JavaScript try...catch
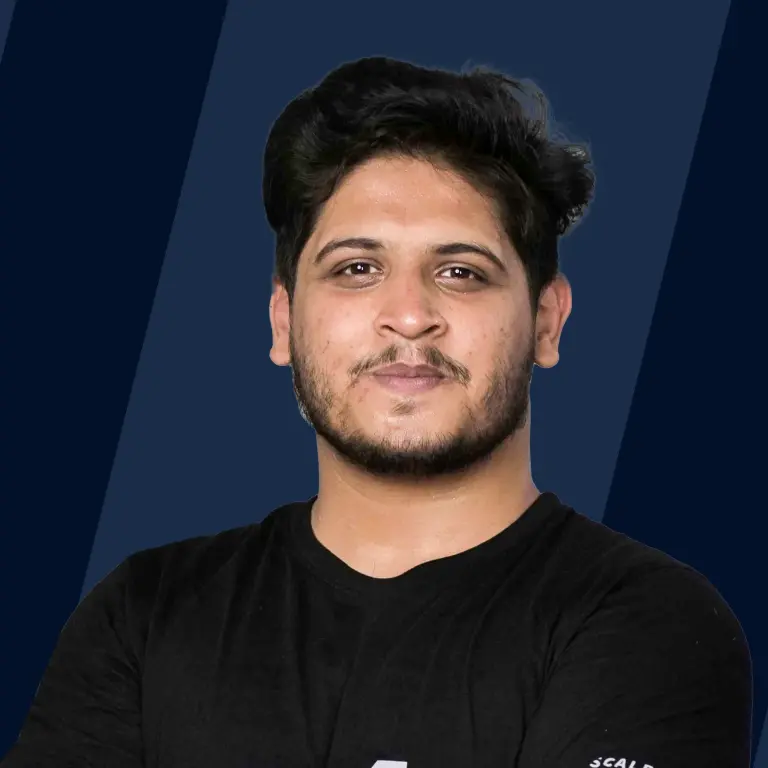
Overview
A try...catch statement is a standard construct in many computer languages. In essence, it is utilized to manage the code's prone to errors. It first checks the code for any flaws it might have, then it takes steps to fix any errors (if occur). The try...catch statements are a wonderful place to hold complex code when programming.
What is a try...catch Block in JavaScript?
A try catch in javascript is a very commonly used statement in various programming languages. These statements are used to manage and manipulate that particular section of the code where errors exist. The try-catch in javascript first tests for all the possible errors that may be contained in the code, and then the try-catch in javascript takes various actions to handle these errors (if there exists any error). We should always keep the complex code in a try-catch statement. The try statement and catch statement in javascript come in pairs. Now let us discuss the try catch in the javascript statements below.
Try{} Statement
The try{} statement in a try catch in javascript is used to define a particular block of code that needs to be tested during the execution of the program. If there are any errors present in that code, then the try{} statement passes it to the catch{} statement block. After that, the actions that need to be taken are done.
Catch{} Statement
The catch{} statement is used for defining a block of code that gets executed when there is any error in the code tested by the try{} statement. There can be two types of handlers present in this catch{} statement. The first one is the exceptional handler and the second one is the built-in handler. The catch block gets executed only when there is any error present in the try block and the error needs to be rectified. Otherwise, the catch block gets skipped. The catch block gets executed only after the execution of the try block. The syntax of the try and catch statement is as follows:
Flowchart That Illustrates the Flow of the try...catch Statement
Now let us understand how the try catch in javascript works. The try-catch statement generally comes in a pair. The try catch in the javascript statement is generally comprised of a try block and a catch block and the last is the final block or both of them.
At first, the try block gets executed which searches for any error in a given block of code and this throws an exception. After that, the catch statement gets executed for any error found in the try block. If there is not any error present in the try block then the catch block gets ignored. And finally, the final block comes. This block gets executed before the ending of the control flow of the program ends. Let us see the syntax of the try catch in javascript.
Description
The try statement is the statement that needs to be executed. The catch statement is that block that gets executed if there is any exception thrown in the try block. There is also an optional identifier in the try catch in the javascript statement. The flow of the try catch in javascript always starts from the try statement. After that, the control goes to the catch block. There may be a catch and final block present together. This will provide us with the three forms of the try statement which are as follows:
- try...catch
- try...finally
- try...catch...finally
There should be always more than one statement in each of the try catch in javascript statements. It should not be a single statement in any of the blocks like other constructs like for, or, etc.
