len() Function in Python
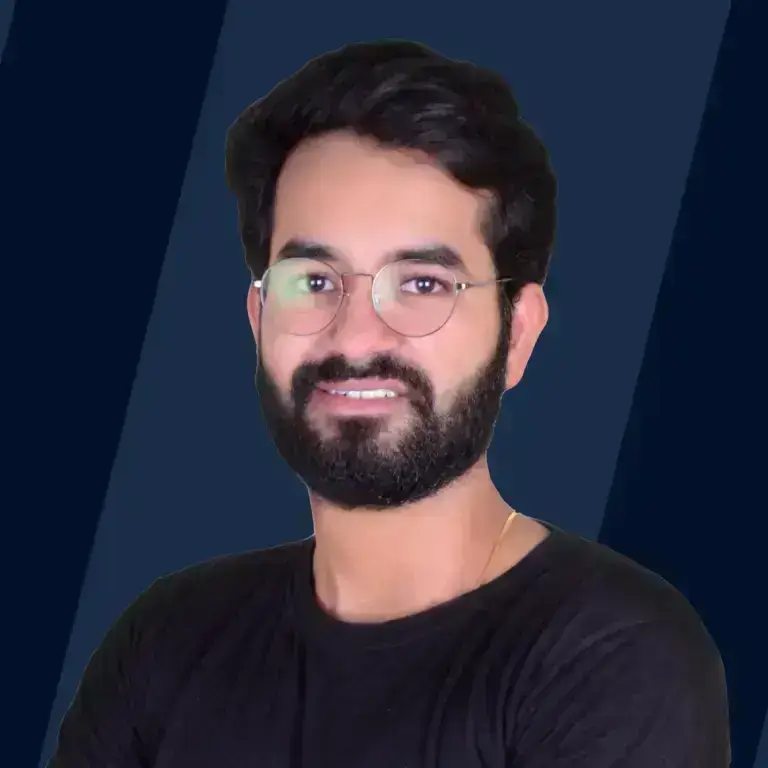
The len function in Python takes an object as an argument and returns the length of that object. For example, the len() function can return the number of items in a Python list.
Syntax of len() Function in Python
The syntax of len() is:
Parameters of len() Function in Python
The len function in python takes a single argument, which can be:
- sequence - string, bytes, iterables like tuple, list etc
- collection - dictionary, set, frozen set etc
Return Values of len() in Python
The len() function returns the number of elements in a sequence or collection object.
Find the length of built-in Sequences
A sequence is an object containing items in an ordered manner. Lists, tuples, and strings are the basic examples of built-in sequences in python, and the len() function is used to calculate the lengths of these sequences.
Code:
Output:
Explanation:
In the above code snippet, to find the length of the string name, the list food_items, and the tuple having coordinates, we use len() similarly. Therefore, all these three data types are valid arguments for len(). The function len() returns an integer as it counts the number of items in the object that is passed as an argument. The function returns 0 if the argument is an empty sequence.
We can also use range() to create a range object sequence. A range object doesn’t store all the values but generates them when they are needed. However, we can find the length of this range object using len().
Code:
Output:
Explanation:
This range of numbers includes the integers from 1 to 9 (10 is excluded). We can determine a range object's length using the start, stop, and step value arguments.
len() with tuples, lists and range
len() with Tuples
Tuples are immutable sequences in Python, used to store collections of heterogeneous data. The len() function can be used to find out the number of elements in a tuple.
Code:
Output:
Explanation:
In this example, my_tuple contains three elements. The len() function counts these elements and returns the total count, which is 3.
len() with Lists
Lists in Python are mutable sequences, capable of storing an ordered collection of items. The len() function is used to count the number of items in a list.
Code:
Output:
Explanation:
The list my_list has five elements. Applying len() to this list returns 5, indicating the list's length.
len() with Range
The range type represents an immutable sequence of numbers and is commonly used for looping a specific number of times in for loops. len() can determine the number of items in a range.
Code:
Output:
Explanation:
my_range is defined from 1 to 9 (10 is exclusive). Thus, there are 9 numbers in this range. The len() function, when applied to my_range, returns 9, reflecting the total number of elements in the range.
len() with strings and bytes
len() with Strings
In Python, a string is a sequence of Unicode characters. The len() function can be used to find out the number of characters in a string, including spaces and special characters.
Code:
Output:
Explanation:
The string my_string contains 13 characters, including letters, a comma, a space, and an exclamation mark. The len() function counts all these characters and returns the total count, which is 13 in this case.
len() with Bytes
Bytes in Python are sequences of byte (8-bits) numbers ranging from 0 to 255. They are commonly used for binary data manipulation and file operations. The len() function is applied to byte sequences to count the number of bytes.
Code:
Output:
Explanation:
In this example, my_bytes is a bytes object representing the string 'Python'. Each character is encoded into a single byte (since all characters in 'Python' are within the ASCII range), resulting in a total of 6 bytes. The len() function, when applied to my_bytes, returns 6, indicating the number of bytes.
len() with dictionaries and sets
len() with Dictionaries
A dictionary in Python is a collection of key-value pairs, where each key is unique. The len() function can be used to find out the number of key-value pairs in a dictionary.
Code:
Output:
Explanation:
The dictionary my_dict contains three key-value pairs. The len() function counts these pairs and returns the total count, which is 3 in this case. It's important to note that len() counts the number of keys, not the individual elements within each key or value.
len() with Sets
A set in Python is an unordered collection of unique elements. The len() function is used to count the number of elements in a set, ignoring any duplicates.
Code:
Output:
Explanation:
The set my_set has five unique elements. When len() is applied to this set, it returns 5, reflecting the total number of unique elements. If there were any duplicate elements in the set, they would be ignored, and only unique elements would be counted.
How len() works for custom objects?
Python's built-in len() function calls a method named __len__() in our class. These special methods, having double underscores, are known as dunder methods as they have double underscores at the beginning and end of the method names. Implementing this method will allow the len() call to work in the class. If it is not implemented, the call to len() will fail with an AttributeError. Python’s built-in len() function calls its argument’s .__len__() method.
Output:
Explanation:
The above program has a class Order having count as an attribute. We have created a dunder function __len__() that returns the total count of orders passed as an argument. So we created an instance named myOrder and passed the count argument as seven, and when len() was called on myOrder, the value of count was returned.
Conclusion
- In this article, we learned how to use the len() function in python to determine the number of items in sequences, collections, and other third-party data types.
- The len() function in python takes an object as an argument and returns the length of that object.
- Python's len function can be used to obtain the length of
- built-in types,
- third party data types (like pandas and numpy),
- user defined classes (by implementing the __len__() method