JavaScript Constructor Function
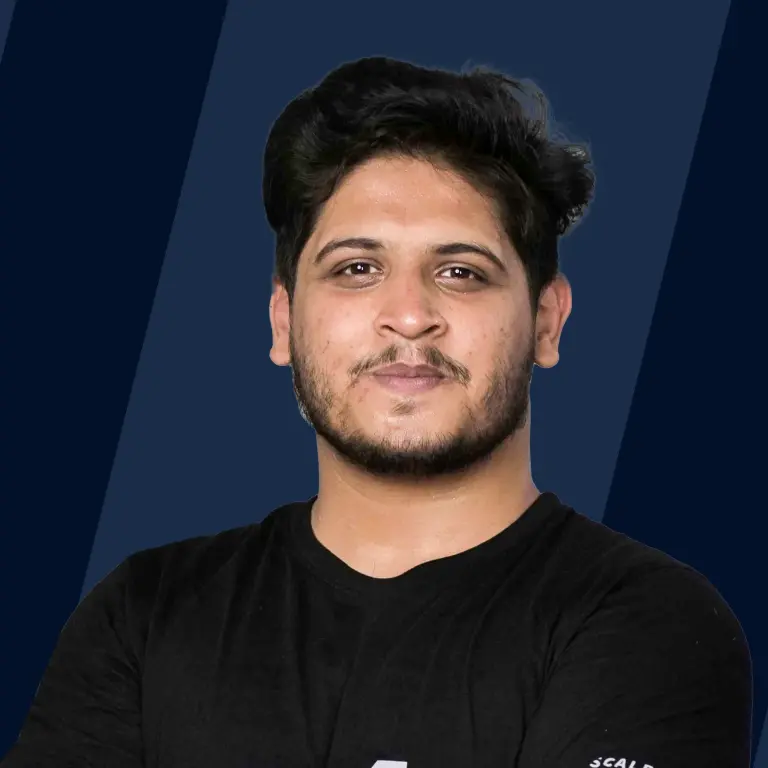
Constructors in Javascript are a special kind of function used to create and instantiate objects, especially when multiple objects of the same kind need to be created. A constructor defines the object properties and methods. Constructors can be created in two ways using Constructor Functions or using Constructor Methods. We use the new keyword to call a constructor. Javascript also provides built-in constructors such as Array, Object, etc.
Syntax
A constructor is created similarly to a regular function, the function keyword followed by the name and arguments.
The convention is that the first letter of a constructor's name should to capitalized.
Syntax
Example:
Takeaway: A constructor function is created in the same way as a regular function except that the first letter of its name should be capitalized.
What Happens When a Constructor is Called?
We have seen that to use a constructor we have to call it now let's see what happens under the hood of that process: When we use the new keyword the following things happen:
- An empty object is created
- this is set to the newly created object
- The constructor is called
- Inside the constructor the given properties and values are bound to the newly created object by using this.arg = arg
- The constructor returns this which means the object is returned since this is currently set to the newly created object.
Takeaway: When a constructor is called a new empty object is created, the this value is set to this object inside the constructor the properties and values are updated in the object using this keyword, and finally the constructor returns this keyword.
Examples
Example 1: Using a Constructor Function
Output:
Example 2: ES6 Class Constructor
Output:
Example 3: Constructor Inheritance
Output:
Adding Properties and Methods in an Object
In JavaScript, you can add properties and methods to an object dynamically, even after the object has been created. This flexibility is one of the core features of JavaScript and allows you to modify objects as your program runs. You can add properties (variables) and methods (functions) to objects, which is particularly useful for customizing and extending the behavior of objects.
Adding Properties
To add a property to an object, you can simply assign a value to a new property name using dot notation or bracket notation. For example:
Adding Methods
You can add a method (function) to an object in the same way you add a property. Methods can be functions that operate on the object's data. For example:
Adding a Method to a Constructor
We can add methods to Javascript constructor functions similar to how we have added other properties which are by using the this keyword. Let us understand this with an example
Output:
Explanation:
- Inside the constructor we first define the function i.e:
function() { console.log("Welcome", this.name) }
- Then we have assigned it a variable welcome
welcome = function() { console.log("Welcome", this.name) }
- Finally we bind it to the object using this
this.welcome = function() { console.log("Welcome", this.name) }
- When the constructor is called whichever object is present in this will invoke the method accordingly hence we see that both student1 and student2 were able to invoke the method.
Takeaway: Methods can be added to Javascript constructor functions similar to how we have added other properties which is by using the this keyword.
JavaScript Built-in Constructors
Javascript also provides some built-in Constructors. Such as:
- Object - Creates new Object object
- Array - Creates new Array object
- String - Creates new String object
- Number - Creates new Number object etc.
Example:
Output:
Takeaway: Javascript has in-built constructors such as Object(), Array(), String(), Number() etc.
Browser Compatibility
Constructors are a fundamental concept in JavaScript and have been supported in all modern browsers. However, the compatibility of certain features or syntactical sugar related to constructors might vary. Here is a brief explanation of browser compatibility issues you might encounter:
Class Syntax: The class syntax for constructors was introduced in ECMAScript 6 (ES6). Some older browsers may not fully support ES6 features. However, it's generally not a significant issue as ES6 features are widely supported in modern browsers.
Class Static Methods: Some older browsers may not support static methods in classes. Static methods are methods called on the class itself rather than on instances of the class.
Arrow Functions: Arrow functions introduced in ES6 have different behavior regarding the this keyword. In constructor functions or methods within classes, they might not behave as expected, especially in handling the this context.
Example:
In this example, Person is a constructor function that takes name and age as parameters and assigns them to the object's properties using this.
Instances of the Person object are created using the new keyword.
Output:
Both person1 and person2 are instances of the Person constructor and have their own name and age properties.
In modern browsers, this example should work without any compatibility issues. However, if you're dealing with older browsers, you might need to consider potential issues as mentioned earlier
Conclusion
- Constructors in Javascript are a special kind of function used to create and instantiate objects, especially when multiple objects of the same kind need to be created.
- Constructors can be created in two ways using Constructor Functions or using Constructor Methods.
- A constructor function is created in the same way as a regular function except that the first letter of its name needs to be capitalized.
- To use a constructor we need to use the new keyword this will invoke the constructor.
- Javascript has in-built constructors such as **Object(), Array(), String(), Number()` etc.
- A prototype is a default object present in all functions and objects in Javascript it can be used to add methods and properties to an object.