What is Global Variable JavaScript?
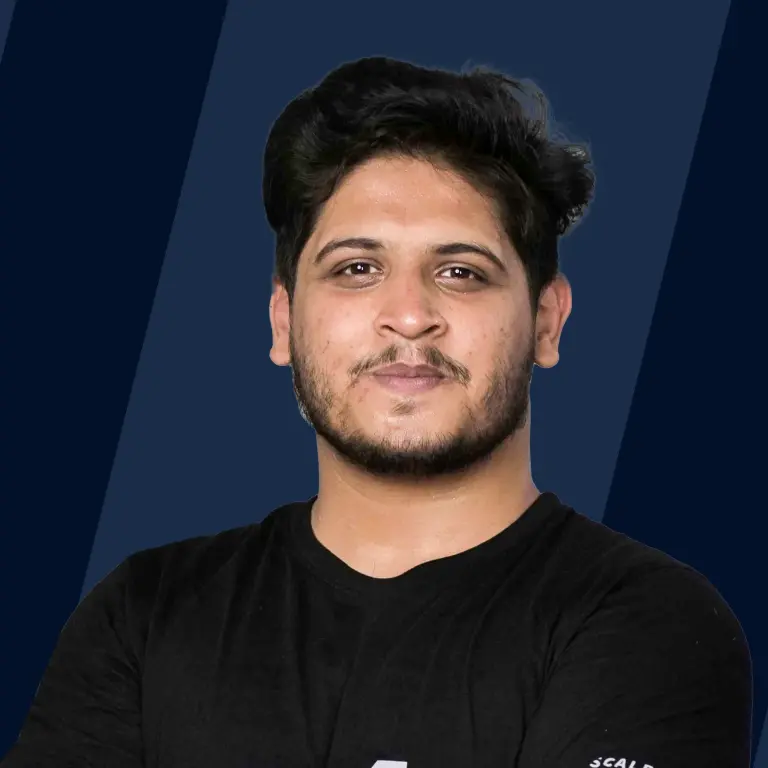
A global variable in javascript is a variable having global scope, meaning, it can be accessed from anywhere inside the programme.
Syntax
What is Global Scope?
Scope of a variable stand for where it will be available to use inside the programme. Variable having global scope are accessible everywhere inside the programme.
What is Automatically Global?
When we initialize a variable without it's declaration first, it results in making that variable global automatically. The reason behind this behavior is, initialization without declaration is the same as initializing the variable into the window object like this window.myVal = 10 in the code below.
Note: In "Strict Mode", initializing a variable without its declaration first doesn't make the global variable automatically.
Example-
Output-
Above, we have a function myFun which is getting called, inside the myFun function myVal get initialized a value, but myVal is not declared first. As result, myVal will automatically become a global variable. Printing the myVal on console outside it's scope prints 10 as output, which verifies that myVal is a global variable now.
How does JavaScript Global Variable work?
When a global variable in javascript is declared it get moved on top of the global scope, i.e. it gets into call stack immediately in the starting, due to hoisting, where it is available to use everywhere inside the programme.
Example-
Output-
Above, we have a global variable myVal which is used in two consecutive functions addOne and print. In addOne function we added 1 into the myVal and prints the value of myVal on console in the print function.
Examples
- Using a global variable to keep track of display theme-
Output-
Above, we have a global variable themeVal having the value of display color which is "dark". Then two if conditional blocks are calling the darkTheme and lightTheme functions based on condition. As result, will be called darkTheme and print the "current value of theme is dark" on the console as output.
- Declaring an automatic global value-
Output-
Above, we have a function add in which myVal get assigned a value of 100 without declaration of myVal first. As result, when add function get called myVal becomes a global variable, and prints "My value is 100" on console as output when display function get called.
How to declare Global Variables in JavaScript?
To declare a global variable in javascript we have to declare a var, const or let variable outside of any block or function, inside the global scope.
Example-
Output-
Above, we declare global variable myVal and assigned a value of 10. myVal is accessible inside the myFun function also which verifies that myVal is a global variable. Use this Online JavaScript Formatter to format your code.
What’s the Difference Between a Global var and a window.variable in JavaScript?
To declare a global variable using global var, we have to declare a var variable into the global scope. But, to declare a global variable using window.variable, we have to explicitly write window. followed by variable name and value like this window.variableName= value.
Example of global variable declared using global var -
Above, we declared a global variable using global var and assigned a value of 10.
Example of global variable declared using window.variable -
Output-
Above, we have a function myFun in which we declared a window.variable and assigned a value of 20. Here, both the examples will get assigned at the same place which is window object.
Variables in javascript are the building block of any program, it helps to give the named reference to any types of data used in the program, learn more about JavaScript Variables.
Conclusion:
* Global variable in javascript is a variable declared in the global scope.
- It is available to use everywhere inside the programme.
- Variable assigned a value without it's declaration first become a global variable.
- To declare a global variable in javascript we can use the global variable method or window. Variable.