Python math Module
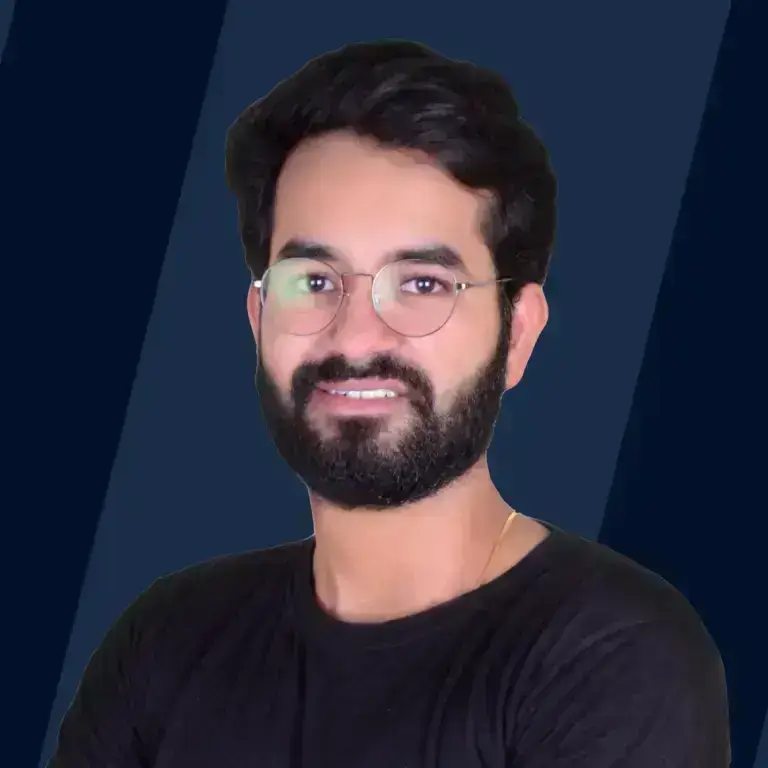
The math module in Python equips programmers with essential tools for various mathematical operations, ranging from basic arithmetic to advanced functions like trigonometry and logarithms. This module simplifies tasks like calculating the sine of an angle, making complex mathematical problem-solving more efficient and accessible in Python.
What is a Math Module in Python?
- The math module in python is a built-in library specifically designed for mathematical operations, offering a wide range of advanced functions and constants.
- It also includes important mathematical constants like π (pi) and e (Euler’s number).
- To access these advanced mathematical capabilities in your Python programs, you can import the math module as follows:
Let us discuss the various numeric functions & constants available in the math library in Python language:
Constants Provided by math Module in Python
Several mathematical constants are required occasionally to perform certain mathematical calculations. For example, you must have used a constant called π (pie) for calculating the area of a circle of radius ‘r’. A variety of constants are available in Python’s math module, which is as follows:
1. Euler’s Number
- It represents the mathematical constant e = 2.718281….
- It is often used in exponential functions and logarithms.
Syntax:
Example: For calculating the value of :
Output:
2. Pi
- It represents the mathematical constant
Syntax:
Example: It can be used for the calculation of the area of a circle with radius ‘radius’ as follows:
Output:
3. Tau
- It represents the mathematical constant
Syntax:
Example: For calculating the value of :
Output:
4. Infinity
- It represents the value of floating-point infinity, i.e. a huge number.
Syntax:
Example: See its value :
Output:
5. Not a Number (NaN)
- It represents the floating-point NaN value, not a Number.
