Float Vs Double in Java - Difference You Should Know
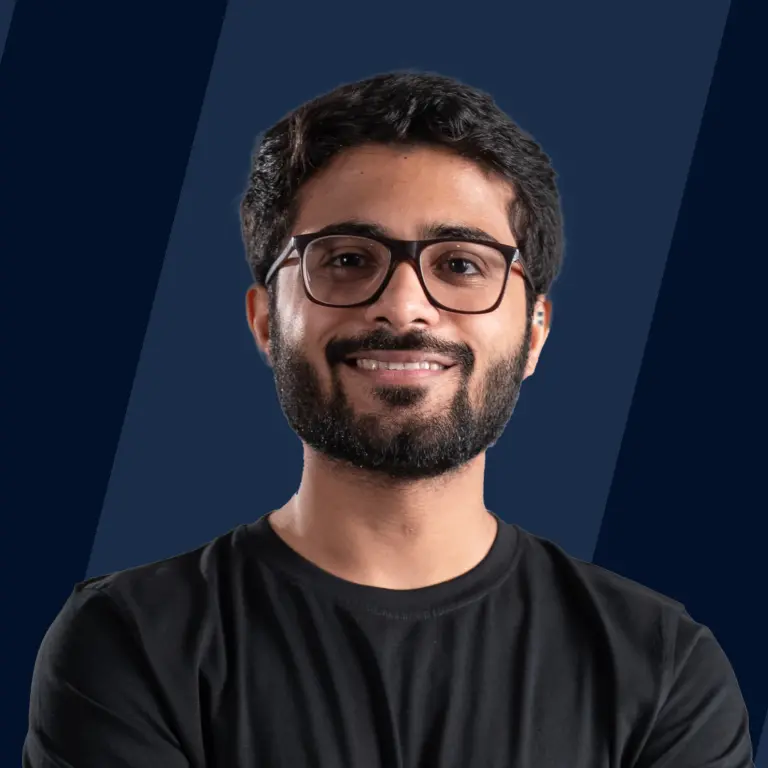
In this article, we are going to learn about the difference between Java Float and Double. So, before getting started with the topic, Let us get a short introduction about the differences between Float and Double in Java.
Float and double are two primitive data types in Java that are used to store floating-point numbers. Floating-point numbers are numbers that can have a decimal point, such as 3.14159 or 1.23456789e10.
Float is a 32-bit floating-point number, which means that it can store up to 7 decimal digits accurately. Double is a 64-bit floating-point number, which means that it can store up to 15 decimal digits accurately.
Float
The float keyword in Java is a primitive data type, and its size limit is 4 byte or 32 bits (1 byte = 8 bits) single-precision IEEE 754 (Standard for Floating-Point Arithmetic) floating-point number, that is it can allow up to 6 digits precision after the decimal. We use this float keyword to declare the variable and methods. It usually stores the value in decimals or fractions. We use this Float data type, if we want to use the memory efficiently, or if the number is in the small range. As the Float consumes less memory in comparison to the Double data type. By default, Java treats any decimal or float numbers as double type, so in case, if we want to define any value as float, we need to manually typecast it using the 'f' or 'F' keyword in the suffix of the number.
For example, if we define a float number as:
In the above code in Java, the declaration of the float variable will throw a compilation error of possible lossy conversion from double to float, but we can fix the error by adding the character 'f' or 'F' in the suffix of the number.
Double
The double keyword in Java is also a primitive data type, and its size limit is 8 byte or 64 bits double-precision IEEE 754 floating-point number, that is it can allow up to 15 digits precision after the decimal. It also stores the value in decimals or fractions. We often use this keyword when we have a larger floating value and if we want a more precise and accurate value. As the Double data type consumes more memory in comparison to the Float data type, but it gives more accurate values of up to 15 digits of precision than the float data type. By default, Java considers any decimal or float values of double type, so we do not need to typecast any decimal value to double manually. Hence, adding the suffix 'd' or 'D' in the number is optional in the case of Double data type values.
For example, we define a double number as:
Key Difference Between Float and Double in Java
The main difference between Java Float and Double is their precision and range.
Float is a 32-bit floating-point number, which means that it can store up to 7 decimal digits accurately while Double is a 64-bit floating-point number, which means that it can store up to 15 decimal digits accurately.
Second major difference lies in their memory usage. Double variables take up twice as much memory as float variables.
Difference Between Float and Double in Java
Now let us look at the Float vs Double comparison table.
