Python Program to Check If a Number is Palindrome or Not
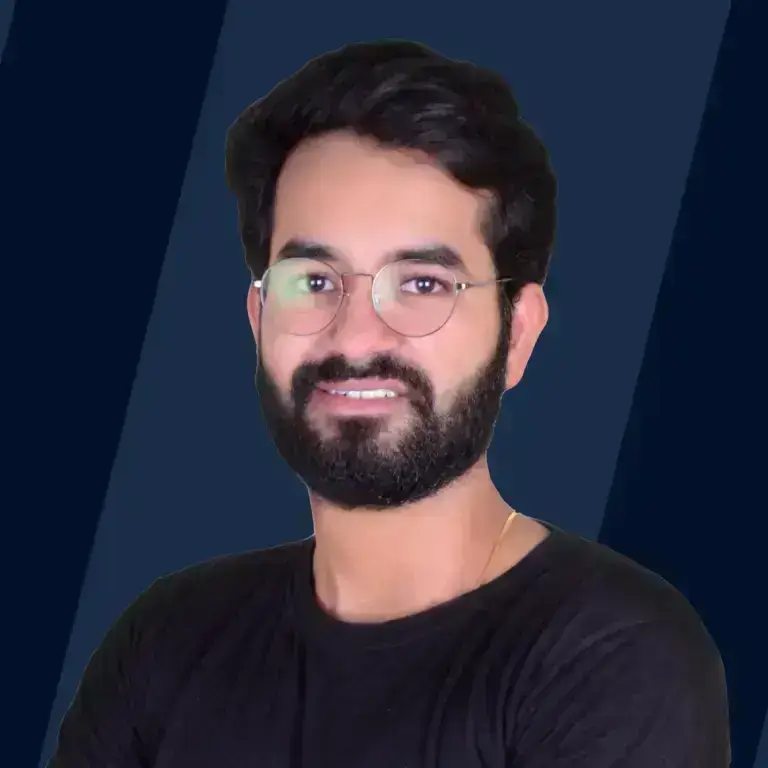
A palindrome in Python is a number, word, or another sequence of characters that reads the same forward and backward. We can use palindromes to solve various mathematical puzzles and computer science-related problems. This article will delve into various ways to check the palindrome in Python.
Python Program to Check If a Number is Palindrome or Not Using Native Approach
Output:
This program defines a function is_palindrome_native that takes a number as input and returns True if the number is a palindrome and False otherwise. It converts the number to a string and checks if it is equal to its reverse using slicing. Finally, it tests the function with a sample number 121 and prints the result.
Python Program to Check If a Number is Palindrome or Not Using Iterative Method
Output:
This palindrome program in python defines a function is_palindrome_iterative that takes a number as input and returns True if the number is a palindrome and False otherwise. It iteratively extracts the digits of the number, reverses them, and compares the original number with the reversed number. Finally, it tests the function with a sample number 12321 and prints the result.
Python Program to Check If a Number is Palindrome or Not using one extra variable
Output:
In this palindrome program in python, we define a function is_palindrome_extra_variable that takes a number as input and returns True if the number is a palindrome and False otherwise. It uses an extra variable, reversed_num, to store the reversed form of the original number. The rest of the logic is similar to the iterative approach. Finally, we test the function with a sample number 12321 and print the result.
Python Program to Check If a Number is Palindrome or Not Using Flag
Output:
In this program, we define a function is_palindrome_with_flag that takes a number as input and returns True if the number is a palindrome and False otherwise. It uses a flag is_palindrome to keep track of whether the number is a palindrome or not. The rest of the logic is similar to the iterative approach. Finally, we test the function with a sample number 12321 and print the result.
Python Program to Check If a Number is Palindrome or Not Using While Loops
It is an iterative method in which we find out the reverse of the given number by extracting the digits from the number and storing them in reverse order to check the palindrome. In this method, we follow the following steps:
- First, we take the input number n.
- Then, we make a copy of the input by assigning it to the temp variable (temp = n). Since integers are immutable in Python, a copy of variable n is stored in the temp variable.
