Python Program to Multiply Two Matrices
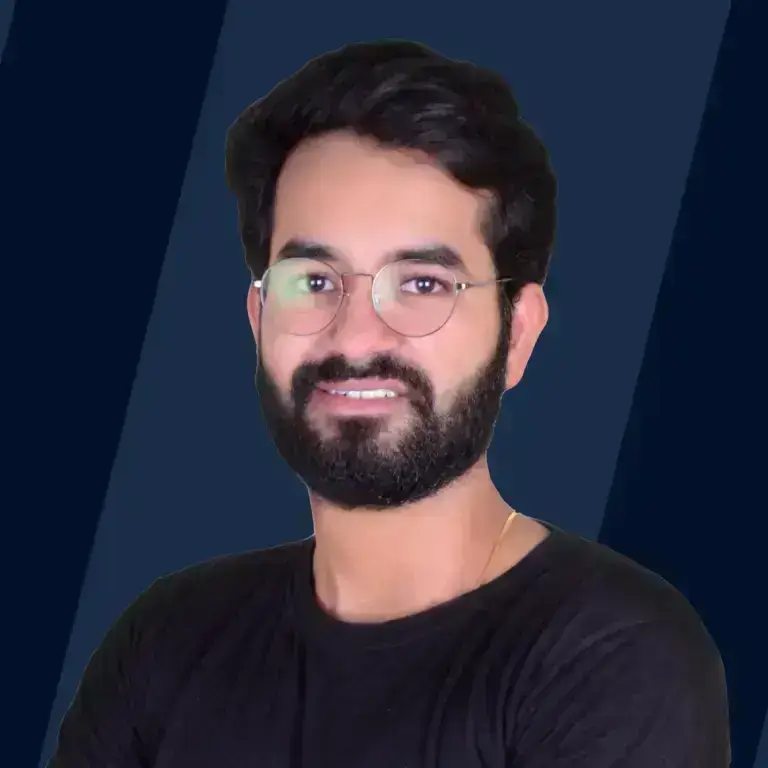
In this blog post, we are going to learn about matrix multiplication and the various possible ways to perform matrix multiplication in Python.
Firstly, understanding loops, lists and other mathematical theories is essential to grasp matrix multiplication. Then, we will look at how to perform the same in Python, with and without using built-in or library functions. The version of Python used for code implementation in this article is Python 3.
The following example will help you understand the Matrix:
Method1: Matrix Multiplication in Python Using Nested Loops
Output
Explanation
- This method encodes two matrices, A and B, as nested lists in Python and retrieves their dimensions.
- It then checks for compatibility and initializes a product matrix C with zeros.
- Matrix multiplication is performed using nested loops, computing each element of C as the sum of products of corresponding elements from A and B.
- Finally, the resulting product matrix C is printed to the console.
Time complexity:
Space complexity:
Method 2: Matrix Multiplication in python Using Nested List Comprehension
Let's learn the Matrix Multiplication in Python using nested list comprehension.
Explanation:
- This implementation uses nested list comprehension to perform matrix multiplication concisely.
- The outer list comprehension iterates over rows of matrix A.
- The inner list comprehension iterates over columns of matrix B.
- Each element in the resulting matrix computes the sum of products of corresponding elements from rows of A and columns of B.
- The result is stored in a new matrix representing the product of matrices A and B.
Output:
Time complexity:
Space complexity:
Method 3: Matrix Multiplication in Python (Vectorized implementation)
- This Python code defines a function matrix_multiply that uses NumPy's np.dot() function to perform matrix multiplication.
- It inputs two matrices, A and B, and returns their product.
- The np. dot() function efficiently computes the dot product of two arrays, corresponding to matrix multiplication for 2D arrays.
- The result is printed to the console.
Output:
Time complexity:
Space complexity:
Method 4: Using recursive matrix multiplication
Program for matrix multiplication in Python using Recursive matrix multiplication:
Output:
Time complexity:
Space complexity :
Conclusion
- Matrix multiplication is a binary operation on two matrices, which yields yet another matrix, which is referred to as the product matrix.
- The blog post covers four ways to multiply matrices in Python: nested loops, list comprehension, NumPy's vectorized functions, and a recursive approach.
- Each method is explained with simple examples and clear explanations, making it easy to understand how they work and when to use them.
- We have also learned a program for matrix multiplication in Python using different methods.