Reverse a String in Java
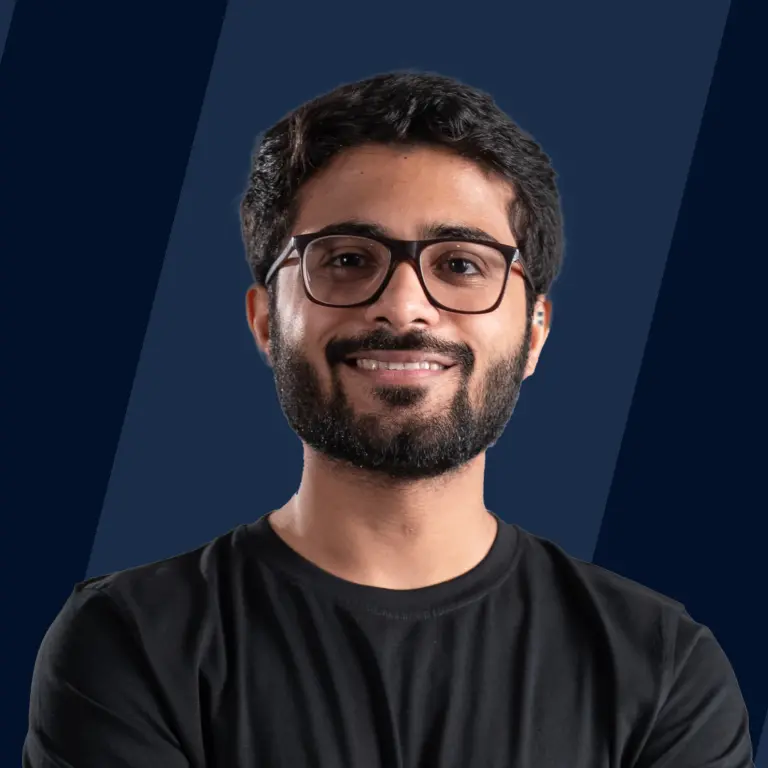
This tutorial demonstrates how to reverse a string in Java, useful for palindrome checks. For instance, reversing "Scaler" results in "relacS", showing character inversion.
A classic palindrome example is "aibohphobia", humorously referring to the fear of palindromes, illustrating a string that reads the same backward and forward. Now, let's discuss the different methods of reversing a string in Java.
Pre-requisites: Difference between StringBuffer and StringBuilder
By Extracting Each Character and Adding in Front of the Existing String
Reversing a string in Java by extracting each character and adding it in front of the existing string is a straightforward approach. This method iterates through the input string, takes each character, and places it at the beginning of a new string. The process effectively reverses the order of characters.
Approach:
- Initialize an empty string to store the reversed result.
- Iterate through the input string from the first to the last character.
- For each character, add it in front of the current result string.
- After the loop completes, the result string will be the reversed version of the input string.
Code:
Output:
This method is simple and intuitive, but it's worth noting that string concatenation in a loop like this can be inefficient for very long strings due to the way strings are handled in Java. Each concatenation creates a new string object, so for more performance-critical applications or large strings, using a StringBuilder or a similar approach might be more suitable.
By Using getBytes() Method
The getBytes() method in Java can be utilized to reverse a string by converting the string into a byte array, then manipulating the array to reverse the order of elements. This method offers a byte-level approach to reversing strings, providing a more fundamental operation on the string's binary data.
Approach:
- Convert the input string to a byte array using getBytes().
- Create a temporary byte array of the same size.
- Copy elements from the original byte array to the temporary array in reverse order.
- Convert the reversed byte array back to a string.
Code
Output:
This method effectively reverses the string "SCALER" to "RELACS", showcasing a practical application of byte manipulation to achieve string reversal in Java.
By Using reverse() Method of the StringBuilder Class
StringBuilder is a class in Java. It has an in-built method reverse(). This function reverses the characters in the string.
Code
- StringBuilder objects are mutable. So we have created an object “obj” of the StringBuilder class.
- Next, we are appending the input string “str” using the append() method.
- The reverse() is then called using obj & the result is returned.
By Using toCharArray()
Reversing a string in Java using the toCharArray() method involves converting the string into an array of characters, then iterating over this array in reverse order to construct the reversed string. This approach provides a simple and straightforward way to manually reverse a string by accessing its individual characters.
Approach:
- Use the toCharArray() method to convert the input string into a character array.
- Iterate over the character array in reverse order.
- Append each character to a StringBuilder or concatenate to a new string to form the reversed string.
Code:
Output:
By using ArrayList objects
Using ArrayList objects to reverse a string in Java involves converting the string into a collection of characters, reversing the collection, and then constructing the reversed string from the collection. This method leverages the Collections.reverse() utility function for reversing, which provides a straightforward way to reverse any List.
Approach:
- Convert the input string into a char array using the toCharArray() method.
- Create an ArrayList<Character> and populate it with the characters from the array.
- Use Collections.reverse() to reverse the order of elements in the ArrayList.
- Build the reversed string from the reversed ArrayList.
Code:
Output:
By Using StringBuffer Class
Using the StringBuffer class is another efficient method to reverse a string in Java. StringBuffer is a thread-safe, mutable sequence of characters with a built-in method to reverse the sequence. This approach is particularly useful when working with strings that require synchronization in multithreaded environments.
Approach:
- Create an instance of the StringBuffer class and initialize it with the input string.
- Utilize the reverse() method provided by the StringBuffer class to reverse the string.
- Convert the StringBuffer object back to a string if necessary.
Code:
Output:
This example demonstrates the simplicity and effectiveness of using the StringBuffer class for reversing strings in Java. The reverse() method directly manipulates the internal character sequence of the StringBuffer object, providing an in-place reversal that is both fast and straightforward.
By Taking Input from User
To reverse a string in Java by taking input from the user, you typically use a Scanner object to read the user's input and then apply a reversing technique. Here's an approach using a StringBuilder to reverse the input string for simplicity and efficiency.
Approach:
- Create a Scanner object to read the user input.
- Read the user's input string using Scanner.nextLine().
- Utilize StringBuilder to reverse the string as it provides a built-in reverse() method.
- Print the reversed string.
Code:
Output:
This example demonstrates taking a string input from the user and reversing it. The StringBuilder.reverse() method provides a straightforward way to reverse strings, making it an ideal choice for this operation.
By Using Stack
Reverse a string in Java can be efficiently done using a stack due to its Last-In-First-Out (LIFO) property. This approach involves pushing each character of the string onto a stack and then popping them off, which naturally reverses the order.
Approach:
- Convert the input string into a character array.
- Create a Stack<Character> to hold the characters.
- Iterate over the character array and push each character onto the stack.
- Pop each character from the stack and append it to a StringBuilder to form the reversed string.
- Print or return the reversed string.
Code:
Output:
This example showcases the use of a stack to reverse a string in Java. By leveraging the stack's LIFO behavior, each character is read in its original order but output in reverse, achieving the string reversal efficiently.
Conclusion
- Java provides several efficient techniques for reversing strings, vital for applications like palindrome checking.
- Techniques range from simple character extraction and addition to utilizing data structures like stacks, and employing built-in methods of the StringBuilder and StringBuffer classes.
- Each method varies in implementation, with some offering more straightforward approaches like the StringBuilder.reverse() method, while others, like using stacks, offer a deeper understanding of data manipulation.
- The choice of method depends on the specific requirements of the task, including considerations for time and space efficiency, with some methods like StringBuilder and StringBuffer being particularly recommended for their balance of simplicity and performance.
Now, get coding and write your very own Java program to reverse a string!