What is Expression in Python?
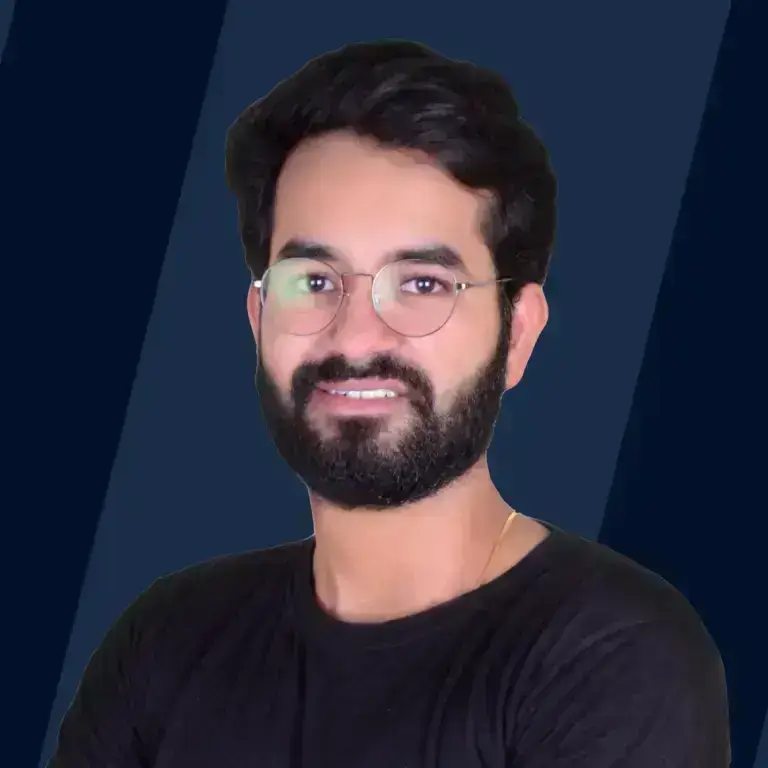
A combination of operands and operators is called an expression. The expression in Python produces some value or result after being interpreted by the Python interpreter. An expression in Python is a combination of operators and operands.
An example of expression can be : . In this expression, the first is added to the variable x. After the addition is performed, the result is assigned to the variable x.
Example :
Output :
An expression in Python is very different from statements in Python. A statement is not evaluated for some results. A statement is used for creating variables or for displaying values.
Example :
Output :
An expression in Python can contain identifiers, operators, and operands. Let us briefly discuss them.
An identifier is a name that is used to define and identify a class, variable, or function in Python.
An operand is an object that is operated on. On the other hand, an operator is a special symbol that performs the arithmetic or logical computations on the operands. There are many types of operators in Python, some of them are :
- + : add (plus).
- - : subtract (minus).
- x : multiply.
- / : divide.
- ** : power.
- % : modulo.
- << : left shift.
- >> : right shift.
- & : bit-wise AND.
- | : bit-wise OR.
- ^ : bit-wise XOR.
- ~ : bit-wise invert.
- < : less than.
- > : greater than.
- <= : less than or equal to.
- >= : greater than or equal to.
- == : equal to.
- != : not equal to.
- and : boolean AND.
- or : boolean OR.
- not : boolean NOT.
The expression in Python can be considered as a logical line of code that is evaluated to obtain some result. If there are various operators in an expression then the operators are resolved based on their precedence. We have various types of expression in Python, refer to the next section for a more detailed explanation of the types of expression in Python.
Types of Expression in Python
We have various types of expression in Python, let us discuss them along with their respective examples.
1. Constant Expressions
A constant expression in Python that contains only constant values is known as a constant expression. In a constant expression in Python, the operator(s) is a constant. A constant is a value that cannot be changed after its initialization.
Example :
Output :
2. Arithmetic Expressions
An expression in Python that contains a combination of operators, operands, and sometimes parenthesis is known as an arithmetic expression. The result of an arithmetic expression is also a numeric value just like the constant expression discussed above. Before getting into the example of an arithmetic expression in Python, let us first know about the various operators used in the arithmetic expressions.
Operator | Syntax | Working |
---|---|---|
+ | x + y | Addition or summation of x and y. |
- | x - y | Subtraction of y from x. |
x | x x y | Multiplication or product of x and y. |
/ | x / y | Division of x and y. |
// | x // y | Quotient when x is divided by y. |
% | x % y | Remainder when x is divided by y. |
** | x ** y | Exponent (x to the power of y). |
Example :
Output :
3. Integral Expressions
An integral expression in Python is used for computations and type conversion (integer to float, a string to integer, etc.). An integral expression always produces an integer value as a resultant.
