Pass in Python
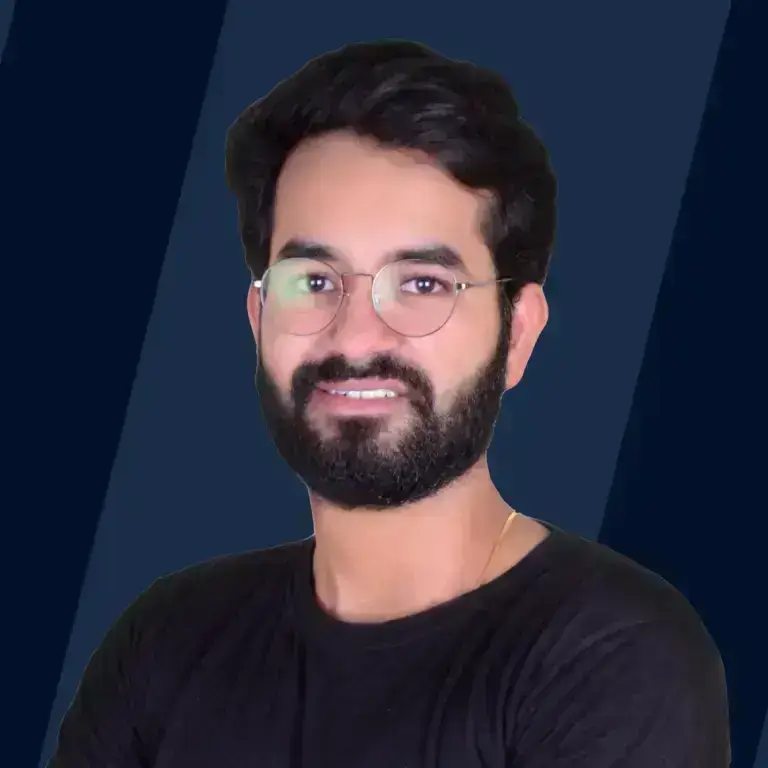
Overview
pass in Python is a null statement which the interpreter simply 'moves over' while executing the code. It can be used in a variety of scenarios where you want to leave parts of your code undefined (such as classes or methods) but the Python syntax doesn't allow it.
What is Pass Statement in Python?
Have you ever felt that you need to test your code/program file, with the exception of a few functions, classes, etc. which you intend to complete later or assign to someone else, but you've declared them so that you remember to complete them later?
- One option would be to remove those functions, classes etc. at from the code, test it for now, and add them later. But sometimes it makes it harder to pick up from where you left.
- Another option would be to just declare them (thus they wouldn't perform any task whatsoever) and test the rest of the functioning code.
The second way is a more practical solution since it helps programmer pick up from where the last changes were made. And in Python, we can achieve this using the pass statement.
pass is an executable statement in Python that does not perform any meaningful work, that is, it is a no-op (no-operation) statement. The program simply moves forward as if nothing had to be executed.
Unlike comments which are completely ignored by the interpreter,pass statements are executed. But their execution does not result in any sort of computational output.
Pass statement is often used in places where -
- We don't want some specific part of code to do anything
- All we want to do for the time being is just declare some functions, methods, classes, etc. but we don't want to implement them completely. Maybe it has to be given to some other team member, or maybe all relevant information is not available right now, or it is just to be scheduled for later.
The pass statement in Python is highly useful in such cases. Why do we need a pass statement at all, can't we leave it blank? It is because Python doesn't allow an empty body for:
- classes and methods
- functions
- loops
- conditional statements
Leaving their definition body blank leads to an error as will be shown in the examples below. We can use the pass statement to just declare them (the classes, functions, etc) and then "pass" along for the time being.
IMPORTANT
It is important to understand that the pass statement doesn't exit control of the program from the given scope after being executed. If there are some other executable statements following pass within that same block of code, they will be executed.
Therefore, pass IS NOT an alternative for the following statements:
- break
- continue
- return
Syntax of pass in Python
pass has a very simple syntax. In any line or indentation where you want to skip the execution, just type:
We will understand it better using the following examples.
Using pass Statement in Empty Functions
We can define empty functions in Python by using the pass statement in the function body.
Example:
This function is exactly similar as:
We can also verify this by running the code and observing the output.
Code:
Output:
As you can see, both these functions have equivalent functionalities. After entering into the scope of the myFunction1, since the program finds nothing to execute after the pass statement, it returns None to where it was called.
