Basic Python Syntax and First Program in Python
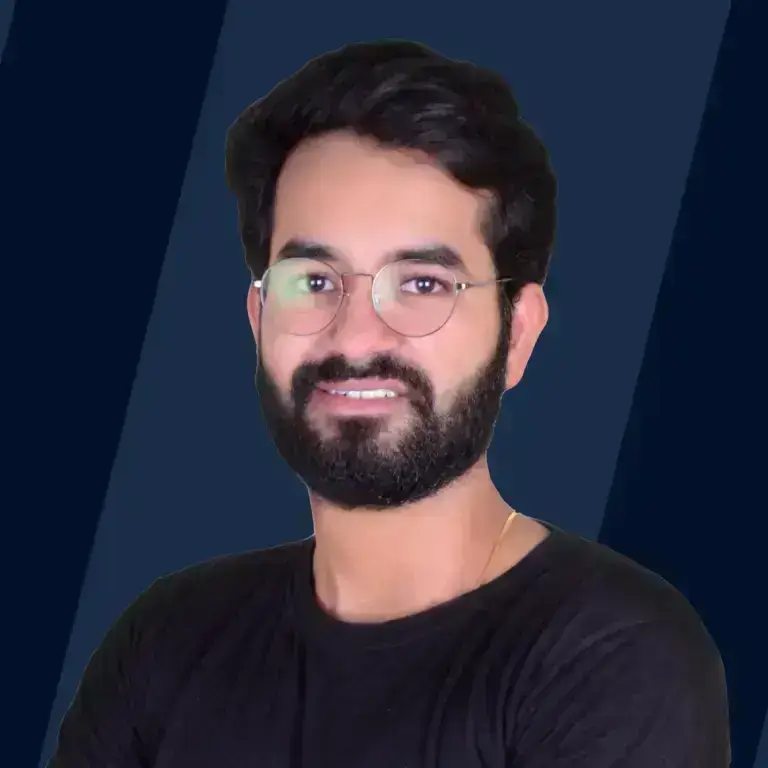
Overview
Syntax is one of the basic requirements that we must know to code in any language. Talking about Python Syntax, we use indentation to represent the block of the code. We have to follow some rules about how to use the indentation. Not just indentation, we have to follow the rules on how to choose the name for the variables.
Introduction
In this article, we will understand the basic syntax of Python.
What is Python Syntax?
Before we move on to understanding python syntax, let’s understand how to write and run a basic Python program.
There are two ways to do so:
1.Interactive mode – In this mode, you write and execute the program
2.Script mode – In this mode, you first create a file (.py file), save it, and then execute it.
Enter the following command in your terminal after installing Python to enter the interactive mode.
$ python
You have now entered the interactive mode. You can run any of the Python statements in the interactive mode in the terminal now. But if you’re using a script editor / IDE, you do not have to do the same.
Now we can move on to understand the python syntax. When we learn the Python syntax, we first start from the basic syntax of python like studying the print statement.
Print Statement
Let us run the Python first program in the terminal now
The output for the print statement is:
Now we discussed the interactive mode above, what about the script mode? To run code in the script mode, you must first create a .py file, write your code and save it. You can write the same code as above (the print statement). Let’s say you saved it as syntax.py. Once you’ve done that, you can either use the ‘run’ button in your IDE or if you would like to run it on your terminal :
Once you execute the command, you see that it prints – Syntax of print on the terminal. Congratulations! You’ve learned your first basic Python syntax.
Check out this article to know more about print() function in Python.
Indentation in Python
Whenever we write any piece of code in Python, say, functions or loops, we specify blocks of code for them. How will we identify which block is for what? It is done by “indenting” statements of that block according to the Python syntax.
To determine the line's indentation level, we use a number of leading whitespaces, which could be spaces or tabs. The General notion is to use one tab (or four spaces) for a single indent level.
Let’s take a look at an easy example to understand indentation levels.
We will not go into the code and what it does; instead, let’s focus on the indentation levels.
It marks the beginning of the function, so every line of code that belongs to the function has to be indented by one level at least. Notice that the print statements and the if statements are indented by at least one level. But what about the last print statement? As we see, it is indented by 0 levels, which means that it is not part of the function.
Let’s now move inside the function block, which is every statement below the function beginning that is indented by at least one level.
The first print statement is indented by one level because it is only under the function and not any other loop or condition. In contrast, the second print statement comes under the if statement.
The if statement is indented by just one level, but any block of code that has to be written under it should be indented by one more level than the if statement. The same goes for the else statement.
Now, in the indentation of code in Python, we have to follow specific rules. They are :
- Indentation cannot be split into multiple lines with the use of the backslash (‘\’) character
- An IndentationError will be thrown if you try to indent the first line of code in Python. You cannot indent the first line of code.
