JavaScript Static Methods
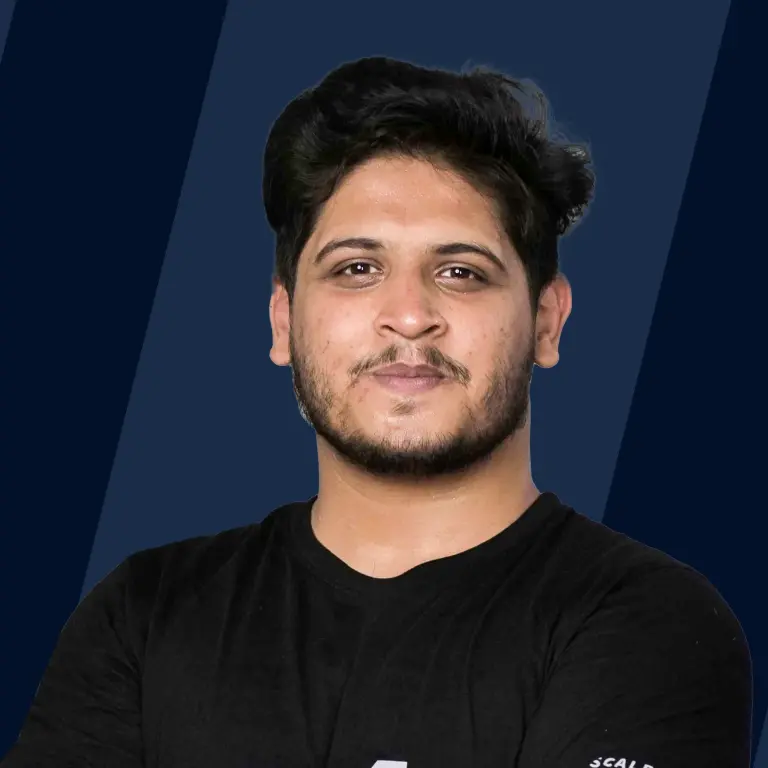
Overview
A static method in JavaScript is a method that has a static keyword prepended to itself. Such methods cannot be accessed through instantiated objects but could be accessed through the class name. This is because static methods belong to the class directly. Inheritance even applies to static methods. Also, these methods can be invoked by non-static methods and even constructors. Static methods are used to create utility functions and create objects that contain default information.
The Urge to Use Static Method in JavaScript
Before we jump into the actual usage of the static method, we need to know the urge that makes us use the static method. Consider that we have a class called Square, and a method called findPerimeter, defined as:
Now, let's make two objects of type Square, square1 and square2:
If we wish to find the perimeter of the first Square object, then we can find it by doing square1.findPerimeter(). It would give us the required answer.
It is worthwhile to notice that, in the definition of the method findPerimeter, the keyword this is used. The this keyword in the method refers to the object through which the method would be invoked. Hence, it would be fair to say that when the method is invoked, the method gets bound to the object through which the invoking is happening.
Now, sometimes we have a situation where we wish to invoke a method, but we do not want the method to be bound to an object.
By the way, will that kind of situation ever arise?
The answer is Yes.
Let's say we wish to compare the perimeter of two squares we created above, square1 and square2. But, before we just jump into creating the method called comparePerimeter, we should ask ourselves whether this method, when invoked, should be bound to an object or not. The answer is obviously No because one reason is that it is not possible to bind the method to two objects simultaneously.
Then, to which entity will the method be bound?
The answer is the class.
Now comes the role of the static method. Static methods are those methods that are bound to the class. We can also say that static methods directly belong to the class.
What is a Static Method in JavaScript?
A static method is a method that is defined in a class using the static keyword. Such a method turns out to be slightly different than a usual JavaScript method. The way the static method is different is that it cannot be accessed by any of the instantiated objects of the class. Rather, the static method can only be accessed using the class name because it directly belongs to the class.
Before knowing how to invoke a static method, it is essential to know its syntax.