Example :
Output :
4. Floating Expressions
A floating expression in Python is used for computations and type conversion (integer to float, a string to integer, etc.). A floating expression always produces a floating-point number as a resultant.
Example :
Output :
5. Relational Expressions
A relational expression in Python can be considered as a combination of two or more arithmetic expressions joined using relational operators. The overall expression results in either True or False (boolean result). We have four types of relational operators in Python .
A relational operator produces a boolean result so they are also known as Boolean Expressions.
For example :
In this example, first, the arithmetic expressions (i.e. and ) are evaluated, and then the results are used for further comparison.
Example :
Output :
6. Logical Expressions
As the name suggests, a logical expression performs the logical computation, and the overall expression results in either True or False (boolean result). We have three types of logical expressions in Python, let us discuss them briefly.
Operator | Syntax | Working |
---|---|---|
and | and | The expression return True if both and are true, else it returns False. |
or | or | The expression return True if at least one of or is True. |
not | not | The expression returns True if the condition of is False. |
Note :
In the table specified above, and can be values or another expression as well.
Example :
Output :
7. Bitwise Expressions
The expression in which the operation or computation is performed at the bit level is known as a bitwise expression in Python. The bitwise expression contains the bitwise operators.
Example :
Output :
8. Combinational Expressions
As the name suggests, a combination expression can contain a single or multiple expressions which result in an integer or boolean value depending upon the expressions involved.
Example :
Output :
Whenever there are multiple expressions involved then the expressions are resolved based on their precedence or priority. Let us learn about the precedence of various operators in the following section.
Multiple Operators in Expression (Operator Precedence) ?
The operator precedence is used to define the operator's priority i.e. which operator will be executed first. The operator precedence is similar to the BODMAS rule that we learned in mathematics. Refer to the list specified below for operator precedence.
Precedence | Operator | Name |
---|---|---|
1. | ( ) [ ] { } | Parenthesis |
2. | ** | Exponentiation |
3. | -value , +value , ~value | Unary plus or minus, complement |
4. | / * // % | Multiply, Divide, Modulo |
5. | + – | Addition & Subtraction |
6. | >> << | Shift Operators |
7. | & | Bitwise AND |
8. | ^ | Bitwise XOR |
9. | pipe symbol | Bitwise OR |
10. | >= <= > < | Comparison Operators |
11. | == != | Equality Operators |
12. | = += -= /= *= | Assignment Operators |
13. | is, is not, in, not in | Identity and membership operators |
14. | and, or, not | Logical Operators |
Let us take an example to understand the precedence better :
Output :
Difference between Statements and Expressions in Python
We have earlier discussed statement expression in Python, let us learn the differences between them.
Statement in Python | Expression in Python |
---|---|
A statement in Python is used for creating variables or for displaying values. | The expression in Python produces some value or result after being interpreted by the Python interpreter. |
A statement in Python is not evaluated for some results. | An expression in Python is evaluated for some results. |
The execution of a statement changes the state of the variable. | The expression evaluation does not result in any state change. |
A statement can be an expression. | An expression is not a statement. |
Example : . Output : | Example: . Output : |
Learn More
To learn more about the various Python operators and other related terms, refer to the articles :
Conclusion
- A combination of operands and operators is called an expression. The expression in Python produces some value or result after being interpreted by the Python interpreter. Example : .
- A statement is not evaluated for some result. A statement is used for creating variables or for displaying values.
- A statement can be an expression but an expression is not a statement.
- An expression in Python that contains only constant values are known as a constant expression.
- An expression in Python that contains a combination of operators, operands, and sometimes parenthesis is known as an arithmetic expression.
- An integral expression in Python is used for computations and type conversion (integer to float, a string to integer, etc.).
- A floating expression in Python is used for computations and type conversion (integer to float, a string to integer, etc.). A floating expression always produces a floating-point number as a resultant.
- A relational expression in Python can be considered as a combination of two or more arithmetic expressions joined using relational operators.
- A logical expression performs the logical computation and the overall expression results in either True or False (boolean result).
- The expression in which the operation or computation is performed at the bit level is known as a bitwise expression in Python.
- A combination expression can contain a single or multiple expressions which result in an integer or boolean value depending upon the expressions involved.