String Comparison in Java
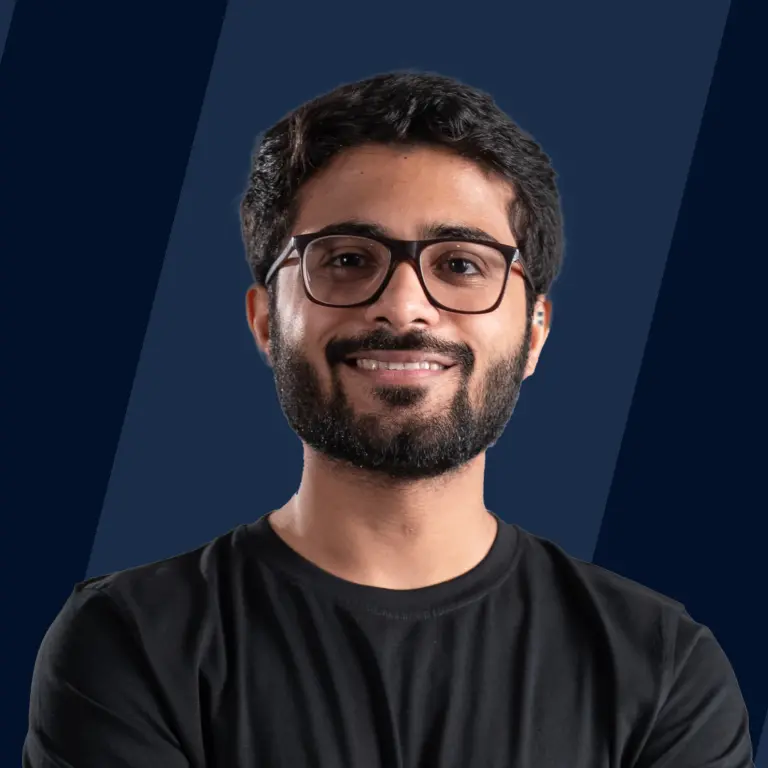
Overview
In Java, objects of the String class contain an array of characters that are immutable i.e. they remain constant and cannot be altered once created and initialized. In real-time, there are various situations where we need to compare two String objects. So, there are multiple ways provided in Java to compare the String objects such as:
- String.equals() method
- String.equalsIgnoreCase() method
- Object.equals() method
- String.compareTo() method
- String.regionMatches() method
How to Compare Two Strings in Java?
There are six ways discussed below to compare two String objects:
- Using User-defined Function
- Using String.equals()
- Using String.equalsIgnoreCase()
- Using Objects.equals()
- Using String.compareTo()
- Using String.regionMatches()
1. Using User-defined Function
We can define a custom method to compare two String objects as follows:
- The method takes two String objects as arguments and traverses through each element of both the string objects to evaluate the equality between them.
- The function searches and tries to find an unmatched value between the two strings. The function returns positive or negative integer values according to the difference between the first unmatched character in both the strings if strings are unequal else zero.
Example:
Output:
Explanation:
-
User-defined function:
- A user-defined function is created which takes two String objects as parameters. The minimum length of both the strings is calculated and a loop runs till the minimum length.
- String objects are traversed using the loop. ASCII values of characters of both strings are calculated and compared for each index.
- When any unmatched character gets encountered then the difference of their ASCII values is returned from the function.
- If there is no unmatched character then there are two conditions, whether one string is the subset of the other or both the strings are equal.
-
Main function: In the main function, 4 strings are compared to one another in the following ways:
- When string objects s1 and s4 are passed to the function. The function returns zero which implies that both the strings are equal.
- When s1 and s3 are passed, the unmatched values are ‘S’ and ‘s’ encountered at index 0 and therefore the function returns -32 which is the difference between ASCII values of 'S' and 's'.
- When s3 and s1 are passed, the unmatched values are ‘s’ and ‘S’ encountered at index 0, and therefore the function returns 32.
- When s1 and s2 are passed, all the values get matched but the lengths of the strings are not equal. Therefore the function returns -6.
Observation:
- If the first string is greater than the second string, a positive result is returned.
- If the first string is less than the second string, a negative result is returned.
2. Using String.equals()
In Java, the String.equals() method compares two strings based on the sequence of characters present in both strings. The method is called using two strings and returns a boolean value. If all the characters of both the strings are equal then the method returns true else false gets returned.
Example:
Output:
Explanation:
- In the above code, 4 string objects are created, initialized, and then passed to the equals() methods in the following manner:
- When string objects s1 and s4 are passed to the equals() method, it returns true which implies that both the strings are equal.
- When s1 and s3 are passed then, it returns false which implies that both the strings are unequal. The first character in both the strings ‘S’ and ‘T’ are not the same and hence both the strings are unequal.
- When s1 and s2 are passed then, it returns false which implies that both the strings are unequal. s1 is a subset of s2 and hence are unequal.
3. Using String.equalsIgnoreCase()
In Java, the String.equalsIgnoreCase() method compares two strings based on the sequence of characters without considering their case (lower or upper) present in both the strings. It is called using two strings and returns a boolean value. If all the characters of both the strings are equal ignoring their cases then the method returns true else false gets returned.
Example:
Output:
Explanation:
- In the above code, 6 string objects are created, initialized, and then passed to the equalsIgnoreCase() methods in the following manner:
- When string objects s1 and s4 are passed to the equalsIgnoreCase() method, it returns true which implies that both the strings are equal irrespective of their case. However, both of them have the same case and set of characters.
- When s1 and s3 are passed then, it returns true which implies that both the strings are equal irrespective of their case. Here, both strings have different cases.
