List of Dictionaries in Python
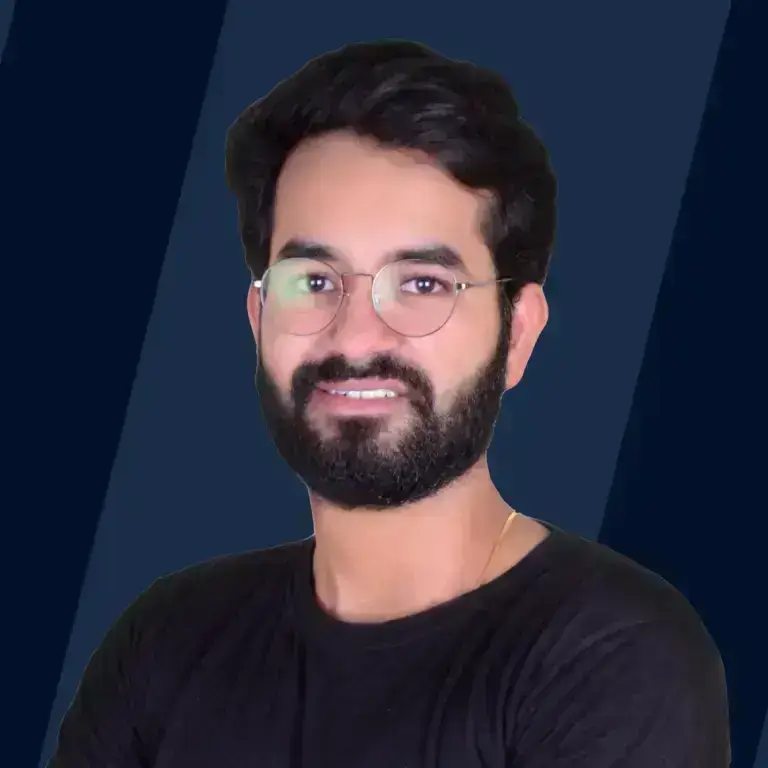
Overview
As we know, a dictionary is a collection of elements that are ordered and can be modified. However, dictionaries in Python do not allow duplicate keys. Dictionaries are used to store data values in "key: value pairs". In this article, we will learn about the list of dictionaries in python. We can create a Python "list" whose each element are Python dictionary. That’s why we call such a type of Python list using a special name – list of dictionaries. Now, let us get started with our article!
Introduction to List of Dictionaries in Python
As we know, a list is a linear data structure in Python that stores a collection of elements in an ordered manner. The values which are stored are the python objects.
Also, a dictionary is a Python object which stores the data in the key: value format.
So, we can create a python list, with its elements as the Python dictionary. Finally, we can call that a List of Dictionaries in Python.
In the above image, we have created a list of dictionaries in Python. {1:'car'} these are dictionaries and they are in the Python list.
How to Create List of Dictionaries in Python
To create a list of dictionaries in Python, the following are the steps involved:
Syntax:
To create a list of dictionaries in Python, we need to:
- Create a Python list.
- Inside the list, put or append the elements which should be python dictionaries.
- Please note, that the keys should be unique.
Code:
Output:
Explanation:
In the above code, we created a list of dictionaries in Python and printed the same. We also verified the type of our list of dictionaries.
Exception:
The dictionaries we pass into our lists must have unique keys otherwise, our value will be overridden by the last key with the same name present. For example:
Code:
Output:
In the above example, 2 keys("name") are having the same name. Hence, the name 'Jack' got overridden by the name 'Jill'. So we must avoid keeping the same keys.
Append a Dictionary to a List of Dictionaries in Python
Just like any other element, dictionaries can also be appended to a list in Python. For that, we use our append() method in Python. It is similar to appending a normal Python list.
Syntax:
To append a dictionary to a list of dictionaries in Python, the parameter we pass to our append method is a dictionary. The append() method adds the passed Python dictionary to the end of the existing Python list of dictionaries.
Code:
Output:
Explanation:
Here, we create a list of dictionaries ls in Python. After that we take another dictionary dict and append it, using "append" in python, to the end of the original dictionary.
Exception:
There is no such exception when it comes to appending a dictionary to a list of dictionaries in Python. We can even append an empty dictionary to our list.
Access the Key Pairs from the List of Dictionaries
We have learnt how can we create a list of dictionaries in Python. Now, let us see how can we access the key: value pair in our dictionary from the list.
Syntax:
To access any key : value pair of a dictionary, we access it using the index of the element in the dictionary. In other words, we use indexing to achieve our result. As we get our dictionary, we can easily access any key: value pair of the dictionary.
In the above image, we are fetching individual key:value pair of the dictionary using list indexes. After that, we are also fetching the dictionary values by using the dictionary keys.
Let us write some code to understand the same.
Code:
Output:
Explanation:
Here we use indexing to get element from our list of dictionaries in python. First we fetch individual elements of the dictionary by ls[0] or ls[1]. After that, we get the value of the dictionary using the dictionary's key, for example ls[1]['fruit'].
Exception:
We should always know the length of our list while accessing the value from our list of dictionaries using index. Otherwise we will face the IndexError: list index out of range. Also, we must provide our key properly while accessing the value otherwise we will get the KeyError:. For example:
Example 1:
Output:
Example 2:
Output:
Update the Key Pairs in the List of Dictionaries
There are several activities which we can perform while we are updating our list of dictionaries in python. Some of them include:
- Adding a new key-value pair
- Updating any existing key value pair
- Deleting any existing key value pair
Add Key : Value Pairs
Let us see the code to add a new key value pair to an existing list of dictionaries in Python:
Syntax:
To add a new key value pair to our list of dictionaries, we can fetch an already existing dictionary's index from our list of dictionaries. Finally, by using a key we can provide our value. Hence, our key-value pairs will be added in our list of dictionaries.
Code:
Output:
Explanation:
In the above example, we added a value to our existing dictionary. We simply find the index of the dictionary in which key value pair is to be added like ls[1]['class'], and using the key class we add a new value.
Exception:
We can only add a new key pair value to an already existing dictionary in our list. We cannot add a completely new dictionary by just using the index, for that we need to use append. For example, if we try to add a key-value pair into the 2nd index, we will get an IndexError, because there is no dictionary at index 2.
Code:
Output:
Update Key : Value Pairs
After this, let us now see how can we update any already existing value in our list of dictionaries.
Syntax:
To update a key-value pair in a dictionary, we can use the already existing index in our dictionary, with the key we want to update. Finally, we will assign that with the value we want to update.
Code:
Output:
Explanation:
In this example, we updated an existing value to a new value in our dictionary. We did so by fetching the element at index 0, followed by the key ls[0]['class'] and assigning it to a new value.
Exception:
- We must be sure we are in the index boundary, while we assign our key with a new value, otherwise we will face IndexError.
- Using the above method, we cannot update the key of our dictionary. Only values of an existing key can be edited or updated.
Conclusion
In this article we read about the list of dictionaries in python. Let us just recap what we learnt throughout:
- List of dictionary is a list consisting of dictionaries as it's elements.
- We can create a list of dictionary by appending the elements of a dictionary into the list.
- We can also access the key value pairs of a dictionary using the index of the list.
- We can also add elements to the list of dictionary by using the index of the list and giving a key.
- We can update and delete the elements of the list of dictionary. Deletion can be performed using "del" keyword in python.