How to Use try...catch Statements?
A try...catch statement in javascript consists of two or three blocks of statements. These blocks can be a try block and a catch block and there may be a final catch block also along with the try and catch blocks. The try block's code is executed first, and the catch block's code is then executed if the try block's code throws an exception. Before the control flow leaves the entire construct, the final frame's function is always run.
Error Object
The error object in javascript is a built-in feature that provides information about the error if there is any error present in the code. There are two properties of this error object that are name and message.
- Name: This property is used to set the error name or to return an error name.
- Message: This property is used to set a message or to return an error message. That message will be a string value.
Optional “Catch” Binding
The optional catch binding is a function in javascript that is used for the error argument. This feature was added in the javascript in ES10/2019. In a try ...catch statement, if there is any error present and we want to skip the manipulation of that error then we use the optional catch binding. The syntax for the optional catch binding is as follows:
The error argument contains the reason and the cause for which the exception occurs in the code. The optional catch binding is used in the situation when we want to fix the error only and we are not interested in knowing the reason behind the error. Let us understand this using a simple example.
Output:
Explanation: In the above example, we have parsed a string abcd. This string is being parsed as a JSON by the use of the JSON.parse method. Still, this will be shown as an error. This is because the given string is not a valid JSON string. After that, the error argument is skipped using the optional catch binding in the catch block of the code.
JavaScript Errors Categorization into Six Groups
Exceptional handling in javascript is a very important feature in javascript that is used to manage all the possible errors that can occur in a code. And the exceptional handling fixes these errors to ensure a smooth flow of the code.
An exception is defined as an object which contains an explanation of what is going wrong in the code. The exception also detects where the error in javascript occurs. The errors in javascript can appear due to mistakes done by any of the developers, wrong inputs, etc.
There are some reasons why an exception occurs in a code which are given below:
- If a number is divided by zero. This division results in an infinity answer and it will throw an exception.
- If the user requests a file and that file is not present in the system.
- If the user provides a wrong input.
- If there is a network drop in between the communication.
Let us now discuss the types of errors in javascript.
EvalError
The EvalError is a type of object error in javascript that represents an error regarding the global eval() function. The global function eval() may throw an instance of the EvalError class if it is called by any other name. The limitations on how this method may be called are described in eval(). For information on throwing and catching exceptions, see Error.
RangeError
The RangeError object in javascript can be seen when the provided value is not in the set of allowed values of that function. In order to inform a user that a value was entered into a function that does not accept an input range including that value, a RangeError is utilized. For instance, all non-zero positive values can be the input range for a function that converts centimeters to inches. In this case, a RangeError would be thrown because the integer -100 is outside the permitted input range.
ReferenceError
The ReferenceError object in javascript represents an error a variable is called and it is not defined early in the code.
syntaxError
A syntax error is a type of error in javascript that occurs when we do not use the pre-defined syntax correctly. Syntax errors are spotted during the compilation of the code or during the parsing of the code. Suppose we are defining a function in javascript and we forget to close the curly braces {} of the function. Here we will get a syntax error.
TypeError
The TypeError object represents an error in javascript if an operation fails to perform in the code. These failures of the operation happen when the value is not of the same type as expected. The TypeError can occur due to the following reasons:
- When we try to modify a value that is static.
- When we try to use a particular value in the wrong way.
URI (Uniform Resource Identifier) Error
The URIError object in javascript represents an error if the URI function is used in the wrong way. A logical (abstract) or physical resource can be identified by a Uniform Resource Identifier (URI), a character string that is typically but not necessarily associated with the internet. One resource is separated from another using a URI.
Parse-time Errors
The syntax errors in javascript are also known as the parse-time error or the parsing error. This error occurs during the interpretation time in javascript which is different in other programming languages. In other languages, this error occurs during the time of compilation.
A parse-time error is a type of error in javascript that occurs when we do not use the pre-defined syntax correctly. Syntax errors are spotted during the compilation of the code or during the parsing of the code. Suppose we are defining a function in javascript and we forget to close the curly braces {} of the function. Here we will get a parse-time error.