Example: Creating empty functions in Python using pass. The code below shows the error we get when we leave an empty block in a Python function definition.
Code:
Output:
Let's see the correct way to leave a function undefined using the same code.
Code:
Output :
Since we defined the function with a pass statement, we got a function with a None return value.
IMPORTANT
As was mentioned earlier, pass is not an alternative for return, break, or continue statements.
If there are statements after pass in a given code block, they will be executed. See the following code block for understanding it better.
Code:
Output:
As you can see, all the statements present inside the function addnums after pass are executed and even the returned value is no longer None.
Using pass Statement in an Empty Class
Classes, just like functions, cannot be left undefined in Python. The only way we can just declare a class and not define it is using pass statement, docstring, or a dummy statement. Here we will focus only on the first one.
Example: Let's see the error we get when we leave a class body empty
Code:
Output:
The interpreter was expecting some executable code within the class body before it went back to the parent indent, but it showed an error since it found nothing to run (comments are always ignored by the interpreter)
Let's the correct way to leave the class undefined for the time being using the pass statement.
Code:
Output:
Here we can see that wheel1 is an object of the class Wheel. The program does not show any error even though the class has no data members or methods. When an object of this class is instantiated, it is also without any data members or methods. pass statement makes it possible to create such objects.
Interesting ⚡
Since Python classes and objects are very dynamic in nature, we can do some interesting things even with empty classes. The example shown here displays the concept of instance variables in Python. You can read more about this behaviour here.
Output:
The instance variable name is created at runtime for the empty class A, and it works even though there is no pre-defined data member called name. It is important to understand this "data member" is available only to the object a, because it is created/added to the object during the runtime. Any other object of the class A will have to get its own instance variable explicitly defined.
Example:
Output
This will give an error since b has no attribute called name. Correct code will be:
And the corresponding output is:
Using pass Statement in Loop
As we saw in the case of functions and classes, we're also not allowed to leave an empty body in a loop. If we want to implement the loop later, or just leave it for someone else to implement, all we have to do is write a pass statement in the loop's body. Let us understand it through some examples.
Example:
In case of while loop
Code :
Output:
Yes, there will be no output! Since there is a pass statement inside the while loop, the value of i is never changed. The condition i==10 will remain true always, and therefore, we have entered our program into an infinite loop.
The syntax in the case of for loop is also quite similar to what we discussed above.
Code:
Output:
Notice that here we did not enter an infinite loop. It is because the for loop iterates until the iterable (which, here, is the list a) gets completely exhausted. Though it is not impossible to emulate the infinite loop scenario using for-loops. It can be done using iter() as shown in the code below.
Code:
Discussing the iter() method is out of the scope of this article, and therefore the readers are implored to look up literature on the same on the internet.
Here too, remember it is not an alternative to a break or continue statements. The following two code samples will make it clear.
Suppose we want to write a program to come out of the loop as soon as we encounter 5 in the list.
Output:
As you can see, the program iterated over all the elements of the list, and neither did it break the loop nor continued itself for the next iteration. The correct way to achieve our aim would have been to use break instead as shown below.
Code:
Output:
As you can see, the loop program broke out of the loop as soon it encountered 5 in the list.
Using pass Statement in Conditional Statement
pass statement is often used in conditional statements (if, else, and elif) to make the code more readable. We always have the choice to skip the conditional cases where our code would be doing nothing, but to explicitly convey it to the reader, we can use the pass statement. Examples given below will make it more clear.
Example:
Consider a program that prints "Hello" in case the input number is less than or equal to 10, and prints "World" if the number is greater than 20.
Code:
Output:
We could also write the program using pass statement, and depending on the programmer's style of writing code, they could consider either one of these as being more readable since it shows a clear gradation of all possible cases.
Code:
Output :
Output :
As you might have guessed, in the second case when n = 17, the program entered the elif block and after executing the pass statement, it terminated. There was nothing else for the program to execute.
Similar to classes, functions, and loops, conditional statements are also not allowed to be left empty. Try it yourself and see it in your code editor.
Conclusion
We learnt the following things about pass statement in Python:
- It is the standard no-op (no operation) statement in Python
- It is different from a comment since it is not ignored, but executed by the program
- pass is highly useful when the definition of some function, class, etc. is to be scheduled for later.
- Its execution does not result in any form of computational output
- We can use it to define empty a. classes and methods b. functions c. loops d. conditional statements
- As a corollary for point 5, the execution of any code block does not stop at pass, if there are any statements following the pass statement, they will be executed.