Syntax for Static Method in JavaScript
To define a static method in JavaScript, we need to prefix the static keyword before the definition of the method.
Suppose, we have a JavaSript method sendMessage defined inside a class Chat:
And now we wish to convert the above sendMessage method into a static method. Then we rewrite the class as follows:
Accessing Static Method in JavaScript
First, we will see the wrong way of accessing the static method and then the right way. Knowing the wrong way first will make us aware of the approach that does not work.
Wrong Way to Access Static Method in JavaScript
Suppose we create an object out of the class Chat as:
And now, we wish to use this instantiated object to invoke the static method sendMessage. Then if we do:
We will get an error saying myChat.sendMessage is not a function. This shows that accessing the static method is not possible through objects.
Right Way to Access Static Method in JavaScript
On the other hand, if we access the static method sendMessage using the class name Chat directly, then:
Gives us the returned string "You got me!". This shows that the static method can be accessed by using the class name directly.
Relationship between Static Method and Class
In order to understand the practical relationship between static method and class, we will continue with the example of class Square and static method comparePerimeter, which we saw at the beginning of this article. This time we would implement the static method inside the class:
Output:
Now, if we wish to compare the perimeters of the two Square type objects, then the expression Square.comparePerimeter(square1, square2) gives us the result as the "Second square has more perimeter". So, we see that we were able to successfully access the static method through the class name. This is because static method directly belongs to the class.
Relationship between Static Method and Object
There is no direct relationship that exists between static method and object. Objects are not allowed to access a static method. Technically speaking, this happens because whenever a static method is defined in a class, the static method essentially gets stored inside the class's constructor. A static method is not stored inside the class's prototype and hence becomes inaccessible for the objects. In JavaScript, objects can access only those members of a class that are stored inside the class's prototype.
The picture below articulates the idea:
Static Methods and this
Essentially, a static method belongs to a class. Hence, when we use this keyword inside the body of static method, then the this keyword refers to the class itself. The idea becomes clear in the following example:
In the above example, we have a static method checkThis defined inside a class Chat.
Now, if we do:
We get the answer as true. This proves that this keyword inside a static method refers to the class that defined it.
Accessing Static Members from Another Static Method
Yes, even accessing static members from another static method is possible. Such accessing is achieved using the this keyword. Suppose, we have a class User and three static members are defined in the class:
Output:
Now, if we try out User.loginHelloEmail(), we get the above output. So, we see that the static method loginHelloEmail can access static property helloEmail and static method checkValidEmail through this keyword.
Accessing Static Members from a Class Constructor and Non-Static Methods
First, we will see how to access static members from a class constructor, and second, we will see how to access them from non-static methods.
Accessing Static Members from a Class Constructor
Well, a class constructor can access a static member. Such access can be achieved through the class name. The example below depicts the idea:
As we can see above, the constructor is accessing static property helloEmail and static method welcomeMessage through the class name User. Now, to execute the constructor, we need to create an object. So when we do:
Output:
We are shown hello@company.com and Welcome to our team! as the output.
Accessing Static Members from Non-Static Methods
Non-static methods can access static members through class name just the way we saw in the case of the constructor in the previous sub-section. The example below depicts the idea:
So, in the above code, we see that the non-static method greet is accessing the static property helloEmail and static method welcomeMessage through the class name User. So if we do:
Output:
As shown above, it would give us hello@company.com and Welcome to our team! as the output.
Memory Allocation of Static Method in JavaScript
Since the static method belongs to a class and is not accessible through objects, this means that the memory for the static method is allocated only once and is not dependent on the number of instantiated objects that are created. This also implies that the static method is not shared among instantiated objects because such a method cannot be invoked through any of those instantiated objects.
Why Do We Need Static Method in JavaScript?