- Initialize a new variable called reverse. It will be used to store the reverse of the input number.
- Now, we find out the reverse of the input number using the below-mentioned mathematical formula inside a while loop:
Here,
- The digit variable stores the remainder attained by dividing the variable n by 10.
- This remainder (digit) is then added to the reverse variable after multiplying the past value of the reverse variable by 10.
- At last, the input number n is divided by 10. This statement acts as the decrement statement for the while loop.
- Finally, we check whether the reversed number is equal to the original input number, i.e., check if temp == reverse is True or not.
Now, Let's look at an example to understand the while loop method:
Code:
Output:
To perfectly grasp the working of the above example, let's notice the value of every variable after each iteration of the while loop:
Initial Values:
First Iteration:
- = =
Second Iteration:
Third Iteration:
The while loop will terminate after the Third iteration, as the condition, i.e., n>0 results in False. Hence, we can check the palindrome in Python by using the modulus operation (%) and while loops.
Python Program to Check If a Number is Palindrome or not using Recursion
In this palindrome program in Python, we will check whether the first element of the sequence is the same as the last element, the second element is the same as the second last one, and so on. If any element mismatches, the number is not a palindrome. This method can be implemented using recursion.
Let's look at the recursion implementation of the Length Technique:
Code:
Output:
Here, when the function check_palindrome is called, the following steps take place:
- Firstly, we convert the input number n to a string using the built-in str() function.
- Then, we find out the length of the string using the built-in len() function to verify if the string is empty or just a single character long.
- Finally, the recursive case is executed in which:
- We check if the first character of the string (s[0]) is the same as the last character (s[len(s)-1]) or not. If it doesn't match, the program halts, and the function returns the value False. But, if it matches, then these two characters are removed from the string via slicing (s[1 : len(s) - 1]), and the new string is sent as an argument to the recursive function.
- For a palindrome number, this process is continued until we are left with the string of unit (input number has odd digits) or zero-length (input number has odd digits), i.e., the base case is attained.
- Hence, in every function call, we are reducing the sequence size by 2.
Python Program to Check If a Number is Palindrome or Not Using Built-in Functions
We can also check palindrome in Python with the help of the built-in reversed() function provided for sequence data types in Python:
- reversed(sequence): It returns an iterator that accesses the sequence passed as an argument in the reverse order.
To check palindrome in Python using the built-in reversed(sequence) function, we can convert the input number to a sequence and find the reverse of the sequence using the reversed method. After which, we can compare these two sequences to check the palindrome.
Let's look at an example to understand the concept of the reversed() function:
Output:
Here, we are converting the input number n to a string data type using the str() function and storing it to a variable seq. Then, we check whether the result of the reversed(seq) function is equal to that of the string seq. If it matches, the input number n is a palindrome.
Palindrome in Python Using Slice Operator
Another way in which we can make use of sequences to check palindrome in Python is by using the slice operator:
- Slice operator [n : m : step-size] - It returns the part of the sequence starting from the nth element to the (m-1)th element by iterating the sequence according to the defined step size.
After converting the input number to a sequence, we can generate a reversed sequence by using a negative integer as the step-size argument. This reversed sequence is compared with the sequence generated from the input number to check the palindrome. It is the simplest way to check the palindrome of a number in Python.
Let's look at an example to understand the use of slicing to check palindrome:
Output:
Here, s[::-1] indicates that the step size is -1, and since no other arguments are given, it iterates the whole list in reverse order.
Note- For a large number, the built-in function reversed(sequence) performs better than the slice operator. The reversed(sequence) method returns an iterator that accesses the sequence in reverse order. At the same time, the slice operator creates a new list that contains the elements in reverse order as that of the original list.
Conclusion
- To check if a number is palindrome, you must find its reverse first.
- The native approach and the iterative method provide straightforward solutions.
- Using one extra variable or a flag introduces additional variables for storing the reversed number.
- The while loop method offers an iterative approach with direct manipulation of the number.
- The recursion method of the palindrome program in Python provides an effective solution but may be less efficient for large numbers.