JSON Dump in Python
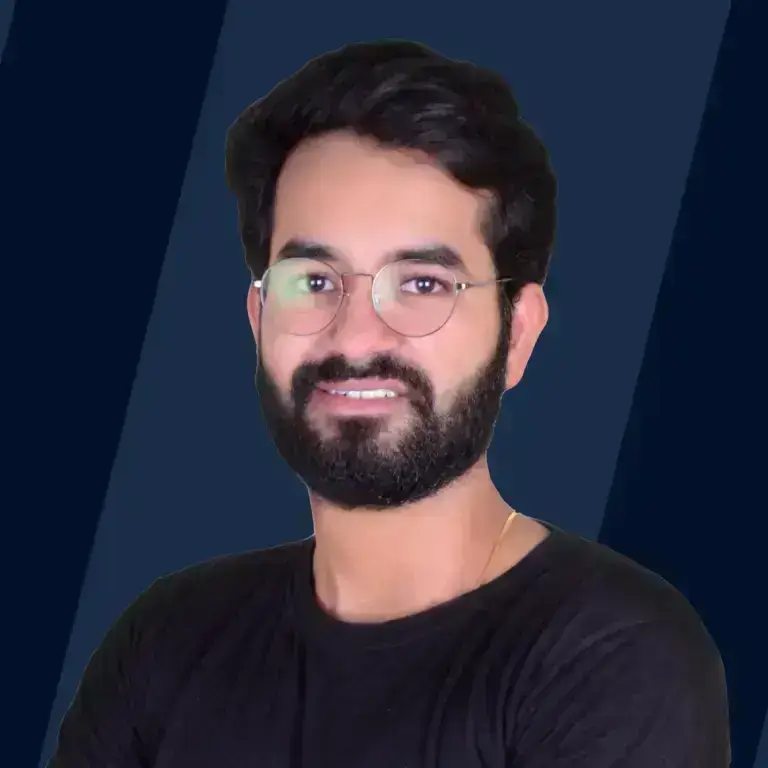
Overview
The dump function in Python is mainly used when we want to store and transfer objects (Python objects) into a file in the form of JSON (JavaScript Object Notation - a standard text-based format or a lightweight format used to store and transport data on the web). The dump function operates quite efficiently. The dump function in Python takes a large variety of arguments. We need to provide the object that needs to be dumped into the file in JSON format as well as the name of the file in which we want to dump our object.
Syntax of json.dump() in Python
The dump function in Python is used to write objects (Python serialized objects) into a file in the form of JSON. The syntax of the json.dump() function is given below.
As we can see that the dump function in Python can take several parameters. Please refer to the next section to know more about the parameters of the dump function in Python.
Note: Serialization is the process of converting an object's state to bits so that it can be stored on a hard drive.
Parameters of json.dump() in Python
The dump function in Python can take a large variety of arguments, let us learn about the various parameters of the dump function in Python in brief.
- object (required): The object parameter resembles the object that needs to be dumped into the file in JSON format.
- output (required): The output parameter resembles the file in which we want to dump our object in the JSON format.
- indent (optional): The indent parameter helps us to improve the readability of the data dumped into the JSON file. We can provide double quotes(" ") as a value to this parameter, we can also provide integer values as the value of the indent parameter. The double quotes(" ") makes the key-value pairs appear in the new line. (Refer to the Example section for more clarity).
- skipkeys (optional): The skipkeys parameter is used to tackle the key error generated during the dumping of data. If the key is not any of the standards-allowed types like int, float, string, None, or bool, the Python interpreter will raise an error so we must set the skipkeys parameter to True.
- separator (optional): The separator takes either one or two values. Now, the first value of the separator parameter tells the Python interpreter about the symbol that will separate one key-value pair from another. On the other hand, the second parameter is used to specify the separator that will separate the key from its value.
- sort_keys (optional): The sort_keys parameter can also take two values (boolean values True or False). So, if the parameter's value is set to True then the keys are set in the ascending order, on the other hand, if the parameter's value is set to False then they are set in the same order as it was in the original Python object.
- ensure_ascii (optional): The ensure_ascii parameter can also take two values (boolean values True or False). The default value of the ensure_ascii parameter is set to True. Now, if the parameter's value is set to False then the non-ASCII characters of the Python object are dumped into the output file as it is in the Python object.
- allow_nan (optional): The allow_nan parameter is used to serialize the range of floating values in the file.
Let us now look at the data types of the parameters:
- skipkeys - bool.
- ensure_ascii - bool.
- sort_keys - bool.
