Round off in Java
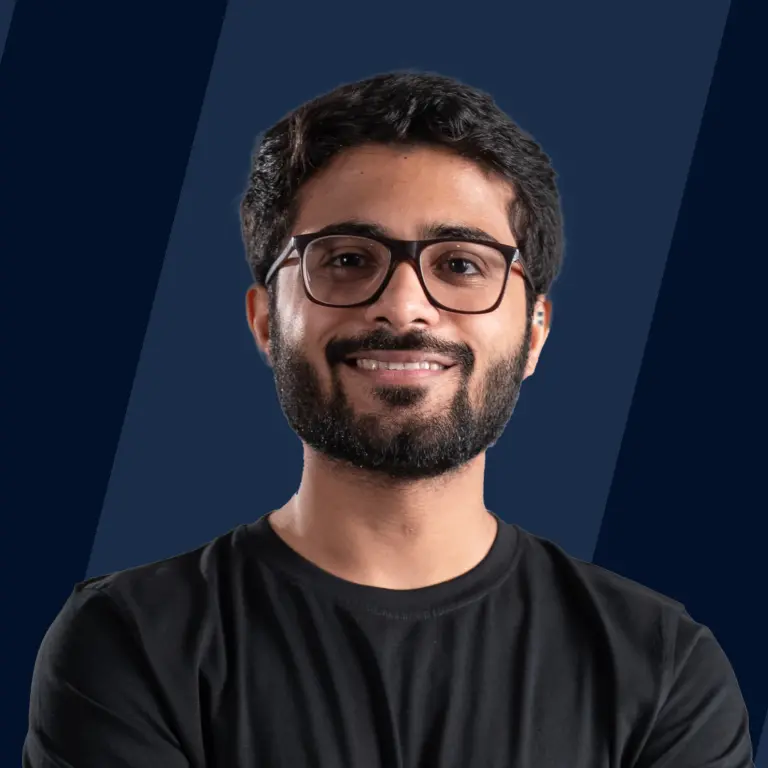
Round off is a term in mathematics that refers to the conversion of a decimal value to the nearest integer value.
Math.round() is one of the many built-in Java methods that allows you to round a floating-point number to the nearest whole number.
- It takes a single argument of type double or float.
- It returns the rounded value as a long or an int, respectively.
- If the fractional part of the number is less than 0.5, it rounds down. If it's 0.5 or greater, it rounds up.
Introduction to Math.round() in java
Round off is used to convert decimal values to integer values for ease of calculations or according to the need of calculation. Values are rounded off to their nearest integer. These values can either be rounded off to a higher integer or a lower integer. If the decimal value is greater than 0.5 then the decimal value will be rounded off to its higher value and if the decimal value is less than 0.5 then it will be rounded off to its lower value.
Lets's see some simple examples to see how we round off normally in mathematics:
=
=
=
Primitive data types are data types that are not considered objects and represent variables. Most of the variables we declare are of primitive data types in java.
There are 8 primitive data types in Java: int, byte, short, long, float, double, boolean, and char. In this article, we will focus on two primitives: float and double.
Both float and double are floating-point types that store fractional numbers, i.e., decimal numbers containing one or more decimals.
1. float
- The float data type can store fractional numbers in range 3.4e-038 to 3.4e+038.
- The precision of float is only six or seven decimal digits.
Example:
2. double
- The double data type can store fractional numbers in the range 1.76-308 to 1.7e+308.
- The precision of double is up to 15 digits. Hence, double is preferred when accuracy is needed.
Example:
Different ways to Round Off in Java
There are different ways provided by Java libraries to round off floating numbers. Some of these methods are part of the java.lang.Math class. However, there are other approaches that can be used for rounding off in Java without using Math class methods.
Java has a predefined class in its java.lang package known as the Math class, which deals with mathematical calculations. The java.lang.Math class extends the java.lang.Object class, which is a superclass in Java. It contains methods for performing basic numeric operations such as logarithm, square root, trigonometric functions, etc.
1. Using Math.ceil() method
The java.lang.Math.ceil() method is used to find the nearest integer value that is greater than or equal to the number given as an argument.
Examples:
ceil(1.2656) = 2
ceil(6876.677) = 6877
ceil(-0.897) = -0
a. Syntax
b. Parameter
The Math.ceil() method takes a double variable as an argument/parameter.
c. Returns
This method returns the nearest floating-point value that is greater than or equal to the number given as an argument to the method.
- If the argument is Not a Number (NaN), this method will return the argument itself.
- If the argument is positive or negative Infinity, this method will return Infinity value with the same sign.
- If the argument is zero, this method will return 0.
- If the argument is a number less than 0 but greater than -1.0, this method will return -0.
- If the argument is a positive or negative double value, this method will return the ceiling value, i.e., the nearest value that is greater than or equal to the argument.
Notes: Even though the Math.ceil() method rounds off the argument, the data type of the output will be a double that's equal to its mathematical integer value. You can remember this by comparing it to the ceiling of your room. A ceiling is at a higher level. The output of the Math.ceil() function will give the upper/greater number as an output.
d. Example
Output:
2. Using Math.floor() method
The java.lang.Math.floor() method finds the nearest integer value that is less than or equal to the number given as an argument.
Examples:
=
=
=
a. Syntax
b. Parameter The Math.floor() method takes double variable as an argument/parameter.
c. Returns This method returns the nearest floating-point value that is less than or equal to the number given as an argument to the method.
- If the argument Not a Number(NaN), this method will return argument itself.
