Python Generators
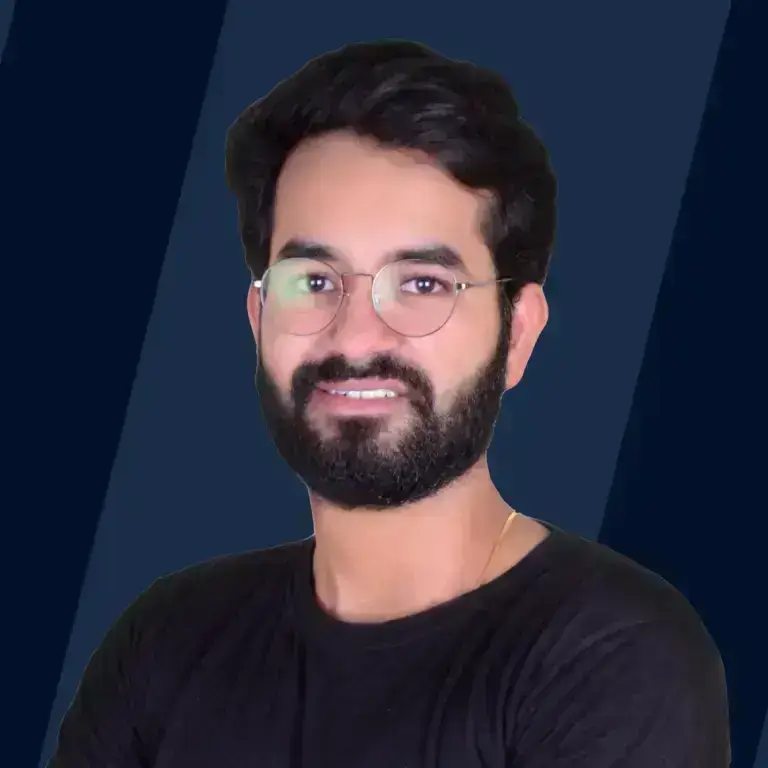
Overview
Generators in Python are used to create iterators and return a traversal object. It helps in traversing all the items one at a time with the help of the keyword yield.
What are Python Generators?
Python's generator functions are used to create iterators(which can be traversed like list, tuple) and return a traversal object. It helps to transverse all the items one at a time present in the iterator.
Generator functions are defined as the normal function, but to identify the difference between the normal function and generator function is that in the normal function, we use the return keyword to return the values, and in the generator function, instead of using the return, we use yield to execute our iterator.
Example:
In the above example, gen_fun() is a generator function. This function uses the yield keyword instead of return, and it will return a value whenever it is called.
Yield vs Return
Yield | Return |
---|---|
It is used in generator functions. | It is used in normal functions. |
It is responsible for controlling the flow of the generator function. After returning the value from yield, it pauses the execution by saving the states. | Return statement returns the value and terminates the function. |
Difference Between Generator Function & Normal Function
- In generator functions, there are one or more yield functions, whereas, in Normal functions, there is only one function
- When the generator function is called, the normal function pauses its execution, and the call is transferred to the generator function.
- Local variables and their states are remembered between successive calls.
- When the generator function is terminated, StopIteration is called automatically on further calls.
Python Generators With a Loop
The Generator functions are also used for the loop.
Example:
Output:
The function seq will run 10 times, and when seq is called using next for the 11th time, it will show an error StopIteration.
But when using for loop, instead of next.
Output:
It will not show any error. for loop automatically handle the exception statements.
How To Create Generator Function in Python?
In Python, there are some ways to create a generator function.
- Using a Yield statement
- Using Python Generator Expression
1. Yield Statement
Yield keyword in Python that is used to return from the function without destroying the state of a local variable. We have already discussed how to create a generator function using yield.
2. Python Generator Expression
Generator Expression is a short-hand form of a generator function. Python provides an easy and convenient way to implement a Generator Expression.
According to its definition, they are similar to lambda function as lambda function is an anonymous function, and generator functions are anonymous.
But when it comes to implementation, they are different, they are implemented similarly to list comprehension does, and the only difference in implementation is, instead of using square brackets('[]'), it uses round brackets('()').
The main and important difference between list comprehension and generator expression is list comprehension returns a list of items, whereas generator expression returns an iterable object.
Example:
Output:
(i for i in range(x) if i % 2 == 0) in this generator expression, the first part is the yield part, and next is the for loop, followed by the if statement.
Use of Generators in Python
1. Easy to Implement:
Generator functions are easy to implement as compared with iterators. In iterators, we have to implement iter(), __next__() function to make our iterator work.
2. Memory Efficient:
Generator Functions are memory efficient, as they save a lot of memory while using generators. A normal function will return a sequence of items, but before giving the result, it creates a sequence in memory and then gives us the result, whereas the generator function produces one output at a time.
3. Infinite Sequence:
We all are familiar that we can't store infinite sequences in a given memory. This is where generators come into the picture. As generators can only produce one item at a time, so they can present an infinite stream of data/sequence.
Output:
Conclusion
- Generator functions in Python are used to create iterators and return an iterator object.
- In the generator function, we use a yield statement instead of a return statement.
- We can use multiple yield statements in the generator function.
- When iterating over the generator function using next, in the end, it will show up StopIteration error, whereas when we use the for loop, it doesn't show any error.
- Generator Expressions are anonymous functions. They are implemented as similar to a list comprehension, and the only difference is that they use round parentheses instead of square parentheses.