Syntax:
Example: See its value:
Output:
List of Mathematical Functions in Python
Function Name | Description |
---|---|
ceil(x) | Yields the smallest whole number that is greater than or equal to 'x' |
copysign(x, y) | Generates a numerical value possessing the sign of 'y' and the magnitude of 'x'. |
fabs(x) | Delivers the non-negative value of 'x' |
factorial(x) | Calculates the product of all positive integers up to 'x' |
floor(x) | Gives the largest whole number less than or equal to 'x' |
gcd(x, y) | Determines the largest positive integer that divides both 'x' and 'y' without a remainder |
fmod(x, y) | Provides the remainder of 'x' divided by 'y' |
frexp(x) | Decomposes 'x' into its binary significand and an exponent for 2 |
fsum(iterable) | Calculates the sum of a sequence of floating-point numbers with precision |
isfinite(x) | Tests if 'x' is a finite value, neither infinite nor NaN |
isinf(x) | Verifies if 'x' represents an infinite value |
isnan(x) | Checks if 'x' is not a number |
ldexp(x, i) | Computes x multiplied by 2 raised to the power of 'i' |
modf(x) | Splits 'x' into its fractional and integer parts |
trunc(x) | Trims 'x' to its whole number component |
exp(x) | Computes the exponential of 'x', or e to the power of 'x' |
expm1(x) | Calculates e to the power of 'x', minus one |
log(x[, b]) | Determines the logarithm of 'x' with base 'b', defaulting to the natural logarithm if 'b' is not provided |
log1p(x) | Computes the natural logarithm of (1 plus 'x') |
log2(x) | Evaluates the base-2 logarithm of 'x' |
log10(x) | Evaluates the base-10 logarithm of 'x' |
pow(x, y) | Raises 'x' to the exponent 'y' |
sqrt(x) | Extracts the square root of 'x' |
acos(x) | Evaluates the arc cosine of 'x', in radians |
asin(x) | Evaluates the arc sine of 'x', in radians |
atan(x) | Determines the arc tangent of 'x', in radians |
atan2(y, x) | Evaluates the arc tangent of the quotient of 'y' by 'x' |
cos(x) | Calculates the cosine of 'x', with 'x' in radians |
hypot(x, y) | Finds the hypotenuse of a right-angled triangle with sides 'x' and 'y' |
sin(x) | Computes the sine of 'x', with 'x' in radians |
tan(x) | Determines the tangent of 'x', with 'x' in radians |
degrees(x) | Converts the angle 'x' from radians to degrees |
radians(x) | Converts the angle 'x' from degrees to radians |
acosh(x) | Calculates the inverse hyperbolic cosine of 'x' |
asinh(x) | Evaluates the inverse hyperbolic sine of 'x' |
atanh(x) | Determines the inverse hyperbolic tangent of 'x' |
cosh(x) | Evaluates the hyperbolic cosine of 'x' |
sinh(x) | Evaluates the hyperbolic sine of 'x' |
tanh(x) | Determines the hyperbolic tangent of 'x' |
erf(x) | Evaluates the error function of 'x' |
erfc(x) | Evaluates the complementary error function of 'x' |
gamma(x) | Computes the gamma function at 'x' |
lgamma(x) | Computes the natural logarithm of the gamma function at 'x' |
Numeric Functions
The numeric functions in the math module take real numbers/integers as arguments, and after the specified mathematical calculations, it returns a number or a boolean value.
1. Finding the ceiling and the floor value
math.ceil(x)
- Input: Real Number
- Return Type : Integer
This function accepts a single numeric argument and yields the least integer that is not less than .
Example:
Output:
Output is 3 as 3 is the smallest integer, which satisfies the expression
math.floor(x)
- Input : Real Number
- Return Type : Integer
The function takes a single number as an input and provides the largest integer that is less than or equal to the given value, x.
Example 1:
Output:
Output is 2 as 2 is the largest integer such that 2<=2.6
Example 2:
Output:
Output is -3 as -3 is the largest integer such that
2. Finding the factorial of the number
- Input: One positive integer
- Return Type: Positive integer
This function takes only positive integral values, i.e. x>=0 as an argument. If we give any argument other than that, it would show us an error message. This function returns the factorial of the number x. Like, factorial(5)=5x4x3x2x1=120. In real life, the factorial of a number ‘n’ means the number of ways of arranging ‘n’ objects in a row. For example, if there are 3 books on a shelf namely: A, B, C, then they can be arranged in factorial(3) ways = 3x2x1 = 6 ways as shown below:
A,B,C
A,C,B
B,A,C
B,C,A
C,A,B
C,B,A
Example:
Output:
Factorial of a positive integer can also be found using a simple for loop in the following manner :
The time taken for calculating the factorial of 100000 by both approaches is shown below:
By For-loop:
By math.factorial() function:
It can be seen that the math.factorial() function is faster in terms of speed of execution than a simple for-loop approach. So, that’s why using the math module math.factorial() function is better.
3. Finding the GCD
- Input: Integer numbers
- Return Type: Integer number The function accepts two integers as input and outputs the greatest common divisor (gcd) of x and y. For example, as 5 is the greatest integer that divides 5 and 125 together. Also, as 9 is the largest integer that divides both 27 & 81.
Example:
Output:
4. Finding the absolute value
- Input : Real Number
- Return Type : Floating-point number
This function returns the absolute value of the number given as an argument. By absolute, we mean that if the number is -3, it would become positive, i.e. 3.
