Python Length of List
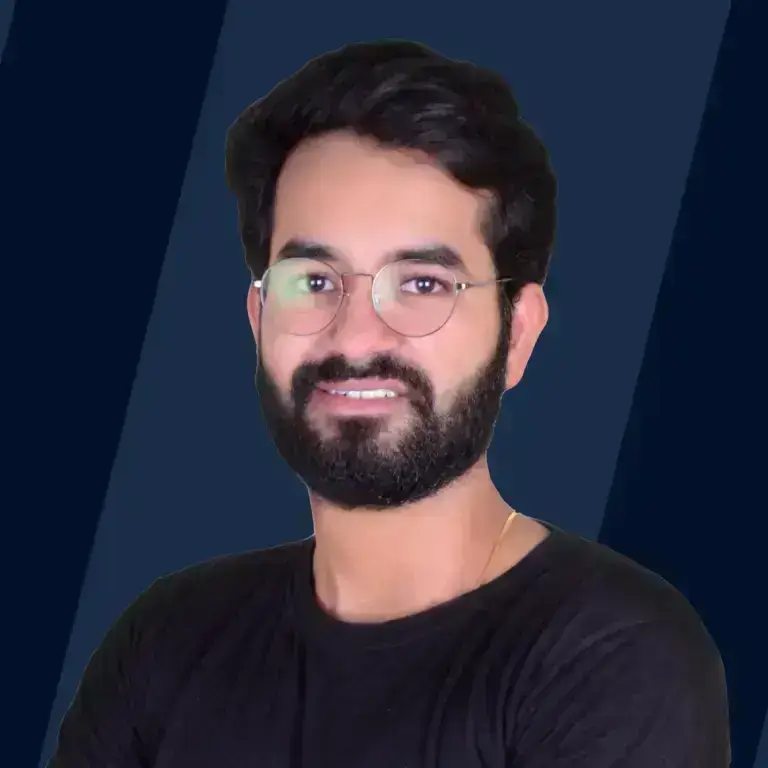
Overview
There are various methods to find out the length of the list in python. Two methods len() and length_hint() are inbuilt methods. They take iterable as an argument and return the length of the given list. The third method is the naive method, as it is simple and can be implemented by anyone. It uses a loop and a counter to calculate the length of the list.
How to Find the Length of List in Python?
List in Python is one of the four built-in methods, it is used to store multiple items under a single variable. Items in the list are ordered, and changeable, and they can be duplicated as well.
The list data structure in python stores a collection of elements. These elements need not be of the same data type. The list can have duplicate entries as well.
All the items in the list are separated by a comma , and enclosed with the square brackets [].
For example:
The above list contains String 'Hello', Character 'A', float 90.32, integer 5673, and another list[1,2,3] as its elements.
To find the length of lists in python, there are the most commonly used and basic methods:
Naive Method
The naive method is a very general approach and it does not require any predefined methods. We simply run a loop and increase the counter until we come across the last element of the list. This strategy can be used with multiple data structures and in almost all programming languages in the absence of other efficient techniques.
Let us take an example and find the length of the given list using Naive method.
Output:
As we can see in the above example, the for loop visits each element of the list. Variable count is incremented with each element. In the end, we simply return the count.
Using len()
The len() method takes an iterable as a parameter and returns the length of the iterable. It is the most conventional and most used technique to find the length of most of the data structures or objects in python. It is a built-in function in python that returns the length of a list, tuple, array, dictionary, etc.
Working of len()
In python, internally a variable storing the length of the list is initially created with the creation of the list. len() function calls the method __len__(). This __len__() method is pre-defined in classes of iterables. This attribute is cheap and easy to maintain. It is incremented if we add elements to the list and is decremented in case an element is removed from it. The __len__() method uses the variable to return the length of the list.
Syntax:
len(list) returns the size of the list as an integer. We can store it in a variable or simply print it in output.
Example:
Let's say we have a list we wish to divide into two halves. squares stores the actual list. list1 and list2 will be two lists such that list1 will store the first half of squares and list2 will store the other half of the squares.
Output:
In the example above, square stores the squares of the first 10 numbers. list1 will store the first 5 numbers of squares and list2 will store the remaining 5 numbers from squares. To divide it into two halves we are using the length of the list. We divide the length by 2, run the for loop for both the ranges (i.e. 0 to length/2 and length/2 to length) and append the elements to the respective lists.
Length of the List
The list can contain another list as well. To find the length of a parent list containing another list len() method is used.
For example:
Output:
parent_list in the above example contains 3 elements, two of which are lists and a string. The length of the parent list using the len() function is 3. Thus, it does not count the elements of the sub-lists. The length of the inner lists is also given by the len() function.
Using length_hint()
The length_hint() function is present in the operator module. It tells the number of items present in the particular list. It takes an iterable as an argument and returns the length of the object.
It is more advantageous than len() as it can handle more data types but the len() function is more reliable as this method doesn't always return the exact number of items. It is a lesser-known function in python to find the length of the given list.
Just like len() method it also calls __len__() for the length of the list. The __len__() internally stores a variable along with list elements. This variable is incremented when an element is added to the list and is decremented when an element is removed.
Let us take an example of a list and find its length using the length_hint() function
Output:
As we can see in the above example, length_hint works the same way as len() method. We are required to import it from operator.
Naive vs len() vs length_hint() Comparison
Naive | len() | length_hint() |
---|---|---|
Doesn't call any method for stored value. | It internally calls the __len__() method. | It also calls the __len__() method. |
It is always accurate. | It is always accurate. | It is not always accurate |
The naive method visits each element of the list. Let us say the list has n elements thus time complexity of a loop running to visit each element is O(n). | As it directly gets the value of length from the variable, the time complexity of len() is O(1). | The time complexity of length_hint() is O(1) as well. |
Less efficient as compared to the other two methods. | Efficient as compared to the Naive method. | Efficient method. |
Thus, we can say the order of efficiency is as follows len() < length_hint() < Naive Method
Conclusion
- The len() method in python takes iterable as an argument and returns the accurate length of the list.
- The length_hint() method in python works the same way as len() method, it applies to more data types than len() method. Also, it does not always return the exact number of elements in the list.
- Naive method uses a loop and a counter. It visits each element in the list and increments the counter. It is easy to implement and doesn't require pre-defined functions.
- Time complexity of the Naive method is O(n) whereas that of len() and length_hint() is O(1). Thus, len() is the most efficient function and the naive method is the least efficient method to find the length of the list in python.