Variable Length Argument in Python
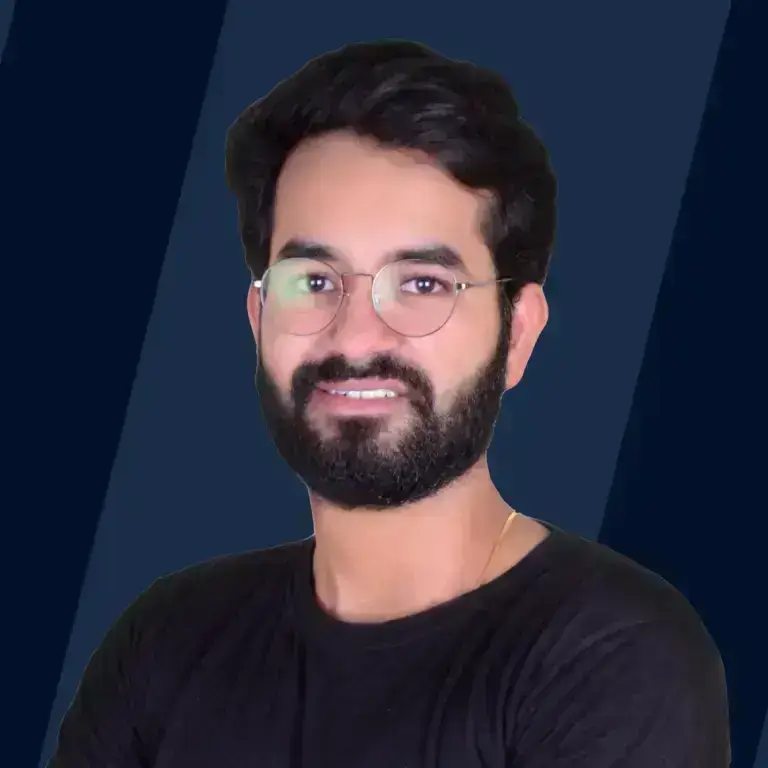
Overview
Several methods have no arguments, while many other methods tend to have a large number of them. There are instances when we have methods with arguments we are unaware of. We wish to provide a versatile API to sure other developers, or because we are unaware of the size of the data received as input, we may have a random number of arguments. We can write Python functions that accept any number of arguments.
This section will look at defining and using methods with variable length arguments. These methods can take an undetermined quantity of data in consecutive entries or named parameters.
Introduction to Variable-length Arguments in Python
Variable-length arguments, abbreviated as varargs, are defined as arguments that can also accept an unlimited amount of data as input. The developer doesn't have to wrap the data in a list or any other sequence while using them.
There are two types of variable-length arguments in Python-
- Non - Keyworded Arguments denoted as (*args)
- Keyworded Arguments denoted as (**kwargs)
Let's understand the concept with the help of an example. In the given Python program below, let's call a method and send two values to it, which are of integer data type, and the method returns the total sum.
Consider the following scenario: If we want to construct a new sum function that can accept any number of parameters (suppose 2, 5, 4, ...). The limitation of the above sum function is that it can only accept two arguments.
This is where variable-length arguments come into the picture. We can write methods in Python that take more than two arguments. Let's discuss how we can use multi-argument functions using the concept of variable-length arguments.
The syntax for writing a method that can take variable-length arguments is as follows:
Where func denotes the function's name, the argument args denotes a list of variable-length parameters.
We can use lists if we want to take more than two arguments as input. While this works, it is better to enclose the integers in a list, which is more complicated than when we had two or three defined arguments. With variable-length arguments, we can have the best of both worlds.
Varargs are defined in Python via the args syntax as discussed above.
Let's try writing the sum code including the args concept:
Code:
Output:
Using variable-length arguments in python, we can use more than two or more arguments while writing our programs.
Non - Keyworded Arguments (args)
Let's suppose we don't know how many arguments can be supplied to a function; the args are a feature of Python that allows us to send a variable number of non-keyword arguments to a method.
To provide variable-length parameters, we need to use an asterisk before the parameter name in the given method. The type of parameters supplied is a tuple, and within the method, these passed arguments form a tuple with the same name as the parameter, excluding the asterisk.
Features of args
- *args defines the number of non-keyworded arguments, and we can perform the operations on the tuple.
- It makes the function flexible.
Syntax of args
The syntax for a method with non-keyword variable arguments:
Before the variable name that carries the contents of all non-keyword variable parameters, an asterisk (*) is put. The tuple is empty if no extra arguments are supplied during the function call.
Example: Using * args to pass the variable-length arguments to the function and finding the multiplication
Code:
Output:
We utilized num as a parameter in the preceding program, which allows us to give a variable length argument list to the multiplier() function. We have a loop inside the method that multiply the input and prints the result. As a parameter to the function, we passed three separate tuples of varying lengths.
Keyworded Arguments (kwargs)
Python uses args to provide a variable-length non-keyword argument to a function, but it cannot be used to pass a keyword argument. kwargs, a Python solution for this problem, is used to pass the variable length of keyword arguments to the method.
To indicate such an argument, we need to use a double asterisk before the parameter's name in the method. Arguments are supplied in the dictionary, which creates a dictionary within the method with a name similar to the parameter except for double asterisk **
Features of kwargs
- **kwargs is responsible for passing a variable number of keyword arguments dictionary to the method, allowing it to perform dictionary operations.
- It is known as kwargs because of the double star. The double star allows us to send keyword arguments through (and any number of them). When you pass a variable into a function as a keyword argument, you give it a name.
- The kwargs can be thought of as a dictionary that maps each term to the value we pass along with it, which is why there doesn't appear to be any sequence in which the kwargs were printed out when we iterate over them.
Example:
Code:
Output:
Using args and kwargs to Call a Function
We can also use *args and **kwargs to pass arguments into functions.
First, let’s look at an example with *args.
Code:
The variables arg_1, arg_2, and arg_3 are defined in the code above. We will be printing the arguments. We next create a variable that is set to an iterable. Since we are using args, we will use tuples and asterisk syntax to send that variable into the method.
Output:
We can use the ** kwargs parameters to call a method similarly. We'll create a variable that's a dictionary with three key-value pairs (we'll call it kwargs here, although it can be anything you like) and feed it to a method with three arguments:
Code:
Output:
Unpacking Arguments with *args and **kwargs
We can use the * operator to unpack the list so that all of its elements can be provided as separate parameters.
Code:
Output:
It's important to remember that the number of arguments must equal the length of the list we're unpacking for the arguments.
We can use the ** operator to unpack the dictionary so that all of its elements can be provided as separate parameters.
Code:
Output:
For the dictionary, ** used with it was unpacked here, and the elements in the dictionary were provided to the function as keyword arguments. As a result, writing "func1(**dim)" was the same as writing "func1(x=1, y=2, z=3)."
Conclusion
- In this post, we learned what variable-length parameters are in Python and how to use them in our function.
- We generally use args and kwargs as arguments when we are not sure about the total number of arguments we need to pass in the methods.
- The * args function receives several arguments in the form of tuples, whereas the (** kwargs) function is known to Receive multiple keyword arguments in the form of a dictionary.