Arrays Class in Java
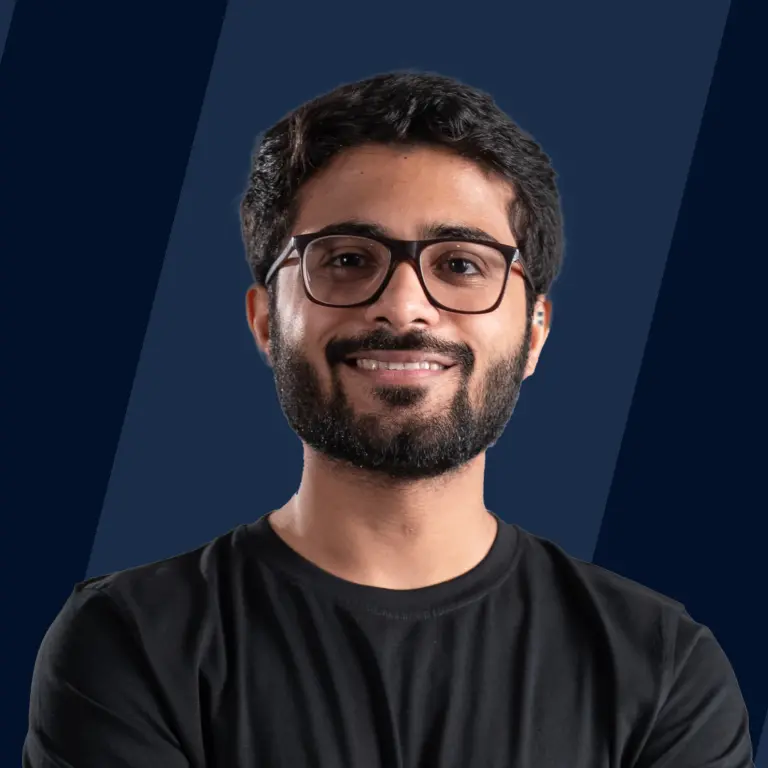
The arrays class is part of the Java collection framework in the java.utilpackage. It only consists ofstaticmethods and methods of theobject` class. Using Arrays, we can create, compare, sort, search, stream, and transform arrays. We can easily access the methods of this class by their class name.
Introduction to Arrays Class in Java
The Java Array class was introduced in Java version 1.2. Its methods are used mostly for manipulating an array, including all major operations like **creating, sorting, searching, comparing, ** etc. The arrays class provides overloaded methods for most data types. java.util.Arrays is a class that belongs to the java.util package.
Why do we need the Arrays class in Java?
This is because the methods provided by the Arrays class help us perform useful tasks on arrays conveniently without using loops.
Now, you might be wondering what the hierarchy of this class looks like.
The class hierarchy for Arrays class in Java:
The Arrays class is extended from the Object class, and the methods it provides are those of the Object class.
Let's take a look at the syntax for the class declaration and the usage of arrays.
Syntax: Class Declaration
Syntax: In Order to Use Arrays
Methods in Java Array Class
The static methods of the Java Array class could be used to perform operations like:
- Filling the elements
- Sorting the elements
- Searching for the elements
- Converting the array elements to String
- Many more...
Let's take a closer look at all the methods provided by the java arrays class. Then, we will discuss a few important ones in detail.
