Not in Python
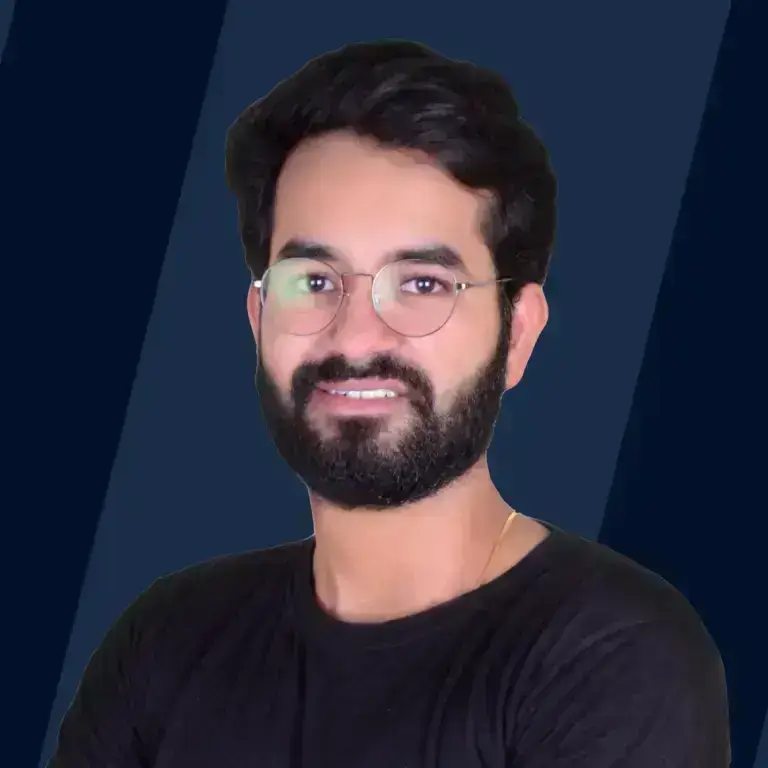
not in Python is a Logical operator which returns True if the expression evaluates to Falsy values and vice versa.
What is Not Operator in Python?
The not operator in Python basically reverses the logical value that the expression returns. It may be True/False depending on the expression evaluated.
The not operator in Python can be understood by the following table:-
If the expression returns | Not operator returns |
---|---|
True | False |
False | True |
Syntax
The not operator in Python can be used as:
Explanation
- not operator in Python performs logical negation to Boolean expression.
- Next, you have to mention an expression or a variable that has a boolean value associated with it.
- If the check is True, not will evaluate it as False and vice versa.
The not in Python is considered one of the basic operators used in Python, along with AND and OR operators.
Its function is similar to the inverter gate used in Digital Electronics.
How to Use Not Keyword in Python?
Python has only one unary Boolean operator, the not operator. This is a fancy way of saying that the operator only accepts one input. When you apply the not operator to input x in Python, you type not x.
The not operator in Python has two possible outcomes based on the input value: True or False.
Example 1
Code:
Output:
Explanation
- In the above example, the number variable is assigned to the value 10. It is used with the if statement, as shown.
- The expression checks if the number is greater than 10.
- In the above case, the number is assigned as ten which means that the expression will return False and not the operator, in this case, will return True.
- The complete statement inside the if condition returns true; hence if statement will execute.
Example 2
Code:
Output:
Explanation
- In the above example, the number variable is assigned to the value 10. It is used with the if statement, as shown.
- Any value other than zero, empty, or none object is considered true.
- When the number is checked with the if condition, the not in Python will return False.
- The complete statement inside the if condition returns False; hence the else statement will execute.
A Python Not With "in" Example
- Here, we'll see how to use the "not" operator with the "in" operator in Python. Following is an example where we have a list of integers assigned to a list named numbers.
- The not in Python here checks if the value 12 is present in the list or not.
- In the example below, 12 is not present in the list; hence it returns true, and the if statement gets executed.
Example
Code:
Output:
More examples of Not Keyword
Python not Operator with Variable
Code:
Output:
Explanation:
In this example, the not operator is applied to a boolean variable a with a value of False. The not operator inverts the value, resulting in True.
not in Loop Conditions
Code:
Output:
Explanation:
Here, the not operator is used to invert the loop continuation condition. The loop iterates over each element in the list until the index is not less than the list's length.
not with Function Return Values
Code:
Output:
Explanation:
In this scenario, the not keyword is used to invert the boolean result of a function. The function is_even checks if a number is even, and not is used to determine if it's not even.
not with Membership Testing
Code:
Output:
Explanation:
This example uses not with the in keyword for membership testing. It checks if 'mango' is not present in the fruits list and prints a message accordingly.
Combining not with all() Function
Code:
Output:
Explanation:
The all() function returns True if all elements in the iterable are true. The not operator is used here to check the opposite condition—whether not all elements are true, indicating the presence of one or more False values in the list.
Practical Applications
- The not keyword is primarily used to invert the boolean value of an expression, making True become False and vice versa.
- In conjunction with if statements, not is used to negate the condition being tested.
- The not keyword is often used together with the in keyword to check for the absence of a specific element within a collection.
Conclusion
So far, we have learned about the use of the not operator in Python.
- It is used with most programming languages that support logical operators.
- It returns the opposite value of the outcome of the expression that follows it immediately.
- It is mainly used to negate the values and logical operations.