JavaScript Anonymous Functions
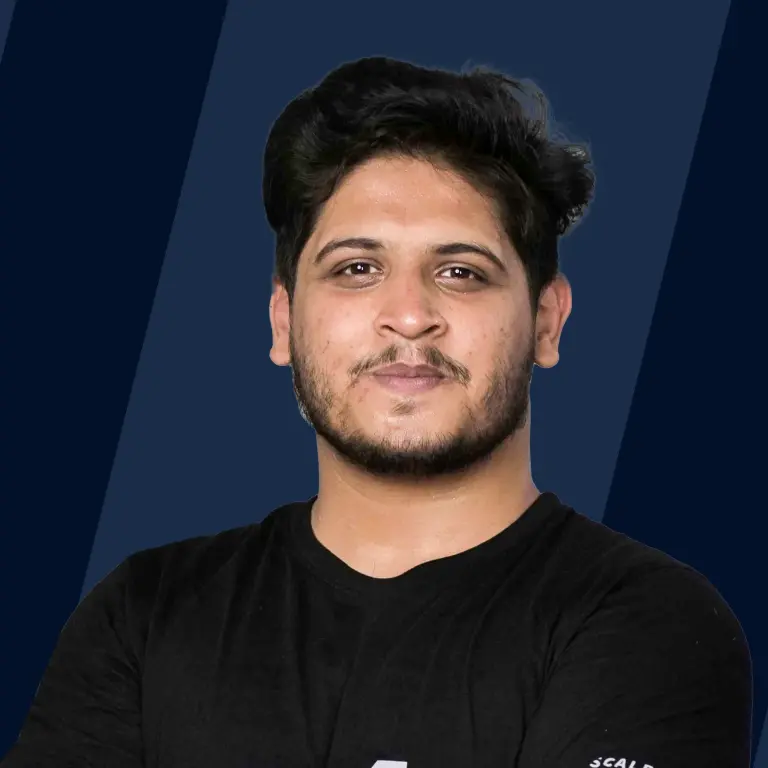
Overview
"Anonymous" as the name suggests, is something that does not have any "name" or "identity". Similarly, anonymous functions in javascript are functions that do not have any name. Arrow functions are also anonymous functions with a compact syntax. Named functions are simply functions, that have some name associated with them, you can consider them as the normal javascript functions we use.
There are several applications of anonymous functions, which we will explore as we go ahead in this article.
What are Anonymous Functions in JavaScript?
Anonymous functions in JavaScript are the functions that do not have any name or identity. Just like, you have a name by which everyone calls you or identifies you. But, the anonymous functions, do not have any name, so we cannot call them like any other function in JavaScript.
Let us take an example:
Output:
Explanation:
If you try to run this function, it will result in an error saying Function statement requires a function name. And, that is completely legit, since ES6 says, you cannot have a function without a name in JavaScript. But then, how do we create anonymous functions, if they do not have any name?
Let's see how.
Syntax
Below given is the syntax for the anonymous function in JavaScript --
Explanation:
We write the anonymous function in Javascript by writing function() followed by the parentheses, where we may pass the parameters, and skipping the function name. Then in a pair of curly braces {}, we write our desired code. Finally, we assign this function to a variable. We can later use the variable whenever we need the value of the anonymous function.
Does Anonymous Functions Always Need to Store their Value Inside Variables?
An anonymous function in javascript is not accessible after its initial creation. Therefore, we need to assign it to a variable, so that we can use its value later. They are always invoked (called) using the variable name.
Also, we create anonymous functions in JavaScript, where we want to use functions as values. In other words, we can store the value returned by an anonymous function in a variable. In the above example, we stored the value returned by the function in the variable variableName.
Key Takeaways:
- Anonymous functions do not have any name
- We can write an anonymous function using the function keyword, followed by parentheses ().
- We can write the function statements, just like we do for any other javascript function inside the curly parentheses {}.
- We store the results returned by an anonymous function in variables.
Implementation of an Anonymous Function in JavaScript
Below given is an example to demonstrate the usage of the anonymous functions in JavaScript -
Example 1:
Output:
Explanation:
In the above example, we wrote a function that logs "Example of anonymous functions", and please note we skipped the function name here. Then we assigned the function to a variable demo. Once we call demo() in line 4, we see it logs the value as expected.
So, this is how we write code for anonymous functions in javascript.
Now, let us take one more example to further illustrate our learning.