Method Name | Function |
---|---|
binary search() | It searches for an element contained in the sorted array by using Binary Search Algorithm. |
asList() | It is used to convert an array to a list of elements. |
binarySearch(array, fromIndex, toIndex, key, Comparator) | A range of specified arrays for the specified object is searched using the Binary Search Algorithm. |
toString(original array) | A string representation of the contents of the array is returned. This representation consists of a list of the elements of the array enclosed in square brackets ([]). A comma and a space separate the elements, and each element is converted to a string using the String. value of() function. |
stream(original array) | A sequential stream will be returned with an array that is specified by its source. The stream is a sequence of objects represented as a data channel with a source where the data is situated and a destination where it is transmitted. |
spliterator(original array,fromIndex, endIndex ) | A spliterator that covers the range of the specified arrays is returned. The Spliterator interface can be used for traversing and partitioning sequences. |
spliterator(original array) | A spliterator covering all the elements of the array is returned. |
sort(T[]a,Comparator <super T>c) | The specified array of the objects according to the order which is induced by the comparator is sorted by this. |
sort(originalArray, fromIndex, endIndex) | The specified range of the array is sorted in ascending order. |
sort(T[]a, int fromIndex, int toIndex,Comparator<super T>c) | The specified range of the specified array of objects according to the specified comparator is sorted. |
sort(original array) | The entire array is sorted in ascending order |
setAll(original array, functionalGenerator) | Sets the elements of the array using the generator function, which is already provided. |
parallel Sort(original array) | Sorts the concerned array using the parallel sort. |
paralleSetAll(originalArray, functionalGenerator) | All elements are set in parallel using the generator function. |
parallelPrefix(originalArray, operator) | For a complete array it performs parallelPrefix function. |
parallelPrefix(original array, fromIndex, endIndex, functional operator) | It performs parallelPrefix for the given range of the array with the specified functional operator. |
mismatch(array1,array2) | It will search and return the index of the first unmatched element between the 2 concerned arrays. |
hashCode(originalArray) | An integer hashCode of the array instance is returned. A hashcode is a unique integer that represents an object in Java. |
equals (array1, array2) | Will check if both the concerned arrays are equal or not. Two arrays are considered equal if both arrays contain the same number of elements and all corresponding pairs of elements in the two arrays are equal. |
fill(original array, fill value) | The fill value is assigned to each index of the arrays. |
deepToString(Object[]a) | A string representation of the deep contents and details of the concerned array is returned. |
deepHashcode(Object[]a) | This method will receive an array, compute hash code based on the deep content in an array, and return it in an integer. |
deepEquals(Object[]a1, Object[]a2) | It returns true only if the 2 concerned arrays are equal to one another. |
copyOfRange(originalArray, fromIndex, endIndex) | The concerned range of the concerned array is copied into the new array. |
copyOf(originalArray, newLength) | Copies the specified array, truncating or padding with the default value (if necessary) so the copy has the specified length. |
compare(array 1, array2) | Two arrays that are passed as parameters lexicographically are compared to each other. |
The table covered all the methods of the java.util.Arrays. Now, we will discuss a few commonly used methods in more detail.
Implementation of Arrays Class in Java
asList (Original Array)
Prototype:
Parameters:
- a – array of objects from which we get our list.
Return Value:
- List<T> => A fixed-size list of a specified array.
Code
Output
Explanation In the example above, we use the asList method of the Arrays class. We declare an Integer array and then pass it to the asList method to obtain a list.
binarySearch (Array, fromIndex, toIndex, Key, Comparator) Method
Prototype:
Parameters:
- a: The array to be searched
- key: The value to be searched for
Return Value:
- int: Sure, here's a rephrased version:
The index of the search key within the array is returned if it is present; otherwise, the formula (-(insertion point) – 1) is returned. The insertion point refers to the position where the key would be inserted into the array. This position is determined by finding the index of the first element greater than the key, or if all elements in the array are less than the specified key, it would be the length of the array. Importantly, the return value will be >= 0 only if the key is found in the array.
Code
Output
Explanation Here we sort the input array as we need a sorted array for our binarySearch method. Then we pass the array and the key to the binarySearch method. the index at which we find the key is displayed.
Note:
- If the input list is not sorted, the results are undefined.
- If there are duplicates, there is no guarantee which one will be found.
toString(Original Array) Method
Prototype:
Parameters:
- a: array whose string representation is required
Return Value:
- string: string representation of an array
Code
Output
Explanation Here, we use the toString method to convert the array to a string. Hence, we take an integer array. Then, we use the toString method, and the array is converted to the string representation displayed in the output.
copyOf (Original Array, newLength) Method
Prototype:
Parameters:
- original: the array that will be copied
- new length: length of our copied array
Return Value:
- A new array that is copied from the original and truncated with zeros depending on our specified length.