- When s1 and s2 are passed then, it returns false which implies that both the strings are unequal irrespective of their case. s1 is a subset of s2 and hence they are unequal.
- When s1 and s5 are passed then, it returns false which implies that both the strings are unequal irrespective of their case. s1 and s5 are very different strings and hence are unequal.
- When s1 and s6 are passed then, it returns false which implies that both the strings are unequal irrespective of their case. s1 and s6 have a different character set as well as are in different cases and hence are unequal.
4. Using Objects.equals()
- In Java, the Objects.equals() method compares two objects based on elements present in both objects.
- It takes two objects as parameters and returns a boolean value. If all the elements of both objects are equal then the method returns true else false gets returned.
- If both the objects are null then true gets returned. If only one of them is null then false gets returned.
Example:
Output:
Explanation:
- In the above code, 6 string objects are created, initialized, and then passed to the Objects.equals() methods in the following manner:
- When string objects s1 and s4 are passed to the Objects.equals() method, it returns true which implies that both the strings are equal.
- When s1 and s3 are passed then, it returns false which implies that both the strings are unequal. The first character in both the strings ‘S’ and ‘T’ are not the same and hence both the strings are unequal.
- When s1 and s2 are passed then, it returns false which implies that both the strings are unequal. s1 is a subset of s2 and hence they are unequal.
- When s1 and s5 are passed then, it returns false which implies that both the strings are unequal. Here, only one of the strings is null. s5 is initialized as null while s1 is not null.
- When s5 and s6 are passed then, it returns true which implies that both the strings are equal. When both arguments are null, true is returned.
5. Using String.compareTo()
In Java, the String.compareTo() method compares two strings based on the sequence of characters present in both strings. It is called using two strings and returns an integer value. It returns three types of integral values:
- Zero(0): If both the strings are equal, then the method returns zero.
If the string objects passed as arguments are not equal, then the following cases arise:
- If one of the strings is a prefix of the other, the difference between the lengths of the first and second arguments is returned.
- Otherwise, the difference between the ASCII values of the first mismatched characters is returned.
Note:
ASCII values are the 7-bit character encoding standards used in electronic communication devices which consist of 128 characters.
Example:
Output:
Explanation:
In the code, 4 strings are declared, initialized, and then passed to the compareTo() methods in the following manner:
- When string objects s1 and s4 are passed to the compareTo() method. The method returns zero which implies that both the strings are equal.
- When s1 and s3 are passed, the unmatched values are ‘S’ and ‘s’ encountered at index 0 and therefore the method returns -32.
- When s3 and s1 are passed, the unmatched values are ‘s’ and ‘S’ encountered at index 0 and therefore the method returns 32.
- When s1 and s2 are passed, then all the values get matched but the lengths of strings are not equal and the method returns -6 (the difference between the lengths of Scaler and ScalerTopics).
6. Using String.regionMatches()
- In Java, the String class contains regionMatches() method that is used to check equality between two strings or substrings by matching their respective set of characters.
- The method is used in two formats, one is case-sensitive form and the other is case-insensitive form. It takes 5 parameters and returns a boolean value.
The 5 parameters used by the method are:
- ignoreCase: If the value of ignoreCase attribute is passed as true then the case-insensitive form of regionMatches() method will be used.
- toffset: It represents the index from where the comparison of the string will begin.
- other: The other string that needs to be compared with is depicted by the attribute.
- ooffset: It takes the index from where the comparison in the other string will begin.
- len: It points towards the number of characters required to be matched.
The regionMatches() method returns boolean values according to the following conditions:
- If ignoreCase is True: The method will return:
- true: If both the strings are equal irrespective of their case.
- false: If both the strings are not equal irrespective of their case.
- If ignoreCase is False: The method will return:
- true: If both the strings are equal having the same case.
- false: If both the strings are equal but have different cases or both of the strings have different characters irrespective of the case.
Example:
Output:
Explanation:
In the above code, 6 string objects are created, initialized, and then passed to the regionMatches() methods in the following manner:
- When string objects s1 and s4 are passed to the regionMatches() method if
- ignoreCase is false, it returns true.
- ignoreCase is true, it returns true. Both of them have the same case and set of characters.
- When s1 and s3 are passed to the method if
- ignoreCase is false, it returns false.
- ignoreCase is true, it returns true. Both strings have different cases but the same set of characters.
- When s1 and s2 are passed to the method if
- ignoreCase is false, it returns false.
- ignoreCase is true, it returns false. s1 is a subset of s2 and hence are unequal.
- When s1 and s5 are passed to the method if
- ignoreCase is false, it returns false.
- ignoreCase is true, it returns false. s1 and s5 are very different strings and hence are unequal.
- When s1 and s6 are passed to the method with
- ignoreCase is false, it returns false.
- ignoreCase is true, it returns false. s1 and s6 have different character set as well as are in different cases and hence are unequal.
Why Not to Use '==' to Compare Strings in Java?
In Java, It is recommended to use methods instead of == operator for comparing two strings. This is because the == operator verifies whether the two objects belong to the same memory location or not. On the other hand, the methods compare the strings represented by these String objects.
Example:
Output:
Explanation:
- In the above code, two String objects having the same characters are compared with the == operator and then with the equals() method.
- Since, both the string have different memory locations ‘==’ operator returns false while the equals() method returns true as both the strings have the same values.
Conclusion
- In Java, There are various ways to compare two string objects. A user-defined function describes the proper code for comparing two strings.
- String.equals(), Objects.equals() and compareTo() methods are used for comparing case-sensitive strings.
- equalsIgnoreCase() method is used for comparing case-insensitive strings.
- regionMatches() method is used for case-sensitive as well as non-case-sensitive comparisons.