Example 2:
Explanation:
This is a very common use case of the anonymous functions in JavaScript. Here, we have attached an "event listener" to the anonymous function. An eventlistener is something, which responds to any event. An event can be anything such as when we click any button or hover on some component of any website. So, performing any such activities on a webpage triggers an event.
The above anonymous function is called whenever a button is clicked. The anonymous function takes a callback function event and listens to the event. And, once the button is clicked, it alerts the message "Button is clicked!".
Now, let's take one last example - Let us create an unnamed function, which calculates the area of a rectangle.
Example 3:
Output:
Explanation:
In this example, we calculate the area of a rectangle. To do so, we create an anonymous function, which accepts two parameters - (length, breadth). After calculating the value, this function returns it to the variable area. To call that function, we simply call the variable, passing the arguments area(10,5) that the anonymous function expects. We store the result of this variable in x. Finally, we log the result into the console.
Function Expression vs Function Declaration
A JavaScript function can also be defined using an expression. A function expression can be stored in a variable. After a function expression has been stored in a variable, the variable can be used as a function.
They are of 2 types:
- Named function: The named function expressions are like normal functions with names, but are assigned to a variable.
- Unnamed function: The unnamed function expressions, do not have any name. They are anonymous functions.
Example:
Function declaration is a normal function that has a function name and function statement. A declared function is “saved for later use”, and is executed later when it is invoked (called). Function Declarations must begin with “function” and have a name.
Use of Anonymous Functions in JavaScript
There are multiple uses of anonymous functions in javascript. Let us look at some very important and popular usage of the same.
Using Anonymous Functions as Arguments of Other Functions
Functions are called first-class citizens because, they can be passed as an argument to any function, or also returned by any function. The best use of anonymous functions is that they can be passed as arguments to other functions.
Let us briefly understand this using an example.
Example 1:
Output:
Explanation:
In the above example, greet() is a function that expects another function as a parameter. So, we have passed an anonymous function to greet() where it returns "Good Morning". In the actual greet() function we get the value returned by the anonymous function in the parameter wish. Then, we log the value by calling the function wish() followed by our message. So, this is an example where we pass an anonymous function as the argument to another function.
Let us take another very popular example of passing an anonymous function to a function. You must have used setTimeout(), which is a method that expects a function and the number of milliseconds after which it will execute the function. Now, let us see how we can pass an anonymous function to setTimeout().
Example 2:
Output:
Explanation:
In the setTimeout() function, have we passed an anonymous function. It will run after a delay of 5 seconds since we have passed 5000ms as the second argument to the setTimeout() function.
Immediately Invoked Function Execution
Sometimes, we need to execute a function right after we declare it so that we don't need to go through the hassle of calling it. In these situations, anonymous functions are a great option since they execute right when declared.
An IIFE (Immediately Invoked Function Expression) is a JavaScript function that gets invoked immediately. In simple words, it runs as soon as it is defined. It is a way to execute functions immediately, as soon as they are created.
Syntax
Explanation:
IIFE is a design pattern that is also known as a Self-Executing Anonymous Function and contains these major parts:
- The first part of IIFE is the anonymous function -- check in the image, where we have only used the function keyword followed by parentheses - function (), and no name.
- The second part is the parameters list, where we can optionally provide a parameter
- The third part is the function body, where we can write our function statements, enclosed in curly parentheses {}.
- The fourth, and most important part is the () parentheses, which help to create the immediately invoked function expression. Using them, the JavaScript engine will directly execute the function.
Let us understand this better with an example.
Example 1:
Output:
Explanation:
In the above example, we created an anonymous function, which is supposed to alert some messages. We defined the function and passed the appropriate parameter to it. Without having us call the function, it directly alerts the message once we execute our code.
Let us break the code into parts, and understand:
- First, the following defines a function expression:
- After that, we call the function by using the trailing parentheses (). We may optionally pass some arguments too to them if the function expects. In this example, the function expects a parameter so, we pass it.
Example 2:
Output:
Explanation:
In this example, we implement a very good use case for the IIFEs. We have counted from 1 to 5 in JavaScript, putting a 1-second timer between every time we log a message. So, we iterate from 1 through 5, and pass the value of each iteration i in the IIFE:
Then, inside our code, we use the setTimeout() for our operation. We maintain a timer, using i, which waits for 1000*i seconds before executing our code. After that, our function logs the message, with the count value and number of seconds after every second.
What Would Happen if we had not Used IIFE here?
