Modulus in Python
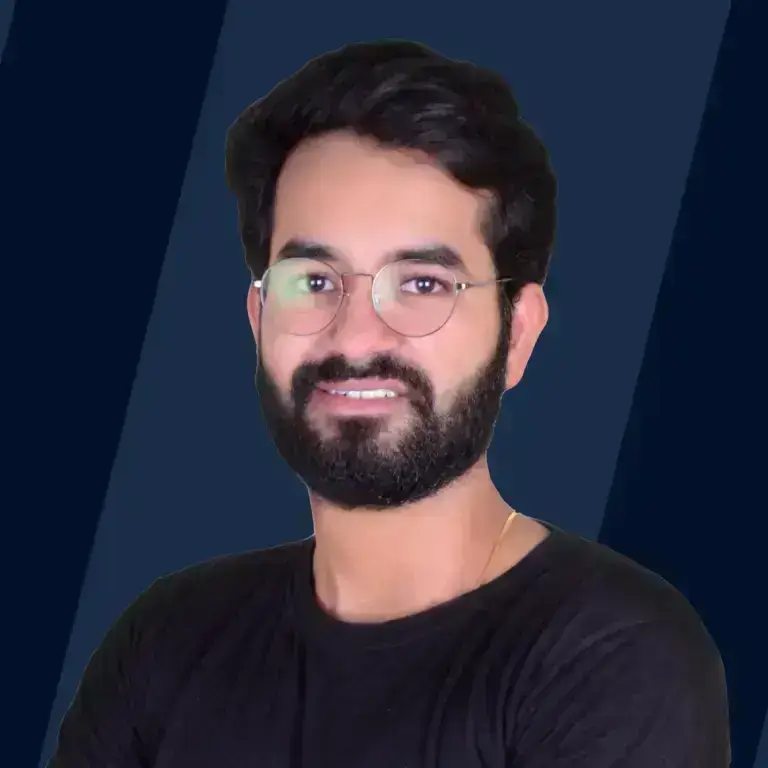
Overview
Modulus is an arithmetic operation that gives the remainder when the first number is divided from the second number. Modulus in Python is achieved by using the modulo operator. Modulo operator in python is represented by the percentage symbol.
The Python Modulo Operator
You must have seen the '%' symbol in mathematics. It denotes the percentage sign. In programming languages, we define it as a modulo operator.
Modulus in python is used as an arithmetic operator when you want to divide two numbers and want the remainder as the output.
Suppose you've two numbers 10 and 3 and you need to find the remainder when 10 is divided by 3. Here comes the function of the modulo operator in python where you can do 10%3 and get the result as 1.
Let's look at few basic examples:
Modulo Operator with Integer
The modulo operator in python, when used with two positive integers, will return the remainder of standard Euclidean division as:
Example:
Explanation:
- In the first case, when 15 is divided by 4 it leaves the remainder as 1. Hence, its output is 1.
- In the second case, 100 is exactly divisible by 2 and hence the output is 0.
Modulo Operator With float with a negative number
You've seen how the modulo operator in python works with positive operands. But things are different when the operands are negative.
Different languages like Python and other handles the negative operands in different ways!
In Python, the output will have the same sign as the denominator. If the denominator has a positive sign then the output is positive and when the denominator is negative, the output is also negative.
Example:
Explanation:
- In the first case, the divisor is negative and thus the output will be negative.
- While that of the second case where the dividend is negative and the divisor is positive, in simple words, with positive dividend, modular occurs like normal operation.
Get the modulus in of two integers numbers using while loop
To find the modulus in python of two integers using a while loop in Python, you can repeatedly subtract one number from another until the remainder is less than the divisor. Here's an example:
Get the modulus of two float numbers
To find the modulus in python of two float numbers in Python, you can use the modulo operator (%) in python just like with integers. Here's an example:
In this example, float_result will be the result of the modulus operation on the two float numbers 10.5 and 3.2. The result will be a float value.
Get the modulus of two numbers using fmod() function
Python has a built-in function called fmod() specifically for float operands.
It is part of the standard library under the math module.
Syntax
Parameters
It takes two parameters that represent the two operands of float data type.
Output:
Example:
Explanation:
- You've to import the math library for using the fmod() function.
- It takes two floating-point values as its arguments.
- In this case, 17.2 is divided by 1.1 leaving the remainder as 0.699999999999998.
Get the modulus of n numbers using function
If you want to calculate the modulus in python of a list of numbers using a function in Python, you can create a function that takes a list of numbers and a divisor as parameters. The function can then iterate through the list, calculating the modulus for each element and storing the results in a new list. Here's an example:
In this example, the calculate_modulus function takes a list of numbers (numbers) and a divisor as parameters. It uses a list comprehension to iterate through each number in the list, calculates the modulus in python with the given divisor, and stores the results in a new list (modulus_results).
Get the modulus of given array using divmod() function
Python has a built-in function that exactly does the same work as the modulo operator. It is a part of python's standard library and can perform mathematical operations.
If integers are passed onto the function, then the Python compiler returns output in integers.
If floating numbers are passed onto the function, the output is also returned in floating data type.
If negative values are passed the remainder will take up the sign of the second operand same as the denominator.
Syntax
Parameters
It takes two parameters that represent the two operands.
Output
A tuple is an ordered sequence of elements enclosed in brackets.
Example:
Explanation:
- In the first case, when 15 is divided by 4, it gives the quotient as 3 and remainder also like 3. Hence, its output is a tuple with (3, 3).
- In the second case, 9.6 is divided by 2 leaving 1.5999999999999996 as the remainder.
Example using the Modulo Operator
Examples:
Output:
Example 1 demonstrates the use of the modulo operator with integers. The result is the remainder when a is divided by b.
Example 2 shows the use of the modulo operator with floating-point numbers. The result is the remainder when x is divided by y.
Example 3 demonstrates the modulo operator with negative numbers. The result is the remainder when c is divided by d.
ZeroDivisionError in Python
In Python, the compiler throws an exception whenever a number is divided by zero known as ZeroDivisionError. Therefore, in order to avoid the error, you shouldn't use zero in the denominator.
Let's see an example to see how Python exceptions in modulus operator work:
Output:
Explanation:
In the above example, try and catch block is used if in case the compiler throws an exception.
So, we try to divide a number by zero and see what the output looks like.
As the output can be seen, the Python compiler throws an exception stating that you cannot divide a number by zero when the modulo operator is used.
Conclusion
- In this article, we came across various methods of using Modulus in Python.
- In fact, Python has built-in functions that work similar to the modulo operator. It is mainly used for time and pattern purposes.
- The modulo operator in python is used to find the remainder of a division operation. It returns the value that is left over after dividing one number by another.
- The modulo operator in python is represented by the percent sign (%) symbol. It is applied to two operands, typically integers or floating-point numbers.
- The sign of the result is determined by the sign of the divisor. If the divisor is positive, the result is positive; if the divisor is negative, the result is negative.
- The modulo operator in python is a convenient and efficient way to calculate remainders compared to manual methods.