- allow_nan - bool.
- write_mode - int.
- number_mode - int.
- datetime_mode - int.
- uuid_mode - int.
- bytes_mode - int.
- mapping_mode - int.
- chunk_size - int.
- indent - string (double quotes) or integer.
- separatoe - string.
Return Values of json.dump() in Python
As we know that the dump function in Python is used to write objects into a file in the form of JSON. The dump function in Python returns a string object (<class 'str'>) which can be used to print the dumped data.
Exceptions of json.dump() in Python
The dump function in Python does not raise any error or exception if we have it correctly. If the name of the object or the file is wrong then the dump function in Python can raise an error(s). The object that needs to be dumped into the JSON format can be of any type like integer, floating type, string, tuple, list, set, dictionary, etc.
Example of json.dump() in Python
So far we have seen the usage, parameters, and return type of the dump function in Python. Let us now take a simple example to understand the working of the dump function in Python.
Output of the test.json file:
What is json.dump() in Python?
Before learning about the dump function in Python in detail, we should be familiar with the JSON format and the Python built-in module json.
Now, JSON stands for JavaScript Object Notation which is a standard text-based format (a lightweight format) used to store and transport data on the web. The JSON format is similar to the dictionary format in Python. The json module is a built-in module that provides us with various methods and functions that helps us to deal with the JSON data.
The dump function in Python is a method found in the json module. So, for using the dump function in Python, we first need to import the json module as: import json. The dump function in Python is mainly used when we want to store and transfer objects (Python objects) into a file in the form of JSON. The dump function operates quite efficiently.
The dump function in Python takes a large variety of arguments. We need to provide the object that needs to be dumped into the file in JSON format as well as the name of the file in which we want to dump our object. The dump function in Python returns a string object (<class 'str'>) which can be used to print the dumped data.
More Examples
Let us now take various examples to understand the usage and work on the dump function in Python.
Package Called Pickle in Python Which Helps Us in The Serialization of The Python Object
The pickle package in Python is widely used with the json.dump() function to serialize the Python objects. Serialization simply refers to the process of converting a Python object into a stream of bytes so that it can be easily stored on a disk or can be easily sent over a network.
Let us look at the example for more clarity.
Output:
Here is a JSON validator where you can validate your JSON files.
In the output above, we can see that since we have dumped the student object by pickling, the data got converted into bit streams. So, to open the binary file, we have used the wb i.e. write a binary method to open the file.
Example for Writing Data Into a List to Json File Using Dump Function in Python
Let us now try to dump a Python list to json file using the dump function in Python.
Output:
Example for Writing Data Into a Dictionary to Json File Using Dump Function in Python
Let us now try to dump a Python dictionary to json file using the dump function in Python.
Output:
Example for The Allow_Nan Parameter
The allow_nan parameter is used to serialize the range of floating values in the file. Let us take an example to understand the context better.
Output:
Difference between dump() and dumps()
So, far we have seen the working of the dump function in Python. The json module also provides us the dumps() function. The json.dumps() function is used when string format is used.
Let us now learn about some of the differences between the json.dump() function and the json.dumps() function.
dump() | dumps() |
---|---|
The dump function in Python is used when we want to dump the Python objects into a file in JSON format. | The dumps function in Python is used when the objects are in string format and it is mainly used in the case of parsing, and printing. |
The dump function in Python requires the JSON file name in which we want to dump our data. | The dumps function in Python does not require any such file. |
The dump function in Python works faster. | The dumps function in Python works two times slower than the dump function. |
The dump function in Python first writes the Python objects in the memory and then executes a command that writes the data into the file separately. | The dumps function in Python directly writes the data into the JSON file. |
Conclusion
- The dump function in Python is a method found in the json module. The dump function in Python is mainly used when we want to store and transfer objects (Python objects) into a file in the form of JSON.
- The dump function in Python takes a large variety of arguments. We need to provide the object that needs to be dumped into the file in JSON format as well as the name of the file in which we want to dump our object.
- The dump function in Python returns a string object (<class 'str'>) which can be used to print the dumped data.
- The dumps function in Python is used when the objects are in string format and it is mainly used in the case of parsing, and printing.
- The dump function in Python requires the JSON file name in which we want to dump our data, on the other hand, the dumps function in Python does not require any such file.
- The dump function in Python works faster, on the other hand, the dumps function in Python works two times slower than the dump function.
- The dump function in Python first writes the Python objects in the memory and then executes a command that writes the data into the file separately.
- The dumps function in Python directly writes the data into the JSON file.