Code
Output
Explanation Here, we use the copyOf method of the Arrays class, which copies the input array into a new one.
copyOfRange (Original Array, fromIndex, endIndex) Method
Prototype:
Parameters:
- original: array from which values in the range will be copied
- from: first index of our range (inclusive)
- to: the last index of our range (exclusive)
Return Value: A new array that is copied from the original and truncated with zeros depending on our specified length.
Code
Output
Explanation Here, we use the copyOfRange and specify a range from 2 to 4. Thus, the output shows an array of two elements.
equals (array1, array2) Method
Prototype:
Parameters:
- a1: the first array that will be tested for equality
- a2: the second array that will be tested for equality
Return Value:
- Returns true only if both arrays are equal. Two arrays are regarded as equal if they have the same number of elements, and each pair of corresponding elements in the two arrays is identical.
Code
Output
Explanation Here, we use the equals method. We use two arrays and call the equals method to check if they are equal. Since they aren't equal, we get false as our result.
fill (Original Array, fillValue) Method
Prototype:
Parameters:
- a: array that will be filled.
- val: value that will be filled in all places of the array.
Return Value:
- None
Code
Output
Explanation This example shows the basic usage of the 'fill' method. We fill our entire array with a key value, and here, the value is 23. Hence, the output received is an array with values of 23.
sort (Original Array) Method
Prototype:
Parameters: a: array that is to be sorted.
Return Value:
- None
Code
Output
Explanation Here, we use an array and call the sort method to arrange the elements of the array in ascending order, sorting the array. Hence, we get the sorted array as our output.
hashCode (Original Array) Method
Prototype:
Parameters:
- a: array whose hashcode will be computed.
Return Value:
- int: Hashcode of the array, a hashcode is a unique integer that represents an object in Java.
Code
Output
Explanation Here, we use the 'hashCode' method, which helps us compute the hashcode for the array we pass in as the argument.
deepEquals (Object[] a1, Object[] a2) Method
Prototype:
Parameters:
- a1: the array that is to be tested for equality.
- a2: the other array that is to be tested for equality.
Return Value:
- Returns true if both the arrays are equal; otherwise, it is false.
Code
Output
Explanation Here, we use the deepEquals method, and the output we receive is false because the arrays aren't equal.
Now that we have understood the implementation of these methods, let's take a look at the benefits of the Arrays class in Java.
Benefits of Arrays Class in Java
- Arrays class makes it easier to perform common operations on an array.
- No need to use complicated loops to work with an array.
- No need to reinvent the wheel for binary search and sorting operations.
Here's a quick summary of all that we have dealt with in the article.
Summary
- java.util.Arrays are a class that belongs to java.util package.
- Arrays class consists of static methods that make it easier to work with an array.
- The static methods of the Java Array class could be used to perform operations like
- filling the elements (fill(originalArray, fillValue))
- sorting the elements (sort(originalArray, fromIndex, endIndex))
- searching for the elements (binaySearch())
- converting the array elements to String (toString(original array))
- Many more…
- We can use these methods as follows: Arrays.<function name>;
FAQs
Q: What is the java.util array?
A: java.util.arrays is a class that belongs to the java.util package. This class provides various methods for manipulating arrays and their types, such as sort (), binarySearch (), equals (), etc.
Q: What does Arrays.sort () method do in Java?
A: The Arrays.sort () method allows us to sort the elements of the array depending on the comparator. The array has many overloads that, when in use, can perform sorting in Java. It has different overloads for sorting different primitive data types.
Q: Which sorting is used in Arrays.sort() in Java?
A: Arrays.sort uses dual-pivot Quicksort on primitives. It offers O(nlog(n)) performance and is typically faster than traditional (one-pivot) Quicksort implementations. However, it uses a stable, adaptive, iterative implementation of the mergesort algorithm for Array of Objects.
Q: What are collections and arrays classes?
A: Collections are generally dynamic(capable of action ) in nature, and the class Collections helps us with direct methods that act upon the collections. Arrays are static and have class arrays that provide us with methods to manipulate them. But we can not term these as direct methods, i.e. Array objects can't invoke these methods. Rather, array objects need to be passed as an argument to these methods
Q: Is array an object in Java?
A: In the Java programming language, arrays are objects. These objects are dynamically created and may be assigned to variables of type Object. All methods of class Object may be invoked on an array.