In that case, our code looked like this:
Output:
So, in that case, we would get a result like this. It is because of the asynchronous code we have here. Whenever JavaScript encounters an asynchronous code, it postpones the callback's execution until the asynchronous task has finished. However, this is not under the scope of this article. But, you have seen how well IIFE solved this problem.
Arrow Functions in JavaScript
Arrow functions are another great use case for anonymous functions in javascript. Arrow functions provide a shorthand way of declaring anonymous functions. They were introduced as part of ES6. They are very compact and allow us to write shorter, leaner code. However, there are certain limitations of arrow functions, which makes them not suitable to be used in some cases.
Syntax
Arrow functions are represented by an arrow-like structure, which is equal to the = sign followed by a greater than symbol >. In simple words, => is the representation for arrow functions.
We write the parameter list in (), followed by the arrow =>. After that, we write the function statements enclosed in parentheses {}.
Let us see the syntax in detail -
- If we have just one parameter:
We can just write the parameter name(without any parentheses). If we have a very simple expression then we may skip the return keyword and enclose braces {} as well.
- We can have any number of parameters in the parameter list. If we have multiple parameters:
We can write the parameter name. And the code statement, for simple expressions, the return keyword may be omitted.
- We can also have 0 parameters, in that case, our syntax becomes:
- If we have multiple parameters and multi-line code statement:
In that case, we can write the parameters enclosed in braces, followed by the arrow => like symbol. And, write our code statements inside the curly braces. Also, optionally we may return the result as we do for any other javascript function.
Note: If we have a multi-line code statement, and suppose we need to return a value from the function, then it is mandatory for us to use the return keyword. However, we may skip return if we have a single line of code statement.
Examples of Arrow Functions
After having studied in detail the arrow functions in JavaScript, let us now see some examples which will further enhance our understanding.
Example 1:
Output:
Explanation:
In this example, we have an arrow function that expects only one parameter, a. So, we may optionally skip the enclosing parentheses of the parameter. Then we write the => symbol, representing an arrow. In the function definition, we compute the square of any number as a * a, which is a single line code, so again we may optionally omit the return keyword. We assign the result of this arrow function to a variable x. We call that variable passing the required argument to execute this arrow function.
Example 2:
Output:
Explanation:
In this example, we have used our arrow functions to display an alert based on the response given by the user. It is a simple yet very efficient use of arrow functions where we can prevent writing a completely new function for this simple task.
Example 3:
Output:
Explanation:
In this example, we try to calculate the power of any number a raised to the power n. Please note that here we pass 2 parameters, so we cannot omit the () braces. Then we write the arrow-like symbol representing the arrow function. We compute the power and return the result. We assign this arrow function to the variable x. To call this, we can use the variable passing to it the arguments list: x(10,3).
How is It a Shorthand for Anonymous Functions?
A "shorthand" is a shorter way to express something already available in another syntax in our code. Arrow functions can be used to express anonymous functions in a much shorter and more compact way. Let us take an example to understand this.
Suppose we have written this code using the anonymous function:
Example 1: Anonymous function
We can write the same using arrow functions in a more compact way like this:
Arrow function:
Explanation:
So, here we can omit the "function" keyword for arrow functions and also the curly braces for writing this single line of code, which makes arrow functions shorter and crispier to write. However, since they make our code one-liner, sometimes, it becomes a bit difficult for us to understand.
Example 2: Anonymous function
Let us write this using arrow functions:
Arrow function:
Explanation:
This is a very short and simple written code in arrow functions, where we can omit the return keyword and the parentheses because of this one line of code and one parameter.
However, anonymous functions cannot be used in certain situations due to the limitations which come with them. It is not in the scope of the tutorial because it is a topic in itself.
Conclusion
Here we come to the end of the tutorial with a thorough discussion on anonymous functions in JavaScript, named functions, and arrow functions. Let us now recap what we have learned in this tutorial:
- Anonymous functions in javascript are functions without any name or identity and are best used for cases when you want to invoke a function directly after defining it. Also, when you just want to use a function only once.
- Anonymous functions in javascript are declared inline, and the code written is more readable to the users.
- Named functions are given a name and are like the regular javascript functions. They are very helpful when you want to reuse your functions multiple times in your code. Also, they are pretty much easier to debug than anonymous functions.
- Arrow functions are a kind of anonymous function that provides shorter syntactical forms for normal javascript functions. They are concise to write and simpler to read.