JavaScript Array slice() Method
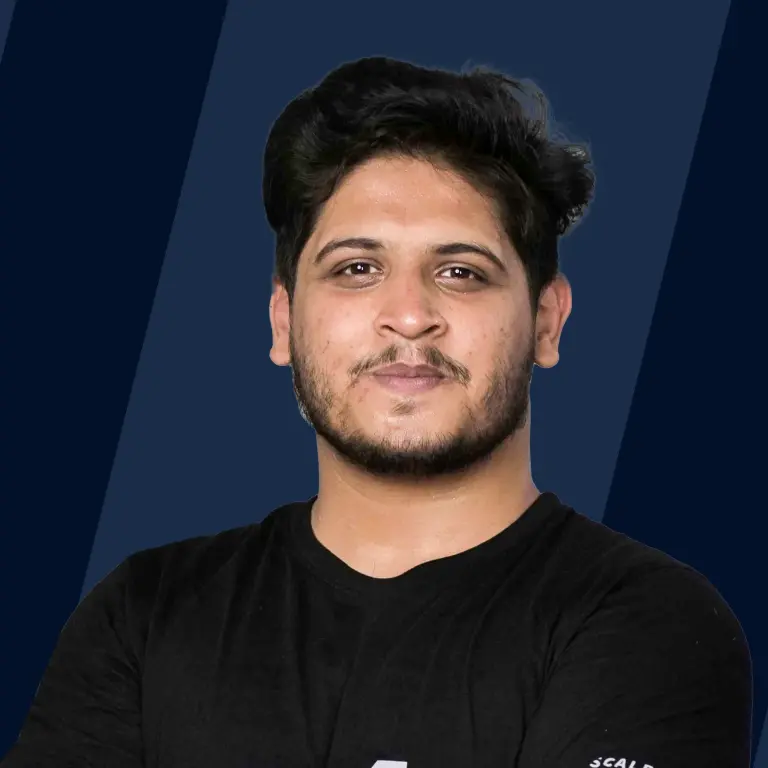
Overview
The slice() function in Array class of Javascript returns a shallow copy of a subarray into a new array object which includes the elements from a user-specified start index to a user-specified end index (end not included). The original array is not modified.
JavaScript Array slice() Syntax
There are multiple syntaxes for the function slice in javascript, and we shall see all of them one by one.
1. Without Any Parameter
This will make a copy of the original array arr and store it in the array brr as both start, and end have not been defined.
2. With One Parameter
This will include the elements starting from the start index (including start) till the end of the array ar and store all these in a new array in br.
3. With Two Parameters
This will include the elements starting from the start index (including start) till the index end (the element at the end index will not be included) of the array ar and store all these in a new array in br.
JavaScript Array slice() Parameters
The function slice in javascript either accepts no parameter, one parameter, or two parameters. Let us discuss in detail what each of these means and does.
There can be at most two parameters in the function slice in Javascript. These are :
- Start:
- This indicates the starting index from where the new array object will be created. Note that this start index will be included and will be the first one in the new array.
- This value is optional, so it isn't necessary to specify it. In case the value is not specified, 0 is taken as the default value. If a negative value is used, then it is treated as taking elements from the end of the array.
- End :
- This indicates the ending index to which elements from the array will be included in the new array object. Note that this end index will not be included, and hence the element before this index will be the last one in the new array.
- This value is optional, so it isn't necessary to specify it. In case the value is not specified, the length of the array is taken as the default value. If a negative value is used, then it is treated as taking elements from the end of the array.
JavaScript Array slice() Return Values
Return Type: new Array object
Whenever we call the array slice in JavaScript, it is going to return a new array object, which contains the elements from the specified start index to the specified end index (not including the end index).
In case both these values are not specified, the new array will contain all of the values of the original array.
Let us take a look at an example to understand this better,
Output :
JavaScript Array slice() Example
Let us look at a simple example to see how to use the slice in Javascript.
Output :
Explanation:
In the example above, initially, we had an array of countries. To extract Asian countries from this array, we used the slice() method in Javascript. As we wanted to extract the subarray between the elements 0 and 3 (including both), we gave the start and end parameters as 0 and 4, respectively (as the end index is not included in the newly created array). You can also see that there was no change to the original countries array.
What is Array slice() in JavaScript?
Consider a case where you want to store the subset of an already existing array in a different array. The two options we have is either manually creating a new array (which can be very time-consuming depending upon the size of the sub-array we want to extract) or we could run a for a loop. But, in Javascript, the Array.prototype object provides us with a very useful method that can accomplish this same task by just invoking it.
The array slice in JavaScript extracts a continuous subarray and places it in a new array. So, the original array is not modified in any way. It does so by taking two indices - start and end. So, the elements starting from the start index and ending at the end index (not including the end) are stored in a new array.
More Examples
Let us look at some more examples to understand the varied uses of the slice in Javascript.
1. Return a Portion of an Existing Array
We can also use the function slice in JavaScript to copy a portion of the array. Let us look at an example.
Output :
Explanation: In this example, we copied the subarray of the marks array from index 4 to the end of the array. As the no end parameter was defined, the end of the array was taken as the default, and hence, all elements from the 4th index to the end were copied to the array end_marks.
2. Copy Complete Array Using slice()
We can also use the slice in javascript function to copy the complete contents of an array to another array.
Output :
Explanation: In this example, as no parameters were given to the slice() function, the default values for both were taken. This means start was taken as 0 and end as the length of the array. So, the elements from the 0th index to the last index were copied to the new array, which means all the elements were copied.
3. Array-like Objects into Arrays
We can also use the slice in Javascript to convert any array-like objects into arrays without having to manually create an array. Let us look at an example.
Output :
Explanation: In this example, we passed individual digits to the function convert(). At this point, they were not an array of digits, but in the function, we used slice() to add all the digits passed as arguments into one array, and this array was returned and stored in the array digits.
Browser Compatibility
The slice method in javascript is supported by all the browsers such as Chrome, Edge, Safari, Opera, etc.
Conclusion
- The function slice in Javascript returns a subarray in the form of a new array object which includes the elements from a user-specified start index to a user-specified end index (end not included).
- The original array is not modified when we use the slice in Javascript.
- The function slice in Javascript takes two parameters as input: start and end, where start denotes the starting index and end denotes the ending index of the subarray to be copied.
- The slice() function in Javascript has three syntaxes:
- No parameters.
- One parameter.
- Two parameters.
- Theslice function in Javascript returns a new array that contains the elements between the specified indexes starting and ending from the original array.
See Also
- splice() in javascript
- call() in javascript
- bind() in javascript