively. However, 'abc' cannot be converted to a numeric value. Hence we can use the isNaN() function to check whether the return value is NaN or not.
More Examples
How to use the isNaN() method in JavaScript?
We pass the value which we want to check whether it is NaN or not, to the function. Note that the value passed need not be a number only. It can be of any type, i.e., strings, characters, Date objects, etc. The function converts the value passed to numeric type before checking if the value is NaN or not.
Example:
Output:
Explanation: Here empty string('') and string with spaces(' ') is converted to 0 which is not NaN, hence the output is false.
Using isNaN() method to determine if a value is a number
Example:
Output:
Explanation:
- Here, '51' is a string, which is converted to the number 51, which is not NaN.
- false boolean value is synonymous with 0 integer value. So false is converted to 0, which is not NaN.
- 'abc' is converted to a number. However, parsing this as a number fails, and thus the function returns NaN.
- '17.43' is converted to the number 17.43, which is not NaN.
- The Date() object is converted to a numeric value, which is not NaN.
Browser compatibility
The isNaN method in javascript is supported by all the browsers such as Chrome, Edge, Safari, Opera etc.
Conclusion
- NaN stands for "Not-a-Number" and the function isNaN() in Javascript returns true if the value passed is NaN, else returns false.
- If the value passed to isNaN() is not of the type Number, then the value is converted to a Number and then checked.
- Traditional equality operators cannot be used to check whether a given value is NaN or not.
- We can use isNaN() in useful special cases and also along with other functions.