- If you have indented your code either by using a tab or space, it’s recommended to continue with the same spacing preference throughout your code. Do not use a mix of tabs and whitespaces to do so, as it may cause the wrong indentation.
- It is best to use 1 tab (or 4 whitespaces) for the first level of indentation and continue adding 4 more whitespaces (or 1 more tab) for higher indentation levels.
Indenting code in Python has benefits like making the code look beautiful and structured. It gives you a good understanding of the code in a single look. Plus, the indentation rules are simple, and if you’re writing code on an IDE, then most IDEs would automatically indent the code for you.
One disadvantage of indentation is that if your code is extensive and involves high indentation levels, even an indentation error in a single line can be very tedious to fix.
Let us look at some indentation errors to make your understanding crystal clear.
As discussed above, since we cannot indent the first line, and Indentation Error is thrown.
It will throw an IndentationError because the second print statement is written with one level indent, but it has extra whitespace, which is not allowed in Python.
In the code above, the print statements inside the if and else are not indented by one extra level, and hence IndentationError will be thrown.
Python Syntax Identifiers
When we talk about variables, functions, classes, or modules, we use “identifiers” to identify them. What are identifiers? It is a name given to something to differentiate it from other code entities.
Now just like for indentation, we have some naming rules here.
- The identifier will contain a combination of lowercase (a-z) or uppercase(A-Z) alphabets or numbers (0-9) or underscore (_)
- The Identifier cannot start with a digit
- Any reserved words or keywords cannot be used as identifier names (you’ll read more about keywords in the article soon)
- Symbols or Special characters cannot be used in the identifier
- Length of the identifier can be variable; there is no restriction
- Remember that Python is a case-sensitive language, so var and VAR are two different identifiers
Examples of correct naming: var, Robot, python_007, etc
Examples of incorrect naming: 97learning, hel!o etc
Some identifier examples for variables:
var = 310
My_var = 20
My_var344 = 10
STRING = "PYTHON"
fLoat = 3.142
Some examples of functions :
To check if your name is a valid identifier or not, you can use the inbuilt function “isidentifier()”.
It can be used as :
The first one is a valid identifier; hence it will print True, and the second one is invalid and will print False.
There is a catch with this function, though. You cannot use any keywords to check if they’re valid identifiers or not. This function will show True for any keyword, but you know that keywords cannot be used for identifiers. Hence, here this function has a caveat.
Now we discussed keywords and reserved words multiple times in this section, let’s take a look at them.
Python Keywords
Listed below in the table are the keywords in Python. They are some unique purpose words that can be used only for specific cases and not as identifiers.
False | None | True |
__peg_parser__ | and | as |
assert | async | await |
break | class | continue |
def | del | elif |
else | except | finally |
for | from | global |
if | import | in |
is | lambda | nonlocal |
not | or | pass |
raise | return | try |
while | with | yield |
To view all the keywords your version of python supports, you can open your script editor or IDE or write the following two commands on your terminal to list all the keywords.
Python Variables
What are variables? To store any data values, we use containers called variables. There is no specific command in Python to declare a variable. A variable is created the moment a value is assigned to it.
For example:
It will give us the output –
In most languages, you are required to declare variables with a particular type, as in the type of value they hold. It could be int, char, etc. But this is not required in Python. You can declare a variable ‘x’ as an integer (by assigning an integer value) and then change it to any other type as a string (by assigning a string value).
The variable names given follow the rules of identifiers that we discussed above.
Click here, to know more about Variables in Python.
Comments in Python
First, let’s understand why we need comments or why commenting in a program is essential. Of any program, comments are an integral part. We can make multiple-line comments as docstrings or single-line comments.
Writing comments is essential when you’re reading your code. It makes the code simpler to understand. You may even make specific comments in between to check and edit certain blocks of code. Or even later, say after six months, you have to make some changes in your code. The comments that you make throughout would help you understand your code.
Say you’re working in a team. You understand the code that you’ve written and do not require comments. But it would be challenging for others to understand your code if you don’t explain it in short lines as comments.
Now that we know why comments are critical let’s understand how we can include them in our Python programs.
To write a simple single-line comment, you must add the # symbol before your comment. Like this :
You need not add it on a new line. You can even add it after statements in your code.
According to the Python syntax, anything that is written after the # symbol is ignored by Python. It will not run.
Now say you want to write multiple comments together, as in a multiline comment, you cannot do it directly in Python. Most languages just use /* … */ for multiline comments.
However, you can do this :
If you don’t want to constantly hit enter and add the # symbol at the start of every line, you can make it a docstring by adding three double quotation marks.
Let me also give you a short trick on commenting on multiple lines of code all at once. Select the lines of code that you want to convert into comments, and press Ctrl + / together in Windows or Command + / in Mac. That would make your job much easier!
That’s all you need to know about comments in Python!
Multiple Line Statements
Before understanding multiline statements, let us take a look at statements.
