Convert Set into a List in Python
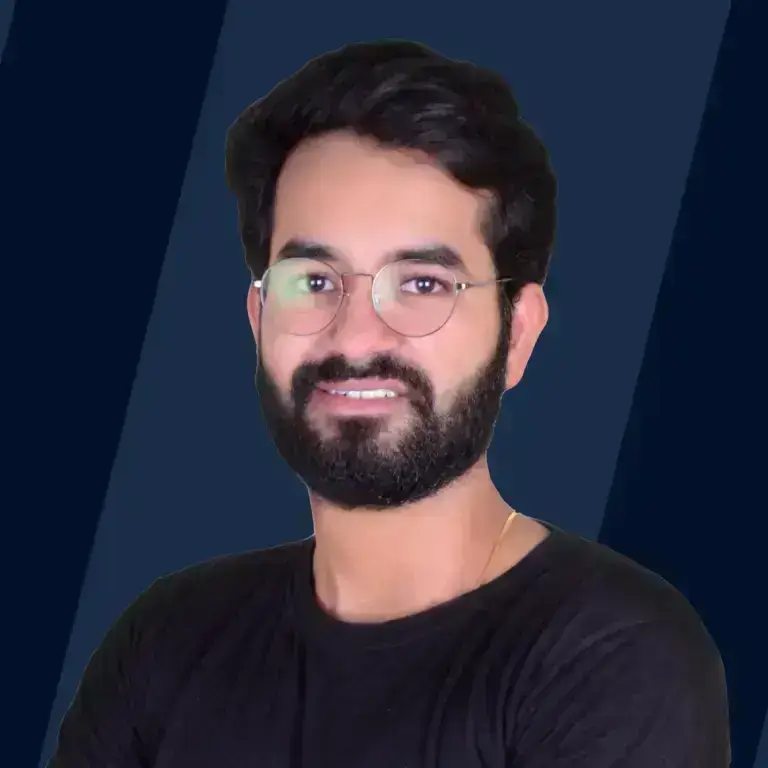
Overview
Type conversion is one of the most important concepts to understand while programming. One can define typecasting as converting the data or variable from one data structure to another for performing necessary operations per the need.
Regarding Python set and list, multiple methods can be used to perform explicit type conversion, that is, in this case, to convert set to list. This article will help you understand all these methods in detail with code snippets for intuitive learning. But before that, this article requires you to have some knowledge of basic Python programming fundamentals as a prerequisite.
How to Convert Sets to Lists in Python?
Before we dive into the core, let's briefly revise the Python sets and lists. Both sets and lists are inbuilt data structures in Python used to store multiple items of different data types in a single variable. Sets and lists are two of the four inbuilt data structures available in Python, with the other two being tuples and dictionaries. Although they are used for the same purpose, different properties and methods allow users to use them for different tasks.
- What are sets ? As the same in mathematics, sets do not allow repeated or duplicate elements in them. Set items have unique properties like unordered and unchangeable and do not allow duplicate or repeated values. Sets are created using curly brackets. For further information on sets, you can refer Sets in Python article. Syntax:
- Creating a set Sets are created in Python in the following way.
Output:
Here a set in the name of items was created, and we can observe it contained non-duplicate elements with different data types. The shuffled order of the output proves that sets are an unordered data structure.
- What are lists ? Lists are created using square brackets, allowing one to store duplicate or repeated items in the same variable. Lists, unlike sets, are an ordered data structure.
Syntax:
Lists are created in Python in the following way.
Output:
Here a list in the name of items was created, and we can observe it contained duplicate elements with different data types.
For further information on lists, you can refer Lists in Python article.
Now that we've seen how to create lists and sets. Let's dive into the article's core, converting sets into lists.
But why do this conversion ? Now let's look at different methods to convert Python sets into lists.
Note: A point to be remembered here is that since lists are ordered, mutable(changeable), and allow multiple instances of the same element, we need not bother about the constraints on sets, except for the order of the elements.
Although the change in typecasting from sets to lists in Python is a visual change in the output. That is, {} to []. But we will still use an additional function to confirm the actual change. Throughout the following code snippets, we will use an inbuilt Python function called type() to verify whether the set was converted to an object in the list class.
Method 1: Using The list() Function
This method will use an inbuilt Python function called list(). It is a simple and commonly used method to convert sets to lists.
Syntax:
Now let's see how to use it.
Output:
Here we created a set called items and later converted it to a list by the same name, items, using the list() function. The two things to observe here are the change from {} to [] while using the print() function and the change in the value returned by the type() function. The type() function returned different values before and after using the list() function.
Method 2: Using the sorted() Method
This method will use an inbuilt Python function called sorted(). Although this function primarily uses to sort elements in a data structure, it can still be used to convert sets into lists in Python. Before dealing with the function itself, one must be aware that this function sorted() can only be used if the elements in the data structures are sortable. If the elements are of numeric type, then the elements will be sorted in either ascending or descending(if the optional argument reverse=True is given) according to the values in the number system. Suppose the elements happen to be categorical or strings. In that case, the elements will be sorted in either ascending or descending order. (if the optional argument reverse=True is given) according to the order of the first character of the element in alphabetical order. The alphabets are compared using the ASCII code of each said alphabet. We can't use this function if each element happens to be another data structure itself, for example, sets of sets or a list of lists.
