Remove Key From Dictionary Python
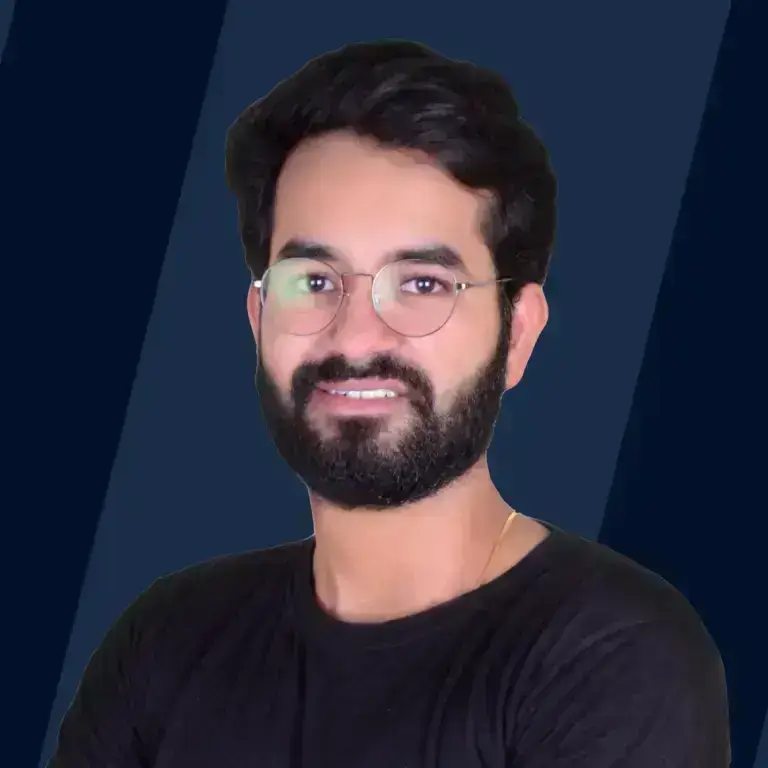
Overview
This article discusses various methods to remove a given key (along with its associated value) from a dictionary in Python using various dictionary class methods in Python.
Introduction
Suppose you have a dictionary in Python (that is essentially a collection of key-value pair mappings) that you use to store the names of the students in your class using their roll numbers as keys.
For example:
Now, imagine that some of your students have to leave your subject or they want to change their school. In such a scenario, you must remove their information from the records of your current students. In other words, you need to remove the key from your dictionary that represents the roll number of the leaving student.
You can perform this action, that is, remove key from dictionary in Python, in a number of ways, all of which we describe below.
Method 1 - Using pop() to Remove Key from Dictionary
You can remove key from dictionary in Python using the pop() method on a dictionary object, say dict. It removes the specified key (and associated value) from the dictionary and returns the value associated with the key.
Syntax:
Please note:
- All the forms of the pop() method remove the key-value pair for the specified key, and return the value.
- The second parameter, defaultVal is not compulsory. It is returned when the key passed is not found in the dictionary.
- If the second parameter is not specified and key is not present in the dictionary (on which the .pop() method is called), KeyError will be raised.
- Other viable values that may be passed as the second parameter are None, -1, 0, etc.
Code Example 1:
Output:
Explanation:
- Since we passed 1601 as the key parameter, and it was present in the dictionary, it was removed successfully.
- Next, when we removed 1603, we captured the return value in removedName so that we may verify the removed key-value pair.
- Next, we passed a default value that is to be returned when the key that we want to delete, is not present in the dictionary. Since the key 1600 does not exist in dict, we get "No such roll number found" string as the output.
Code Example 2:
Output:
Explanation: As stated earlier, since the key 1600 does not exist in the dictionary, we get KeyError.
Method 2 - Using pop() with Try/Except Block
Try-except blocks are used to handle exceptions that may occur while executing a block of code. A block of code that is to be executed is surrounded by a try block. The code that should be executed in case an exception is encountered, is placed in the except block. You may learn more about try-except blocks here.
To remove key from dictionary in Python using try-except block, we use the following syntax:
Syntax:
Code Example 1:
Output:
Explanation: The program tried to delete the key 1600 from the dictionary. But because the key was not present in the dictionary, a KeyError exception was raised that was caught by the except block, and the code present in it was executed.
Please note that you can specify the exception in the code as follows:
For the above example, both the code snippets are equivalent to remove key from dictionary in Python.
Method 3 - Using items() Method with For-Loops
We can use the items() method with a for-loop to remove a key from dictionary in Python. We simply iterate over our current dictionary and copy all key-value pairs (except the key to be deleted) to our new dictionary. Finally, we assign this new dictionary to our old dictionary variable.