BASIS FOR COMPARISON | Float Java | Double Java |
---|---|---|
Definition | The float keyword in Java is a primitive data type, single-precision 32-bit. | The double keyword in Java is a primitive data type, double-precision 64-bit |
Usage | The float data type is less precise, that is, it is used where accuracy is not a big factor, and storage is limited. | The double data type is more precise, that is, it is used where accuracy is a big factor, and we want to avoid data compression or bit loss. |
Storage | The float data type consumes 4 byte or 32 bits for storing a variable | The double data type consumes 8 byte or 64 bits for storing a variable |
Benefits | The float data type have ample support libraries. Open source and community development. | The double data type has more uses for web development, and is more web focused |
Academics | The float data type is a 32-bit IEEE 754 floating point | A double is a 64-bit IEEE 754 floating point. |
Precision | The float data type precision is up to 6 to 7 decimal digits. | The double data type can provide precision up to 15 to 16 decimal digits. |
Range | The float data type range lies between 3.4e-038 to 3.4e+038, and it has a lower range as compared to the double data type. | The double data type range lies between 1.7e-308 to 1.7e+308, and it has a higher range as compared to the float data type. |
Keyword Used | To define a number as float, we mention the float keyword. | To define a number as double, we mention the double keyword. |
Wrapper Class | The float data type wrapper class is java.lang.Float. | The double data type wrapper class is java.lang.Double. |
Default Data Type | Java does not use float as the default data type for floating point numbers. | Java use the double as the default data type for floating point numbers. |
Data Loss | If we convert any float type value from float to double, there will be no data loss. | If we convert any double type value from double to float, there will be data loss. |
Suffix | The float data type uses 'f' or 'F' as a suffix. It is mandatory to mention the suffix if we want the number to be of float type. | The double data type uses 'd' or 'D' as a suffix. It is not mandatory to mention the suffix if we want the number to be of double type. |
Representation | 28.96f or 28.96F | 12.5 or 12.5D or 12.5d |
Similarities Between Java Float and Double
Now that we have seen various differences between Java float and double, there are also many similarities between float and Double. Let us now look at some of the similarities between Java float and double.
- Both the float and double in Java represent or store real numbers in them, that is the numbers with fractions or decimal points. For example :1.23, 3.14, 1.1111.
- Both the float and double contains the approximate values as they are not precise.
When to Use Double and Float in Java?
We can use different data types in different scenarios, depending on the situation. So let us see when to use double and float in java.
When to use Float in Java: We can use the float data type in Java when accuracy is not so relevant to us. Also when the memory is a constraint, we must use float data type, as it consumes half of the memory double consumes. So, if we want to consume memory efficiently and if the data is in a smaller range or 16 precision decimal digits are not required, the float will be the best and optimal data type to use.
When to use Double in Java: As double is more precise than float. We can use the double data type in Java when the memory is not a big concern, and if we want more accurate and precise results. If the value is not in the range offered by float, that is, if the digits after the decimal point of the value are more than the float precision, we must use double data type, in that case, to get the result more accurate. Another reason for using double over float is if there is no memory and space constraint, and if the data is in larger range and also if we want our data to be more accurate, that is, if 16 precision decimal digits are required.
Let us look at an example to see how the double data type consumes more memory but gives more accurate results than the float data type.
Example:
The following two Java programs clearly show the differences between float and double data types.
Float data type code example:
Output:
Double data type code example:
Output:
Explanation:
Let's understand the above codes :
- In the first code we have stored the value in float, and in the second code, we have stored the value in double.
- We can observe in the above codes, how when we are printing the result of double type, it is giving a more accurate value than the float type.
- Hence, from the above code, it is clear that the double data type consumes more memory than the float data type, also it gives more accurate values up to 16 decimal digits, whereas the float data type gives less accurate values up to 6 decimal digits.
- The double data type is storing the double-precision number whereas the float data type stores the single-precision number.
Learn More
I suggest you go through the below tags to enhance your learning of the Java topics.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
We learned about the differences between the float data type and double data type in Java in this article. Let us now recap the points we discussed throughout the article:
- The best way to choose between float and double is to consider the specific needs of your application regarding precision, range, and memory usage.
- If you need to store floating-point numbers with a high degree of precision or if you are working with large numbers, then you should use double. Otherwise, you can use float.
- On the other hand, if memory efficiency is a primary concern, and you can tolerate lower precision, float may be suitable.
- Use float for storing coordinates, time, and other values that do not require high precision.
- Use double for storing financial data, scientific calculations, and other values that require high precision.
- If you are unsure which one to use, it is generally safe to use double.