The number on the left side of the origin on the x-axis becomes positive, i.e. it gets multiplied by -1 when returned. For example, .
Example:
Output:
Output:
There is another inbuilt function in Python known as abs(). This function works in the same way as the math.fabs() function works with the difference that abs() would return the data type of the number based on the input number given to it, i.e. it would return an integer data type number if the input argument is an integer else it would return a floating-point number if the input argument is a floating-point number.
Example:
-
abs(-1) = 1 # here -1 is an integer, so an integer 1 is returned
-
math.fabs(-1) = 1.0 # here, irrespective of -1 being an integer floating point 1.0 is returned.
-
abs(-1.6) = 1.6 # here floating point 1.6 is returned
Logarithmic and Power Functions
1. Finding the power of exp
- Input: A real number
- Return type: A floating-point number
This function takes one number x as input and returns the value of ex, where Which is known as the base of natural logarithms or the Euler’s number.
This function is generally more accurate than simply calculating e**x.
Example:
Output:
2. Finding the power of a number
- Input: Two real numbers
- Return type: A floating-point number
The function wants two numbers as parameters and yields the result of multiplying x and y. For example : math.pow(3, 4) = 81.0 as 34 = 81.0
Example:
Output:
Note: This expression could also be evaluated using the KaTeX parse error: Expected 'EOF', got '‘' at position 1: ‘̲**’ operator i.e. x**y. But, the math.pow() function is better in terms of efficiency if you calculate the execution time for both approaches and compare them.
Also, read pow() function
3. Finding the Logarithm
- Input: A positive real number, one optional argument with the default value of ‘e’, i.e. the Euler's number
- Return Type: A floating-point number
This function can take one or two arguments. If only one argument is provided, it returns the logarithm of the number x to the base e, i.e. .
When provided with two arguments, the function yields the logarithm of 'x' to the base 'b'.
Example:
Output:
Example:
Output:
4. Finding the Square root
- Input: A real number
- Return Type: A floating-point number
This function takes one number as an argument and returns the square root of x, i.e. √x. For example,
Example:
Output:
learn more about the sqrt() function in Python.
Trigonometric and Angular Functions
1. Finding sine, cosine, and tangent
Sine Function
- Input: A real number
- Return Type: A floating-point number
This function takes one number x in radians as input and returns the sine of x.
Example:
Output:
Cosine Function
- Input: A real number
- Return Type: A floating-point number
This function takes one number x in radians as input and returns the cosine of x.
Example:
Output:
Tangent Function
- Input: A real number
- Return Type: A floating-point number
This function takes one number x in radians as input and returns the tangent of x. Example:
Output:
2. Converting values from degrees to radians and vice versa
Conversion to degrees
- Input: A real number
- Return Type: A floating-point number
This function takes one number, x in radians, as input and returns the converted value of x in degrees. For example, . Here math.pi = π & the value value of math.pi in radians = 180.0
Example:
Output:
Conversion to radians
- Input: A real number
- Return Type: A floating-point number
This function takes one number x in degrees as input and returns the converted value of x in radians. Like degrees equal 3.14.. In radians.
Example:
Output:
Special Functions
1. Finding gamma value
- Input: A real number
- Return Type: A floating-point number
This function takes one number, x, as an argument and returns the value of the gamma function of x.
Example:
Output:
2. Check if the value is infinity or NaN
Checking for infinity
- Input: A real number
- Return Type: Boolean value
The function accepts a number, 'x', as an argument and returns true if 'x' is infinite; otherwise, it returns false.
Example:
Output:
Example:
Output:
Checking for NaN
- Input: A real number
- Return Type: Boolean value
The function takes a number, 'x', as an argument and returns true if the number is 'NaN' (not a number); otherwise, it returns false.
Example:
Output:
Checking for finite
- Input: A real number
- Return Type: Boolean value
This function takes one number x as an argument and returns False if x is NaN(Not a Number) or infinity. Otherwise, it returns true.
Example:
Output:
Example:
Output:
Conclusion
- Using the math module in Python functions is more accurate than using conventional operators like 2**6.
- Some math module functions in Python have high accuracy and precision in their results.
- Utilizing functions from the math module in python is advisable, as they are highly optimized for faster and more efficient results.
- These math module functions can be used in your engineering project, some big neural network model, or anywhere where high-precision results are required in less time.