*args and **kwargs in Python
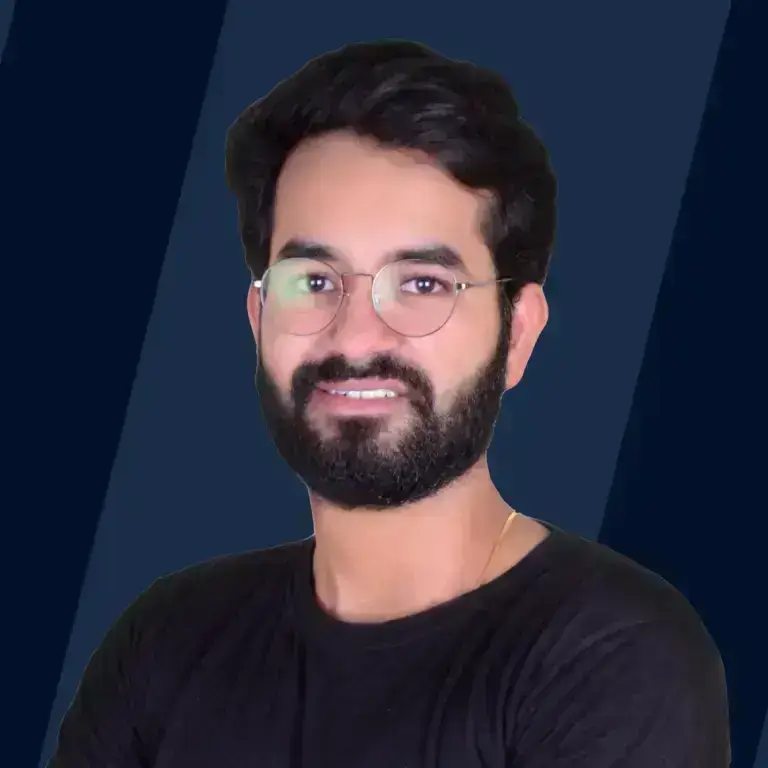
*args specifies the number of non-keyworded arguments that can be passed and the operations that can be performed on the function in Python, whereas **kwargs is a variable number of keyworded arguments that can be passed to a function that can perform dictionary operations.
Python *args
Let us assume that you are building a calculator, which only performs multiplication.
You can make a simple function that can do this, and that function will look like this-
Output:
Now, you want to multiply three numbers, so you have to make a new function.
Output:
You can already see the problem. If you keep going on like this, you will end up doing a lot of functions.
*args can save you the trouble here.
You can use args to solve this problem in a simple and flexible piece of code-
Output:
Now that your problem is solved, let’s understand what is going on here.
Python has *args, which allows us to pass a variable number of non-keyword arguments to a function. Non-keyword here means that the arguments should not be a dictionary (key-value pair), and they can be numbers or strings.
One thing to note here is that "args" is just an identifier. It can be named anything relevant.
When an asterisk(*) is passed before the variable name in a Python function, then it understands that here the number of arguments is not fixed. Python makes a tuple of these arguments with the name we use after the asterisk(*) and makes this variable available to us inside the function. This asterisk(*) is called an “unpacking operator”. We will learn more about it further on in the article.
This variable is then available to us inside the function, and we can use it to perform any operation we want. In our case, we iterated (looped) over it and calculated the product of all the numbers.
Python **kwargs
*args enable us to pass the variable number of non-keyword arguments to functions, but we cannot use this to pass keyword arguments. Keyword arguments mean that they contain a key-value pair, like a Python dictionary.
**kwargs allows us to pass any number of keyword arguments.
In Python, these keyword arguments are passed to the program as a Python dictionary.
Python will consider any variable name with two asterisks(**) before it as a keyword argument.
Output:
In the makeSentence function, we are iterating over a dictionary, so we have to use values() to use the values. Otherwise, it will only return the keys and not the values.
Another example of how kwargs can be used is given below-
Output:
In this code, we have used .items() because we want to get both the key and the value.
Using Both *args and **kwargs in Python to Call a Function
Now that you have understood *args and **kwargs, you might want to design a function that uses both of them. While doing this, the order of the arguments matters; *args have to come before *kwargs.
So, if you are using standard arguments along with *args and **kwargs, then you have to follow this order-
- Standard Arguments
- *args
- **kwargs
A simple example of a function that uses standard arguments, *args and **kwargs in Python:
Output:
Using Both *args and **kwargs in Python to Set Values of Object
When it comes to object-oriented programming, Python provides a flexible approach to initialize objects using *args and **kwargs. These two constructs allow developers to create functions and classes that can accept a variable number of arguments and keyword arguments, respectively.
Consider a simple class representing a geometric shape, like a rectangle.
- We can use *args to allow for flexibility in the number of arguments during object creation.
- We can use **kwargs to handle additional properties of the rectangle, such as colour or label.
Output:
Packing and Unpacking Using Both *args and **kwargs in Python
The single and double asterisks that we use are called unpacking operators.
Unpacking operators are used to unpack the variables from iterable data types like lists, tuples, and dictionaries.
A single asterisk(*) is used on any iterable given by Python.
The double asterisk(**) is used to iterate over dictionaries.
Let’s take some examples-
Output:
Here, the asterisk(*) passed before carCompany unpacked all the values. In other words, the values are printed as separate strings rather than a list.
Output:
Here, the double-asterisk unpacked the key-value pairs inside the mergedStack variable, and thus, we get all the key-value pairs in the mergedStack variable. args and kwargs in Python do the same job of unpacking the contained values, but they take different inputs and outputs.
Learn more about Packing and Unpacking Arguments in Python on Scaler Topics.
Conclusion
After learning about *args and **kwargs in Python, you can now successfully utilize their superpowers.
Here are some key points that will help you-
- *args and **kwargs are special Python keywords that are used to pass the variable length of arguments to a function
- When using them together, *args should come before **kwargs
- The words “args” and “kwargs” are only conventions. You can use any name of your choice