To remove key from dictionary in Python using items method, we use the following syntax:
Syntax:
Code Example 1:
Output:
Explanation: While iterating over the dictionary, we copied all the key-value pairs into our temporary dictionary temp_dict, except the key to be deleted. Finally, we assigned our dict variable to the new dictionary that does not contain the deleted key.
If the target key was not present in the dictionary, this procedure would have essentially just copied the entire dictionary, and no change would be observed.
Method 4 - Using items() Method & Dictionary Comprehensions
Dictionary comprehension is a way to iterate over a dictionary without using loops. You can learn more about comprehensions here.
Here too, we just copy the key-value pairs (other than the key to be deleted) into our own dictionary by reassignment.
To remove key from dictionary in Python using items method and dictionary comprehensions, we use the following syntax:
Syntax:
Code Example 1:
Output:
Explanation: While using dictionary comprehensions, we constructed a dictionary that did not contain our target key to be deleted and assigned our variable dict to the new dictionary created.
Method 5 - Using del keyword
We can use the del keyword to remove key from dictionary in Python. The del keyword simply removes the reference of the variable, so that it is no longer occupied in the memory.
If the key is not present in the dictionary, then the program would raise the KeyError exception.
To remove key from dictionary in Python using del keyword, we use the following syntax:
Syntax:
Using the above statement, we can delete the key from dictionary in Python.
Code Example 1:
Output:
Explanation: Since the key 1602 was present in the dictionary, therefore using del dict[key] removed the key from the dictionary.
Code Example 2:
Output:
Explanation: Since the key 1604 is not present in the dictionary, using del dict[1604] raises a KeyError exception.
Method 6 - Using the del Keyword with Try-Except
We can use the del keyword in combination with try-except blocks to handle the case when the key we were trying to remove is not present in the dictionary.
Thus we can use del along with try-except to remove key from dictionary in Python.
To remove key from dictionary in Python using del keyword and try-except blocks, we use the following syntax:
Syntax:
Code Example 1
Output:
Explanation: Since the key 1604 was not present in the dictionary, using the del keyword raised a KeyError exception. Thus, the control of the program shifted to the except block where the print statement was executed to give the shown output.
Level up your programming game with our Python course - the ultimate path to mastering one of the most in-demand languages in the tech world.
Removing All Keys Using clear() Method in Python Dictionary
We can use the clear() method of the dictionary class to remove all the keys (and associated values) from a given dictionary.
Please Note: Using clear() method on a dictionary results in an empty dictionary, not a None object. See the example below.
To remove key from dictionary in Python using clear() method, we use the following syntax:
Syntax:
Code Example:
Output:
Explanation:
- Calling clear() method on the dictionary dict deletes all the key-value pairs present in that dictionary.
- Notice that clear() results in an empty dictionary, not a None object.
Removing All Keys Using popitem() in Python Dictionary
You can use the popitem() method to remove and return keys in LIFO (last in first out) order from a dictionary in Python. Calling this method until the Python dictionary is empty results in removing all keys from the dictionary.
To remove key from dictionary in Python using popitem() method, we use the following syntax:
Syntax:
This results in dict being an empty dictionary (and not a None object).
Code Example 1:
Output:
Explanation: Using popitem() removes key-value pairs in LIFO fashion. After all such pairs have been removed from the dictionary, the dictionary becomes empty as can be seen from the output.
Removing Multiple Keys from Python Dictionary
If you want to remove multiple keys from a dictionary in Python, you can do so iteratively.
You can store the keys you want to remove in a list and call the pop() method for each element of the list.
Syntax:
Code Example:
Output:
Explanation: Calling the pop() method on the dictionary dict iteratively removes all the keys that were present in the list keys_to_remove.
Python pop() vs del Keyword
Both pop() method and del keyword can be used to remove key from dictionary in Python (as we saw above). The only difference between these two methods is that using the del keyword doesn't return the removed key.
That is, the following line of code to remove a key in Python dictionary would be invalid:
Since the pop() method returns the value of the key that was removed, the following lines of code are valid in Python:
Conclusion
- Python dictionary helps to store key-value pair mappings that are iterable and indexable using keys.
- A given key (and its associated value) can be removed from Python dictionary using the pop() method, which also returns the value removed. It can be surrounded by a try-except block to catch the KeyError exception.
- Another method to remove a key from Python dictionary is using the del keyword. This method does not return the value removed from the dictionary. It may be surrounded by a try-except block to catch a KeyError exception if it occurs.
- A key-value pair can also be removed by copying the rest of the dictionary into a new one while iterating over the dictionary using either a for-loop or dictionary comprehension.
- Multiple keys can be removed from a Python dictionary using either the popitem() method or the pop() method in a loop.
- The clear() method can be used to delete all the key-value pairs in the dictionary.