Multithreading in Java
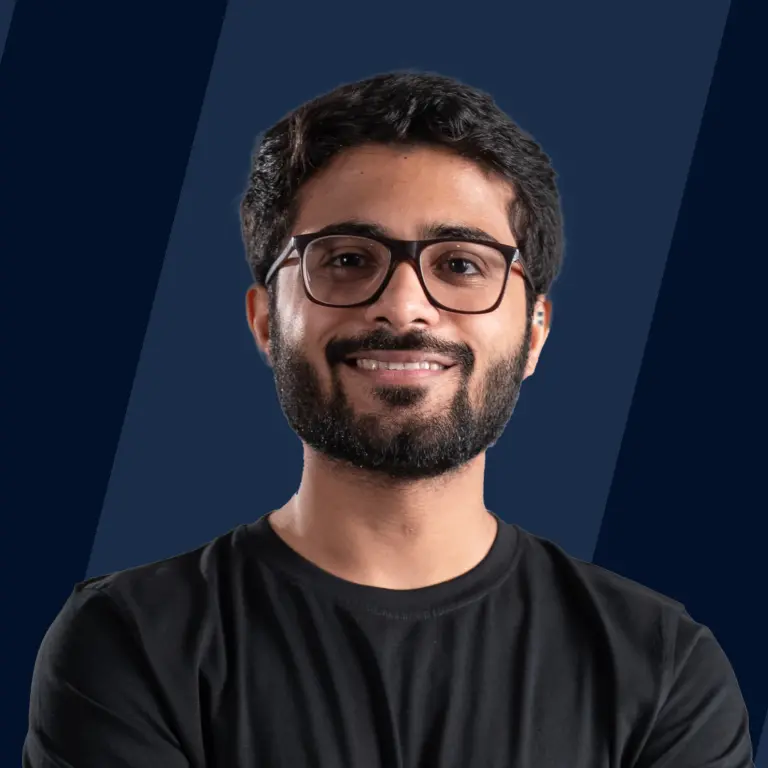
Overview
A thread is an extremely lightweight process, or the smallest component of the process, that enables software to work more effectively by doing numerous tasks concurrently.
Some of its uses include the fact that server-side programs employ it since a server should be multi-threaded so that many clients can be served at once.
Multithreading in Java is a process of executing multiple threads simultaneously. The main reason for incorporating threads into an application is to improve its performance. Games and animations can also be made using threads.
What is Multithreading in Java?
Multithreading is a feature in Java that concurrently executes two or more parts of the program for utilizing the CPU at its maximum. The part of each program is called Thread which is a lightweight process.
According to the definition, it can be deduced that it expands the concept of multitasking in the program by allowing certain operations to be divided into smaller units using a single application.
Each Thread operates concurrently and permits the execution of multiple tasks inside the same application.
Multithreading vs. Multiprocessing in Java
Multithreading | Multiprocessing |
---|---|
In this, multiple threads are created for increasing computational power using a single process. | In this, CPUs are added in order to increase computational power. |
Many threads of a process are executed simultaneously. | Many processes are executed simultaneously. |
It is not classified into any categories. | Classified into two categories, symmetric and asymmetric. |
The creation of a process is economical. | Creation of a process is time-consuming. |
In this, a common space of address is shared by all threads | Every process in this owns a separate space of address. |
How does Java Support Multithreading?
Java supports multithreading through its built-in features for creating and managing threads. It provides a Thread class that can be extended to create custom threads or Runnable interface to define tasks for threads. To use it, you can either extend the Thread class and override its run() method to define the thread's task or implement the Runnable interface and pass an instance of the class to the Thread constructor. The start() method is then called to begin the execution of the thread, which runs concurrently with other threads in the JVM, enabling multithreading capabilities.
What are the different types of threads?
In Java, there are two main types of threads: user threads and daemon threads. User threads are created by the application and continue to run until their task is completed or the application terminates. On the other hand, daemon threads are background threads that are automatically terminated when there are no more user threads running. These daemon threads are useful for tasks like garbage collection, etc.
What is the Use of Multi-Thread in Java?
- Because each Thread is managed individually and several operations can be carried out at once, the user is not blocked.
- It is used to save time as multiple operations are performed concurrently.
- Since threads are independent, other threads don’t get affected even if an exception occurs in a single thread.
Methods of Multithreading in Java
The following are methods used for Multithreading in Java.