Now let's see how to use it.
Syntax:
Input: An iterable data structure Output: A sorted list
Output:
Oops, we ran into an error. Could you guess why? Yes, it happened because the elements were not of a sortable type. The function sorted() could not sort an int and a str data type together. Now let's try this with sortable items.
We have successfully converted a set into a list. The changes to observe here are the changes we initially observed in the method as mentioned above and the fact that the list returned by the sorted() function is sorted in ascending order.
Method 3: Manual Iteration
It is the most classical method programmers use to convert sets into lists. This method is not dependent on any built-in functions and is solved using a programmer's greatest tool, logic. This method involves the programmers' logic of iterating over a data structure. Now let's see how we can accomplish it.
Output:
Here we initially created a set called items and an empty list called list_items. Using a for loop and the list append() function, each element of the set was inserted into the empty list. After the completion of the for loop, every element of items was inserted into list_items. Here unlike earlier methods, we could not use the same name for the list and the set because,
The above code would erase the set and create a new empty list in the same name.
While using this method, you need not necessarily use the append() function. You can also make use of indexing to assign elements.
This method is not exclusive to Python but can be used in almost any general-purpose programming language.
Method 4: Using [*set, ]
It is one of the fastest methods to convert a set into a list. But its syntax makes its readability a bit easier.
Syntax:
* means that a variable number of arguments can be passed onto the function. This method unpacks all elements in the set inside a list, hence creating the elements inside the list. So ultimately, a conversion of a set to a list takes place here.
Now let's see how to use it.
Output:
Here using the [] square brackets to create a list and unpacking each set element allowed us to create the elements inside the list. Hence converting the set into a list.
Method 5: Converting a Frozenset to a List
This method involves the conversion of a frozenset into a list. The frozenset is much similar to a set, only that it is immutable (not changeable), hence the term frozen. This means that set functions like add() or remove() are restricted.
Syntax:
Now let's see how to use it.
Output:
Using the list() method, we converted a frozenset into a list.
Method 6: Unpacking the set inside the parenthesis
This method is a bit hard to read but is faster in execution. Syntax:
* means we can pass a variable number of arguments onto the function. This method unpacks all elements in the set inside a list, creating the elements inside the list. So ultimately, a conversion of a set to a list takes place here.
Now let's see how to use it.
Output:
Here using the [] square brackets to create a list and unpacking each set element allowed us to create the elements inside the list. Hence converting the set into a list.
Time complexity
In the world of computer science, the cost of an algorithm or a method or procedure is mainly calculated using two things, time and space. Here we will discuss the time complexity. An algorithm's time complexity is the time it takes to complete its course and generate the output. Of course, this time may vary with the size of input given to the algorithm. The time complexities are represented in three asymptotic notations.
- The Big O (Worst case complexity)
- The Theta (Average case complexity)
- The Omega (Best case complexity)
These three notations are also used to compute space complexities too.
We often use the big O notation for time complexity. Any idea why?
The worst case is the last possible outcome, and it would have already covered the best and average case scenarios.
You can refer to time complexity in detail at other articles, because it is a wide topic and discussing it here would leave the scope of the article. Now that we have a basic understanding of the term time complexity.
Let's look into the time complexity of each of the functions mentioned above that convert set to list in Python.
-
list()
The internal working of the list() function would require it to traverse through the set or given set once. With n being the number of elements or in general terms, the input size, the time complexity of the list() function can be written as, This essentially means that the time required by the algorithm to complete its course would vary with the input size. Larger the value of n, the larger the time required to complete it.
-
sorted()
Internally, this algorithm involves traversing the data structure and comparing values to sort the given array, list, or set. The sorted() function is a mixed algorithm that uses the best merge sort and insertion sort features. Therefore the time complexity of the sorted() function can be written as,
-
Manual Iteration
This method allows one to traverse through the set and use the append() function or indexing to insert elements into the created empty list. The time complexity of the append() function or indexing (in this case) is O(1). This means that it is independent of the input size; no matter how big or small the input size is, the function will always take a constant time. Manual Iteration requires the traversal over the set through a for loop n times, with n being the input size. Therefore the time complexity can be written as,
-
*[set, ] method
This method requires unpacking each element of the set inside the list. Using this principle, the set must go through a traversal or iteration once. Therefore the time complexity can be written as, While this function still gives an O(n) time complexity, the internal working is faster as it is created using native programming languages like C++, which is fast. So this method can help you to complete the conversion from set to list at a slightly faster rate.
Learn More:
Conclusion
Explicit data type conversion or type casting consumes a lot of time if you are unfamiliar with the methods and means to use them. This article suggests some common and popular methods to convert set to list in Python, along with a brief introduction to set and list in Python. It is always recommended to briefly understand the working of each method dealing with these types of conversions to make your code effective and run faster.
Let's go through what we have learned here.
- Set items have some unique properties like unordered, and unchangeable, and do not allow duplicate or repeated values.
- Lists items have some unique properties as well; they are ordered, mutable (changeable), and allow duplicate or repeated values.
- Different methods to convert sets into lists.
- We also discussed the time complexity of each method we used to convert sets into lists in Python.