Each of these lines is a statement. What if some statements are lengthy and you cannot fit them properly in one line? That’s when multiline comments come in handy.
Using the continuation character backslash, you can explicitly divide a statement into multiple lines (“\”).
For example
Did you also know that we can write multiple statements in one line?
The following three statements have been written in a single line using the semicolon (;).
Quotation in Python
Three types are used when it comes to quotation marks accepted by Python according to the Python syntax.
Single – ' ' Double – " " And Triple – " " " … " " "
We have already seen the usage of the triple quotation marks in the comments section. Single and double quotations are used to declare strings in Python.
Blank Lines in Python
There are three ways to print blank lines in Python according to the Python syntax.
The first and simplest way is to use a blank print statement:
Output:
Another way is to put empty quotation marks in the print statement, single or double.
Output:
The third way is to use a newline character (\n) at the end of the statement:
Output:
Waiting for User
Taking input in the Python programming language is done by the input() function. The program with input() function will not run till the program is not provided input by the user through the console.
Example –
After the second line, the program waits for the user to enter input.
Command Line Arguments
To keep our program’s nature generic, we use command-line arguments. For example, if we have written a program to parse a CSV file, inputting a CSV file from the command line would make our program work for any CSV file, making it generic.
Command-line arguments also make our program more secure. How? We need to put in some login credentials in the code to use them somewhere. If we add them to the script, they may be accessed by anyone and even executed. But on the other hand, if we use the command line to enter the login credentials, it becomes safer.
So how do we pass command-line arguments in Python?
It’s easy. You need to run the Python script from the terminal the way we discussed at the beginning of the article and add the inputs after that.
Here, code.py is the script's name, and arg1 … argN are the N number of arguments required as input from the command line.
You must be wondering how one would read the command line arguments? The Common way is to use the sys module for it.
Sys module
The sys module has a variable named argv. All the command line arguments are stored as a list of strings.
If you want the first command-line argument entered, you can access it by argv[0].
Example:
The first line of the program is just importing the sys library. In the second line, we’re using an if statement to check the length of the user input. Since we’re asking for two values (name and age), we need precisely two values in the command line input. If it is lesser or greater than that, the program is set to raise an error.
In the print statement, you can see that we have used the sys.argv[i] to fetch and print the input.
To run this program, in the terminal, we write :
Laila and 23 are the two command-line inputs we’re giving to the python program.
Its output will be:
If you don’t enter two arguments, you will see a value error.
It is the most commonly used module; however, there are more modules such as getopt or the argparse module.
FAQs
Q. What is the Python syntax for comments?
A: In Python, you can add comments to your code using the hash character (#). Anything written after the hash symbol on the same line is considered a comment and is ignored by the Python interpreter. Comments are useful for adding explanations or notes to your code. Here's an example:
Q. What is the significance of the if _name_ == "_main_": statement?
A: The if _name_ == "_main_": statement is often used in Python scripts. It checks whether the script is being run directly as the main program or if it's being imported as a module. The code inside this block will only execute if the script is the main program. This is useful when you want to include test code or specific actions that should only run when the script is executed directly.
Q. How do you declare and use variables in Python?
A: In Python, you can declare a variable by assigning a value to it using the equals (=) operator. Unlike some other programming languages, you don't need to explicitly specify the variable type. Python dynamically determines the type based on the value assigned to it. For Example:
Conclusion
-
There are 2 ways to write and run a Python program: interactive mode and script mode.
-
Python Syntax for the print statement is – print(‘Syntax of print’)
-
After every line of code that ends with ‘:’, python expects an indented code block. It is usually done with 4 whitespaces or 1 tab.
- Your code can throw an indentation error if there’s extra whitespace, an unexpected indent, or no indent.
-
Identifiers in Python are used to identify variables, classes, functions, or objects. Some correct naming methods are – var, Robot, python_007, etc.
-
Python has 36 keywords which are essentially reserved words that cannot be used as identifiers.
-
Variables are containers that can be used to store any values. Variable names follow the same rules as identifiers.
-
In python, you can use the ‘#’ symbol to write single-line comments like this :
# this is a comment
or write multiline comments like this:
"""
This is multiline.
Written in 3 quotations.
"""
-
If a statement is too long, it can be broken down using the ‘\’ character and written in multiple lines. Alternatively, you can write multiple short statements in one line by terminating each statement with the ‘;’ semicolon.
-
In Python, three types of quotation marks are used. The single and double quotation marks are for strings, while the triple quotation marks are for multiline comments according to the Python syntax.
-
The three ways to add a blank line in Python are:
- Use the empty print statement.
- Use print statements with empty single or double quotation marks
- Use the newline character (\n)
-
The input() function allows us to wait for user input. The program will not proceed further without user input according to the Python syntax.
-
Using the sys module, we can take command-line arguments