What is a Nullish Coalescing Operator or Double Question Mark (??) in JavaScript?
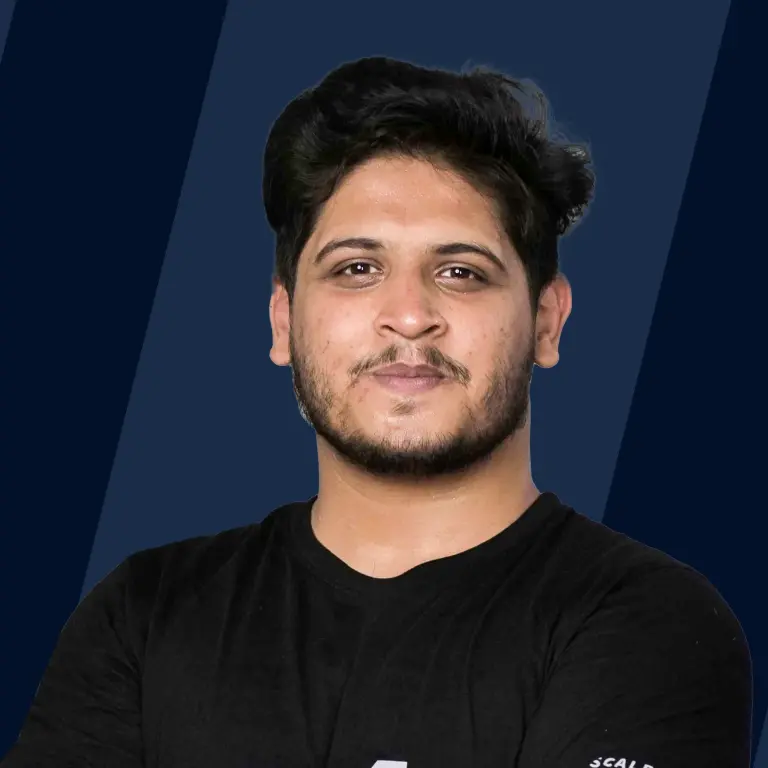
What is a Nullish Coalescing Operator or Double Question Mark (??) in JavaScript?
The Nullish coalescing operator is a new feature introduced in this ECMA proposal and is denoted by the double question marks (??). Javascript double question mark is a logical operator that takes two values and returns the right-hand value if the left-hand value is undefined or null, Else returns the left-hand operand.
Javascript double question mark can simply be explained as a special case of the logical OR ( || ) operator. When the left-hand side operand is any false value, not just null or undefined, the right-hand side operand is returned in Nullish coalescing case. Operator precedence for the nullish coalescing operator is the fifth-lowest. Higher than the ternary operator and directly lower than the || operator.
Syntax
Nullish coalescing is denoted by double question marks (??).
- The result of leftExpr ?? rightExpr will be:
- if leftExpr is defined, then leftExpr,
- if leftExpr isn’t defined, then rightExpr.
In simple words, ?? returns the left argument if it’s not null/undefined. Otherwise, the right one. When a passed parameter is less than the number of parameters specified in the function prototype, the value is called "undefined".
Let’s see an example:
Values That are Considered to be Falsy Values in JavaScript
If a value is encountered in a Boolean context, it is referred to as having a falsy value (also written falsey). The only six values in JavaScript that are considered as false values are the ones listed below.
- false: The keyword false.
- undefined: The primitive value.
- null: The absence of any value.
- ""(empty string): Empty string value.
- NaN: Not a number.
- 0: The Number zero (so, also 0.0, etc., and 0x0).
Why JavaScript Needed the Nullish Coalescing Operator?
The || operator is very useful. However, there are times when we only want the following expression to be evaluated when the first operand is either null or undefined. Therefore, ES11 has introduced the nullish coalescing operator or javascript double question mark.
The logical OR operator (||) is often used to set a variable's default value. For example:
The value variable in the above example is undefined, therefore, coerced to be false. Hence, the result is 5.
However, the logical OR (||) operator can be confusing when you consider empty strings "" or 0 as valid values, as in the following example:
You might not expect the result to be 5, but it is 5, not 0.
By using the nullish coalescing operator or javascript double question mark, these pitfalls can be avoided. When the first value is either null or undefined, it will only return the second value.
Examples
Using the nullish Coalescing Operator
In this example, we'll keep values other than null or undefined but provide default values instead.
Explanation
We initialized three variables to store the number, empty string, and null value; after using the javascript double question mark, we can see the default value as output only for the variable with the null value.
Assigning a Default Value to a Variable
Earlier, it was a common practice to use the logical OR operator || to assign a default value to a variable:
However, || is a boolean logical operator, and any false value (0, ", NaN, null, or undefined) was not returned because the left-side operand was forced to be a boolean for the evaluation. This condition can face unexpected consequences like if you consider '', 0, or NaN as the valid values.
By only returning the second operand when the first operand turns out to either undefined or null, the nullish coalescing operator avoids this pitfall:
The nullish Coalescing Operator is Short-circuited
If the left-hand side turns out to be neither null nor undefined, the right-hand side expression is not evaluated, similar to the OR and AND logical operators.
Explanation
- Define three functions X() which returns undefined, y() which returns false and Z() that returns a string.
- As we can see on X() ?? Z(), X() returned undefined, so both expressions were evaluated.
- However, Y() returned false; hence the right-hand side expression was not evaluated.
No chaining with AND or OR operators
Directly combining the logical AND or OR operator with the nullish coalescing operator will result in a SyntaxError, as in the following example:
However, you can prevent this error by explicitly specifying the operator precedences by enclosing the expression to the left of the ?? operator in parentheses:
Relationship with the Optional Chaining Operator (?.)
The optional chaining operator (?.) and the nullish coalescing operator treat both null and undefined as specific values, making it useful for accessing object properties that may be null or undefined.
Explanation
As we can see, the optional chaining operator (?.) transformed the characters to uppercase without giving the error for the undefined value someBarProp.
Browser Compatibility
The browsers supported by JavaScript Nullish Coalescing Operator or javascript double question mark are listed below:
Browser | version |
---|---|
Chrome | 80 |
Edge | 80 |
Firefox | 72 |
Opera | 67 |
Safari | 13.1 |
Don't just learn JavaScript; get certified in it! Enroll Scaler Topics JavaScript course and validate your expertise in this essential programming language.
Conclusion
- Nullish coalescing operator is a new feature introduced in this ECMA proposal and is denoted by the double question marks ??.
- Javascript double question mark is a logical operator that takes two values and returns the right-hand value if the left-hand value is undefined or null, else returns its left-hand value.
- Operator precedence for the nullish coalescing operator is fifth-lowest. directly lower than || and directly higher than the conditional (ternary) operator.
- Nullish coalescing operator is written as two question marks ??. If a value is encountered in a Boolean context, it is referred to as having a falsy value (also written falsey).
- The only six values in JavaScript that are considered false values are undefined, null, NaN, 0, "" (empty string), and false, of course.
- The nullish coalescing operator treats both null and undefined as specific values, making it useful for accessing object properties that may be null or undefined.
- Javascript double question mark can simply be explained as a special case of the logical OR ( || ) operator. When the left-hand side operand is any false value, not just null or undefined, the right-hand side operand is returned in the Nullish coalescing case.