As discussed at the beginning of this article, the idea of having a static method in JavaScript comes when we wish to associate the method to a class rather than to any instantiated object. There could be two possible use cases (but not limited to) where we wish to have a static method:
To Create Utility Functions
Let's see an example to understand how a static method could be used as a utility function. Suppose we have a class User defined as:
And we have instantiated numerous objects of class User. Now, if we wish to have a method for deleting a certain instantiated object, then declaring that method as a static method would be a good decision:
So, suppose we need to delete an object called user1, then User.delete(user1) will do the needful.
Now, we may argue that the same deletion task could be performed by a non-static method.
But, we need to understand here that deleting an object should be done by a method that is more related to the class whose type the object is. This makes things more meaningful. Just like when we wish to create any variable in JavaScript, we strive to give it a name that ultimately describes what that variable does. Similarly, when we see that a method we are going to define would bear a relation to the class rather than an instantiated object, we declare that method as static.
To Create Objects Containing Default Information
Consider the following static method createDefault inside a class User:
So, whenever we wish to create an object with default information, we need not create that object by ourselves. Instead, we can just invoke createDefault static method, and we will get a new object with that information.
Such a method is helpful when we want to create that kind of object, but we do not know what is the default information that object would contain. Hence, if we do console.log(User.createDefault()), it would show us:
Here, in this use case, again, we see that invoking the method using the class name is creating some meaning. The meaning is that when we write the expression User.createDefault(), we come to an understanding that the method is going to create something of class type User.
Difference between Static Method and Non-Static Method in JavaScript
Static method | Non-static method |
---|---|
1. Belongs to the class directly | 1. Belongs to the class indirectly |
2. Cannot be invoked through instantiated objects | 2. Can be invoked through instantiated objects |
3. Keyword this, when used inside the method, refers to the class | 3. Keyword this when used inside the method, refers to the object through which the method is invoked |
Points to Remember about Static Methods in JavaScript
1. A method can be made static by just prepending static keyword to it.
2. Static method is a method of a class but not of the instantiated object.
3. Static method cannot be accessed by instantiated objects.
4. Static method can be accessed using the class name.
5. Technically speaking, the static method gets stored inside the class's constructor but not the class's prototype.
6. Static methods are not at all shared with instantiated objects.
7. Whenever a static method is defined, its memory is allocated only once, and the memory is independent of the number of instantiated objects.
8. Static methods are generally used to create an object containing default information or for creating utility functions.
9. Inheritance works for static methods too.
Static Properties
In JavaScript, apart from having static methods in a class, we can also have static properties in a class. Static properties differ from usual properties in the same way static methods differ from usual methods that we saw in previous sections.
Syntax for Static Property in JavaScript
Let's say, we have a class Chat and a property message.
But, we wish to have that property message as a static one. So we rewrite the class as follows:
Accessing Static Property in JavaScript
Static property in JavaScript can be accessed through the class name. For instance, in the above example, if we wish to access the static property message, then we would access it as Chat.message. Hence, if we do console.log(Chat.message), it would show us "Have a great day!" as the output.
Inheritance of Static Methods and Properties
Even static methods and properties could be inherited. Let's say we have a superclass called User and a subclass called Customer:
Output:
In the above code, the superclass User has two static members. One is a static property score, and another one is a static method sayHi. We see that the class Customer is declared as the subclass of class User.
So, if we try out console.log(Customer.score) and console.log(Customer.sayHi), we would be shown 20 and Hello, you see I am inherited as well respectively. This means that class Customer is inheriting the static property and method of class User.
Conclusion
- To make a method static, we just need to prepend static to its definition.
- A static method is not a method of an individual object, but it is the method of the class itself.
- A static method directly belongs to the class.
- Static method could not be accessed by instantiated objects.
- Static method could be accessed using the class name.
- this keyword, when used inside a static method, refers to the class that defined the static method.
- A method is defined as static when that method is going to have an association with the class rather than an object.
- Memory for the static method is allocated only once, irrespective of the number of objects that are instantiated.
- Static method is not shared among instantiated objects.
- Static methods could be used to create utility functions and an object containing default information.
- Static methods and properties are inherited as well.