- If the argument is +ve/-ve Infinity, this method will return Infinity with the same sign.
- If the argument is +ve/-ve Zero, this method will return 0 with the same sign.
- If the argument is a number less than 0 but greater than -1.0, this method will return -1.
- If the argument is +ve or -ve double value, this method will return floor value i.e. nearest value that is less than or equal to the argument.
Notes: Even though Math.floor() method rounds off the argument, the datatype of output will be double that's equal to its mathematical integer value. You can remember this by comparing it to the floor of your room. As the floor is downside/at a lower level, the Math.floor() function output will give a lower/smaller number as an output.
d. Example
Output:
3. Using Math.round() method
The java.lang.Math.round() method in Java is used to round off decimal numbers to their nearest integer value. This integer value can be either higher or lower than the rounded-off decimal value. This method takes both float and double variables as arguments.
The result of rounding the argument to an integer is calculated by adding 1/2 (i.e., 0.5) to the number and then taking the floor of the result. This determines the nearest integer value that is closer to the argument.
Let's understand it with some examples:
Example 1: Round off 8.76
- Step 1: Add 0.5 to the floating number.
8.76 + 0.5 = 9.26
- Step 2: Take the floor of the value obtained after adding 1/2.
Math.floor(9.26) = 9
- Step 3: Answer
Math.round(8.76) = 9
Example 2: Round off 1.2
- Step 1: Add 0.5 to the floating number.
1.2 + 0.5 = 1.70
- Step 2: Take the floor of the value obtained after adding 1/2.
Math.floor(1.70) = 1
- Step 3: Answer
Math.round(1.2) = 1
a. Syntax
b. Parameter The Math.round() method takes floating-point numbers, i.e., both float and double data types, as arguments.
c. Returns This method returns the nearest integer value as an output. It is calculated by adding 1/2 to the argument and then taking the floor of the result.
- If the argument is Not a Number (NaN), this method will return zero.
- If the argument is negative infinity or any value less than or equal to the value of Integer.MIN_VALUE, then the function returns Integer.MIN_VALUE.
- If the argument is positive infinity or any value greater than or equal to the value of Integer.MAX_VALUE, then the function returns Integer.MAX_VALUE.
- If the argument is a positive or negative number, this method will return the nearest value. The output can be higher or lower than the argument passed, depending on which value is closer.
Notes: This method uses float and double values as arguments. In the float argument case, the output data type is int. In the case of a double argument, the output data type is long.
Short trick: You can remember this using a short trick. If the decimal value of a positive argument is greater than 0.5, then it will return the nearest number greater than the argument as the output.
Example: 9.8 will be rounded off to 10.
If the decimal value of a positive argument is less than 0.5, then it will return the nearest number smaller than the argument as the output.
Example: 9.2 will be rounded off to 9.
Note: The above trick is for positive numbers; it will be vice versa for negative numbers.
d. Example
Output:
4. Using format
Apart from using the Math class in Java, there are other ways to round a number. One of those methods is the format() method in Java. This method returns the formatted string in the given format and is one of the easiest ways to format output in Java.
To use this method, we can use the System.out.format() statement for output formatting in Java. Similar to the printf statements in C language, we can specify the output format using %c, %f, %d, etc. For example, %f stands for a floating-point number, and we can provide precision for the decimal value using %.4f, which means the output should be a floating-point number rounded to 4 decimal places.
In the Java String format() method, we can specify the expected output format. Now, let's see how we can use this method to round off a floating-point number in Java.
Output:
In the above program, the format() method is used to print the given floating-point number number to 2 decimal places. The output format is specified to the format() method using the format .2f.
In this format, the .2 specifies that we want to display up to 2 decimal places after the dot, and f indicates that we want to print a floating-point number.
5. Using DecimalFormat
DecimalFormat is a subclass of the NumberFormat class in Java. NumberFormat extends the Format class, which is a subclass of the Object class in Java. The provided image shows the hierarchy of the DecimalFormat class.
The java.text.DecimalFormat class is used to format floating-point values according to specific patterns. The format of the expected output is defined using # patterns.
When creating an object of the DecimalFormat class, we specify a pattern using #, representing the expected output format. For example, if the pattern is #.##, it means that the given argument should be rounded off to 2 decimal places. The last decimal place is rounded using the CEILING mode of the RoundingMode class.
Here's an example program that demonstrates the usage of DecimalFormat:
Output:
1087.1235
In the above program, the number num1 is rounded off using the DecimalFormat class. The format of the required output is declared using the # pattern (#.####). This means we want num1 up to 4 decimal places. The rounding mode is set to CEILING, indicating that the last given decimal place should be rounded up.
So, 1087.12345567 rounded to 4 decimal places is 1087.1234, and the last decimal place (4) is rounded up using the CEILING mode, resulting in 5. Hence, the output is 1087.1235.
Note: We need to import the DecimalFormat and RoundingMode classes in Java using the import statement, as they are not part of the default java.lang package.
Conclusion
- Round-off is used to convert decimal values into integers.
- The java.lang.Math class has Math.ceil(), Math.floor(), and Math.round() methods to round off floating-point values.
- Math.ceil() and Math.floor() explicitly convert the decimal value to higher and lower integer values, respectively. They are used in complex calculations where accuracy is required.
- Math.round() converts the decimal value to a higher or lower integer value, whichever is nearest.
- The String format method can be used to round off decimal values up to a desired number of decimal places.
- The java.text.DecimalFormat class, a subclass of java.text.NumberFormat, can be used to format floating-point values into a specific format with the help of RoundingMode.