Methods | Description |
---|---|
void start() | Causes the Thread to begin execution |
void run() | Utilised to carry out a thread's action. |
long getId() | Returns the identifier of the thread |
final String getName() | Returns the threads name |
final void setName(String name) | Sets the name of the thread |
final int getPriority() | Returns thread’s priority |
final void setPriority(int newPriority) | Changes the priority if the thread |
boolean isAlive() | It checks to see if the Thread is alive. |
void suspend() | It is used to suspend the Thread. |
void resume() | The suspended Thread is resumed using it. |
void stop() | The Thread is stopped using it. |
void destroy() | The thread group and all of its subgroups are destroyed using it. |
static void sleep(long millis) throws InterruptedException | Causes the currently executing Thread to sleep (temporarily cease execution) for the specified number of milliseconds. |
static Thread currentThread() | Returns a reference to the currently executing thread object. |
static void yield() | A hint to the scheduler that the current Thread is willing to yield its current use of a processor. |
final void join() throws InterruptedException | Waits for the Thread to die |
boolean isDaemon() | It checks to see if a thread is a daemon thread. |
void setDaemon() | It marks the Thread as daemon or user thread. |
void interrupt() | It interrupts the Thread. |
boolean isinterrupted() | It checks to see if the Thread has been interrupted. |
static boolean interrupted() | It checks to see whether the current Thread has been interrupted. |
static int activeCount() | It gives back how many threads are currently active in the thread group of the current Thread. |
void checkAccess() | It determines if the currently running Thread has permission to modify the Thread. |
static boolean holdLock() | It returns true if and only if the current Thread holds the monitor lock on the specified object. |
static void dumpStack() | It is used to print a stack trace of the current Thread to the standard error stream. |
StackTraceElement[] getStackTrace() | It returns an array of stack trace elements representing the stack dump of the thread. |
static int enumerate() | It is used to copy every active Thread's thread group and its subgroup into the specified array. |
Thread.State getState() | It is used to return the state of the Thread. |
ThreadGroup getThreadGroup() | It is used to return the thread group to which this Thread belongs. |
String toString() | It is used to return a string representation of this Thread, including the Thread's name, priority, and thread group. |
void notify() | It is used to give the notification for only one Thread that is waiting for a particular object. |
void notifyAll() | It is used to give notification to all waiting threads of a particular object. |
Examples of Multithreading in java
Implementation of Multithreading using java :
Example 1: To facilitate thread programming, Java offers the Thread class. To generate and manage threads, the Thread class offers constructors and methods. The Runnable interface is implemented by the Thread class, which extends the Object class.
Output:
Explanation: In this example, the class MultithreadingTest implements the class Runnable. The method run() prints the current Thread and its ID. If any exception occurs, then it is caught.
Example 2: Another Program to implement Multithreading in java.
Output:
Explanation: The class RunnableTest implements Runnable interface which has two private variables, Thread t and String threadName. The method run() prints the currently running Thread. After printing the threadName (Thread's name), the Thread sleeps for 50 microseconds.
Other Benefits of Multithreading in Java
-
There occur several I/O requests and concurrent computation operations within a single process. Thus the throughput is increased.
-
Total symmetric and simultaneous utilization of multiple processors for I/O and computation.
-
If a request is launchable on its own Thread, applications do not freeze or show loading indications (hourglass, rotating ring, etc.).
This implies that an entire application would not have to wait, which prevents pending the completion of another request. Thus the overall application responsiveness is improved.
-
Slow clients or huge or complex requests do not block other service requests. Therefore, the overall server responsiveness is also increased.
-
Threads produce tiny effects on system resources. A smaller overhead is required to create, manage, and maintain threads in contrast to a traditional process. Thus threads are easy on system resources.
-
Threads are used to simplify the structure of complicated applications. Simple routines can be written for every activity, thus making complex programs more adaptive to a broad alteration in user demands and more convenient to design and code. Thus the program structure is simplified.
-
Functions for thread synchronization provide stronger communication among processes. Furthermore, sharing big amounts of data within the same address space through different threads of execution provides very high bandwidth and low-latency communication between separate tasks within an application.
Conclusion
- All things considered, Multithreading in Java is a crucial concept that every Java developer should be familiar with.
- It greatly aids in increasing program efficiency and minimizing storage resource utilization.
- No matter how complex they are, it ensures that the CPUs are used to their fullest potential.
- Understanding the Thread class and Runnable interface is essential if you want to learn more about threads and why developers use them so frequently.