The Rethrow Error
As we know the try...catch statement is used to point out any exception in the javascript program. The Rethrow Error exception can be seen in the case when the catch block is unable to rectify the error caught by the try statement. The rethrow exception makes the original thrown object rethrown again. For example
Unconditional Catch-block
In a try catch statement in javascript, if there is a catch block present the catch block gets executed if there is any exception found in the try block. For example, if the exception is present in the given code, the execution gets transferred to the catch block.
The catch block represents a specifier that contains the value of the exception. This value of the exception will be inside the catch block only.
Conditional Catch-blocks
The conditional catch block can be created by adding the try...catch block with the if...else if...else statements. For example:
The Exception Identifier
When there is an exception present in the try block, the exception_var keeps the value of the exception (exceptional value). The user can use this value later in the program to get information about that exception thrown. The exceptional identifier is present in the scope of the catch block. Also, the user can exclude the value of that exception if the user does not require this anymore. The syntax of the exceptional identifier is as follows:
The Finally-block
The finally-block is that block of the code that consists of the statements that need to be executed after the try block and the catch block. But these statements should be executed before the try...catch...finally block.
Flowchart That Illustrates the Flow of The try...catch...finally Statement is as Follows
- Before the try block gets executed, (no exceptions are thrown in the code).
- Just before the statements of the catch block get executed normally.
- Just before the statements of a control-flow statement ( break, continue, return, continue ) get executed present in the try and catch block.
If there is any exception present in the try block, and there is no need to execute the catch block to rectify any error in the try block, the finally-block still gets executed.
The onerror() Method
The onerror is an event handler in javascript. It was one of the first features regarding error handling in javascript. The error event gets triggered on the window object when there is any exception appears on that particular web page. Given below is an example to understand this.
Explanation: In the above example, we created a simple web page. In the script tag, we created an onerror event and assigned a function to it that will create an alert and a message will appear like "An error occurred."
The onerror handler gives the information in three parts that are discussed in the below section.
Error message
This is the piece of message that the browser will display if any error occurs.
URL
This is the file in which the error occurs
Line number
The line number is provided in the URL in which the error occurs.
Global Catch
The global catch error handler gets triggered to enable the library users they can manipulate and handle the exceptions. The thrown exceptions may stop the loop of the program.
Nesting Try Blocks
The nested try blocks are defined as the try blocks inside the try block. But in javascript, other nested statements are easy to understand. But a nested try block in javascript becomes unreadable, so better not to prefer this.
Using the nested try blocks, the user can use only one catch statement for many try statements. But it is not compulsory to do this. The user can also write a catch statement for every try statement. For example:
Examples
Now let us see some examples of the try catch statement in javascript to understand it in a better way.
Example 1. JavaScript try...catch statement to display an undeclared variable.
Output:
Explanation: In the above example, we create two variables namely num and deno. After that, we assigned these variables their value which is 50 to the variable num and x to the variable deno. This variable is not defined. When you try to print the variable, the program throws an error. That error is caught in the catch block.
Example 2. JavaScript try...catch...finally statement
Output:
Explanation: In the above example, we create two variables namely num and deno. After that, we assigned these variables their value which is 50 to the variable num and x to the variable deno. In the above program, an error occurs and that error is caught by the catch block. The final block will execute in any situation. ( if the program runs successfully or if an error occurs). You need to use the catch or finally statement after the try statement. Otherwise, the program will throw an error Uncaught SyntaxError: Missing catch or finally after try.
Conclusion
- The try catch in javascript is used to manage and manipulate that particular section of the code where errors exist. The try-catch in javascript first tests for all the possible errors that may be contained in the code.
- The try{} statement in a try-catch in javascript is used to define a particular block of code that needs to be tested during the execution of the program.
- The catch{} statement is used for defining a block of code that gets executed when there is any error in the code tested by the try{} statement.
- The error object in javascript is a built-in feature that provides information about the error if there is any error present in the code.
- The optional catch binding is a function in javascript that is used for the error argument. This feature was added in the javascript in ES10/2019 which is used if there is any error present and we want to skip the manipulation of that error.
- The JavaScript errors categorization into six groups that are EvalError, RangeError, ReferenceError, SyntaxError, TypeError, and the URI (Uniform Resource Identifier) Error.
- The finally-block is that block of the code that consists of the statements that need to be executed after the try block and the catch block.
- The onerror is an event handler in javascript and it gets triggered on the window object when there is any exception that appears on that particular web page.
- The onerror handler gives the information in three parts that are Error message, URL, and Line number.