Lang Package in Java
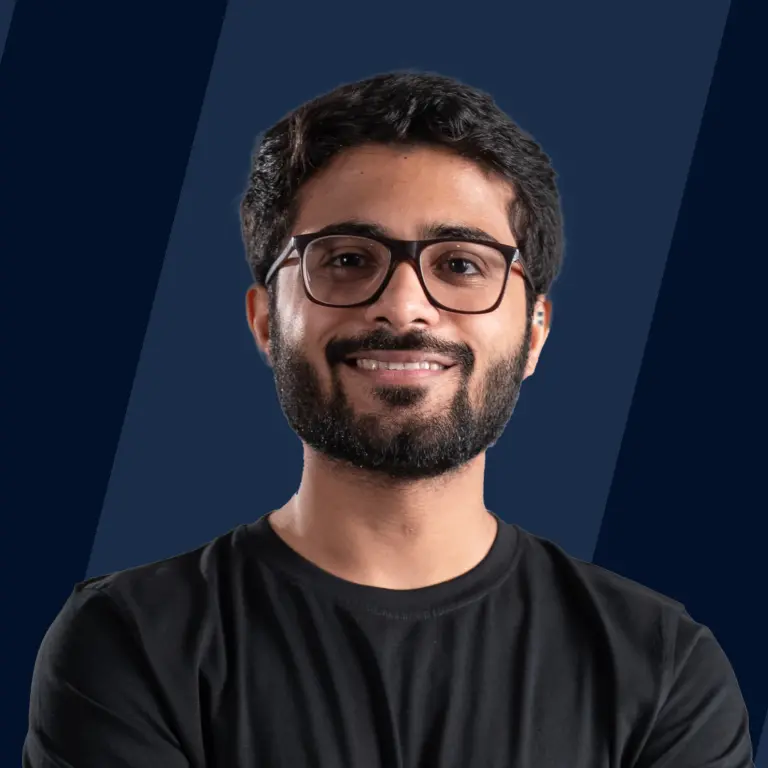
Overview
The lang package in Java provides classes and interfaces that are fundamental to the design of the Java programming language. It is a built-in package provided by the JDK (Java Development Kit). The classes and interfaces of the lang package are found under the path java.lang.
java.lang package is a default package in Java, which means that it is automatically imported into all Java programs. As a result, you can use the classes and interfaces in this package without explicitly importing them.
What is the Lang Package in Java?
Java programming language provides built-in classes and interfaces used to build softwares. For example, java.awt provides APIs to develop Graphical User Interface (GUI), java.io provides APIs to process input and produce output, etc.
java.lang is one of the built-in packages provided by Java that contains classes and interfaces fundamental to the design of the Java programming language. The lang packages contain classes such as Boolean, Integer, Math, etc., that are considered the building blocks of a Java program.
Important Classes of Lang Package in Java
The lang package in Java contains numerous useful classes. In this section, we will look into some of the important classes of the lang package.
Boolean
The Boolean class wraps the value of a boolean primitive type in an object. It contains a single attribute value of type boolean. The signature of the Boolean class is:
Byte
The Byte class wraps a value of primitive type byte in an object. An object of type Byte contains a single field whose type is byte. The signature of the Byte class is:
Character
The Character class wraps a value of the primitive type char in an object. An object of class Character contains a single field whose type is char. The signature of the Character class is:
Class
The instance of the Class class represents the classes and interfaces in a running java application. Class has no public constructor. Instead their objects are constructed automatically by the Java Virtual Machine. The signature of the Class class is:
Double
The Double class wraps a value of the primitive type double in an object. An object of type Double contains a single field whose type is double. The signature of the Double class is:
Exception
The Exception class and its subclasses are a form of Throwable that indicates conditions that a reasonable application might want to catch. The signature of the Exception class is:
Float
The Float class wraps a value of primitive type float in an object. An object of type Float contains a single field whose type is float. The signature of the Float class is:
Integer
The Integer class wraps a value of the primitive type int in an object. An object of type Integer contains a single field whose type is int. The signature of the Integer class is:
Long
The Long class wraps a value of the primitive type long in an object. An object of type Long contains a single field whose type is long. The signature of the Long class is:
Math
The Math class contains methods for performing basic numeric operations such as the elementary exponential, logarithm, square root, and trigonometric functions. The signature of the Math class is:
Object
The Object is the root of the class hierarchy. Every class has Object as a superclass. The signature of the Object class is:
String
The String class represents character strings. The values in the String class are constant and cannot be changed after they are created. The signature of the String class is:
StringBuilder
The StringBuilder class contains a mutable sequence of characters. The StringBuilder class is an alternative to the String class as its values are mutable. The signature of the StringBuilder class is:
System
The System class contains several useful class fields and methods. It contains only a private constructor and cannot be instantiated. The signature of the System class is:
Thread
The Thread class is used to create and run threads in a Java program. It is very useful when a Java program has to be run in a multi-threaded environment. The signature of the thread class is:
Advantages of Lang Package in Java
- The lang package in Java provides primitive values as classes to be used as objects. For example Integer can be used in Generics, but not int.
- The lang package in Java provides the Math class that contains methods to perform various mathematical operations.
- The lang package in Java provides classes to throw different types of exceptions. For example, NullPointerException, NumberFormatException, etc.
Examples of Lang Package in Java
Data Types
In this example, we will look into the usage of data type objects such as Boolean, Byte, Character, Double, Float, Integer, and Long.
Ouput
The code creates objects of different wrapper classes (Boolean, Byte, Character, Double, Float, Integer, Long) by passing string values, and prints the result of each one of them by using the System.out.println() method.
String and StringBuilder
In this example, we will look into the String and the StringBuilder classes.
Output
The above code creates a new String object with the value "ABCD" and assigns it to variable s. Then it creates a new StringBuilder object and assigns it to variable sb. Then it uses the append() method of the StringBuilder class to append the string "A", "B", "C", "D" to the StringBuilder. Finally, the code prints the value of the String object and the StringBuilder object. Note that the toString() method is called on the sb object before printing, since the sb object is not a string but a StringBuilder.
System
In this example, we will look into the System class and its usage.
Output
The code prints "Hello" to the console using the System.out.println() method, then it prints the current time in milliseconds by calling the System.currentTimeMillis() method.
Thread
In this example, we created a thread using the Thread class
Output
The above code defines a Main class that has a main() method which is the entry point of the program. Inside the main() method, an instance of the Task class is created and its start() method is called which starts the thread. The Task class extends the Thread class and overrides the run() method which is where the task's code is executed. In this case, it just prints "Running thread ..." to the console.
Conclusion
- The lang package in Java provides classes and interfaces that are fundamental to the design of the Java programming language.
- lang is a built-in package provided by the JDK itself.
- The classes and interfaces of the lang package are found under the path java.lang.
- The lang package in Java contains numerous useful classes such as Boolean, Integer, String, StringBuilder, etc.
- The lang package in Java provides primitive values as classes to be used as objects, Math class that contains methods to perform various mathematical operations, etc.