Menu Driven Program in Python
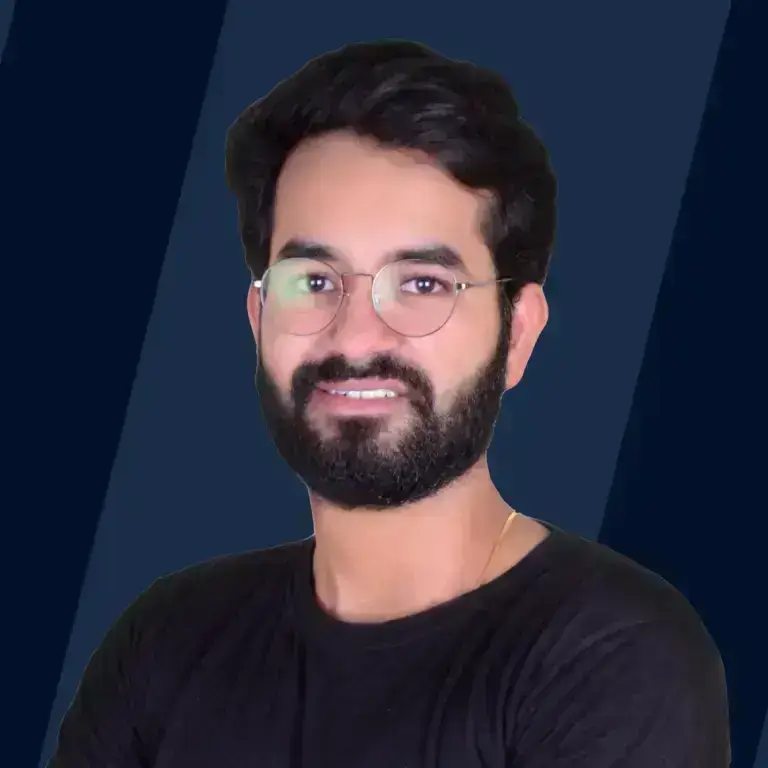
Overview
Menu-driven programs are interactive programs that take input from the users and perform actions according to their choice. While making the applications easy to use menu driven programs also make them unambiguous by giving fixed choices to the user.
With this approach, the user can select an operation from a menu and provide input as needed. The program acts according to the input taken from the user. All the data required for completing the tasks are taken from the user. Implementation of some menu-driven programs is available in this article.
Introduction To Menu-Driven Program
A menu-driven program is a programming approach that displays the list of operations the code can perform and asks the user to choose one of them.
User can interact with the Operating System while execution of code through GUI(graphical user interface) or CLI(Command Line Interface).
The image above displays the output of a menu-driven program designed for a shopping store. The program handles product-related information for that store. An interactive command-line interface shows a list of operations that the program can be used for and prompts users to choose an input, and further execution will be based on the input. We will be creating a program similar to the one displayed in the above image in this article.
We can see many applications of the Menu-driven programming approach in our day-to-day life. Microprocessor-controlled appliances like washing machines, microwaves, etc. have systems that follow the same approach. ATMs (Automated Teller Machines) and vending machines also use a menu-driven programming approach. Many software systems also follow the same approach for implementing their functionalities. All these systems take input from users from the single keystrokes and perform actions accordingly.
Let's take the ATM case, the user gets a list of options that he can perform. He chooses one of them like balance inquiry or cash withdrawal and with some keystrokes, he receives the cash.
The menu-driven system makes it much more interactive and the limited options provided make them precise. The input is taken from users in a single keystroke reducing the chances of errors.
In this article, we will see some examples of how we can implement menu-driven programs.
Advantages Of Menu-Driven Program
- Menu-driven programs have a simple, user-friendly, and interactive interface.
- These are self-explanatory and users can easily operate the systems.
- Menu-driven systems are less prone to errors as they have limited options and users give input in single keystrokes.
- The programming approach is best for beginners.
Perimeter And Area Calculation Of Geometric Shapes
Let's code a menu-driven program for calculating the area and perimeter of different geometric shapes like circles, triangles, rectangles, and squares. Here, we will get a menu on the command prompt which will display the shapes and the calculations users will be able to perform using the code. The program will ask users for their choices, and required inputs and print the output accordingly.
Here, for displaying the menu continuously and making users interact with the system we will use the while loop in python and for switching into the loop according to the user input nested if-elif-else loops.
-
In order to understand how the while loop works in Python, read the following article - Click Here.
-
In the following article, you'll learn more about the if-elif-else loop in Python - Click Here.
Now, let's see a simple mensuration program that calculates the area and perimeter of different geometric shapes.
Program :
Output :
Here, I have calculated the perimeter and area of a rectangle using the above code. You can choose any other option available from the menu.
In the above code, methods are defined that take required arguments and calculate the perimeter and area of the specified shapes. For eg, the method per_circle takes the radius of the circle as an argument and calculates the perimeter of the circle using a mathematical formula.
Similar to per_circle several methods are defined that return perimeters and areas of different shapes.
while True loop is used for infinite iterations, it displays the menu repeatedly and the users can choose the operations they can perform. The while loop displays the menu of different shapes and takes input from the user in variable shape_choice.
According to the input from the user in the shape_choice variable, the code executes relevant block form if-elif-else conditional blocks.
When execution enters in any one of the if_elif conditions one more menu is displayed where the user is asked to choose if it wants to calculate the area or perimeter of the chosen shape.
After getting input from the user in the variable choice1 code takes the required arguments for calculation from the user. The appropriate method is called and the result is given to the user.
This is an endless loop, if the user wants to stop execution and get out of the loop it has to give the input of the Exit option in the menu.
The exit condition is handled in the else loop where the break statement is used to terminate the execution of the loop.
Menu-Driven Program To Create A Simple Calculator
Let's see one more simple example of a menu-driven program that we use in our daily life. The calculator takes different inputs from a user and performs arithmetic operations.
In this code of Calculator, we will use menu driven programming approach that displays the operations the code can perform. Choose one of these operations, give the inputs and get results accordingly.
Program :
Output :
In the above code, we have displayed a menu of the operations that the calculator can perform. Methods are defined that performing the calculations and displaying results.
while True loop is used for infinite iteration and if-elif-else loop is used for decision making to execute according to the choice made by the user. break statement is used to terminate the execution of the while loop in the final else condition.
Check out this article on Calculator Program in Python to learn more about building a calculator from scratch!
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.
Conclusion
- Menu Driven Programs are used to make the program interactive and take inputs from users.
- while True and if-elif-else loops are used for iteration and decision making respectively.
- The menu displayed on the command prompt makes the user aware of the operations program can perform.
- Taking inputs from the user in a single keystroke makes the menu-driven programming approach easy and precise.