Python math.sqrt() Method
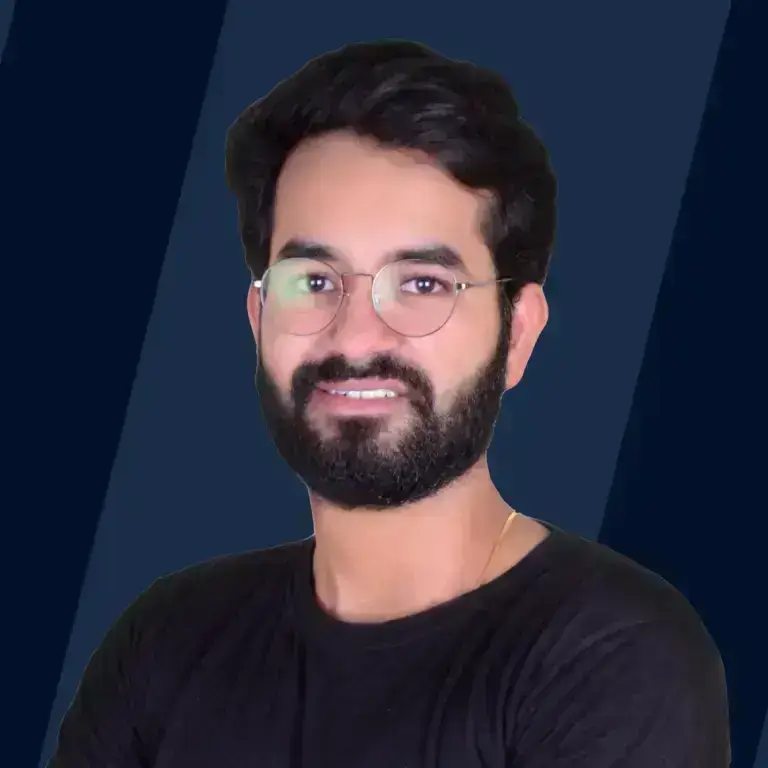
The sqrt() in Python is a built-in function specifically designed for finding the square root of numbers. As a fundamental square root function in Python, it requires numbers greater than or equal to zero as input.
For example, to use this python square root function, you would call sqrt(4) to get 2.0 as the result, demonstrating its straightforward application. Remember to import the math module with from math import sqrt to utilize this function in your Python code.
What is math.sqrt() Function?
The math.sqrt() function in Python is a mathematical operation within the math module that calculates the square root of a specified number. To use this function, you first need to import the math module using import math.
It's a precise and efficient way to compute square roots, crucial for various mathematical computations. For instance, math.sqrt(25) returns 5.0, showcasing its utility in finding the square root of 25. This function is essential for tasks requiring python square root calculations, making it a staple in the toolkit of developers and data scientists alike.
math.sqrt() Method Syntax
The syntax of sqrt() in python is as below:
where you first import the math module and then you can start using the sqrt() in python. The parameter 'n' in the syntax is any number more than or equal to zero.
Quick Tip − The sqrt() function in python cannot be used directly while writing the code. Hence, to call the sqrt() function in python, we first need to import the math module. Then, to execute this function we need to start by calling the sqrt function in python using the math static object.
math.sqrt() Method Parameters
The parameters of sqrt() in python are as below:
n- To find the square root of any number we need to specify the number in the parameter section.
We need to make sure that the number that we are passing as a parameter is always more than or equal to zero. If it is less than 0, then we may encounter will a ValueError. While if the value is not a number, it will output a TypeError.
math.sqrt() Method Returns
The return values of sqrt() in python is as follows:
The output returned from the square root of the number is a floating-point value for that number that is being passed in the parameter. For example, the square root of 25 would be 5.0 and not 5 as an output.
Examples of sqrt() in Python
Now as we have understood the concepts around the sqrt in python, let us start practicing it with a few code examples as listed below:
checking if a number is prime or not
The below code explains how we can make use of the sqrt() in python to calculate if a given number that is entered by the user is prime or not.
Code:
Output:
using sqrt() function to find the hypotenuse of a triangle
To find the hypotenuse c of a right-angled triangle using the sqrt() in python, apply the Pythagorean theorem: c = sqrt(a^2 + b^2), where a and b are the lengths of the other two sides. Use from math import sqrt to access this function.
Code:
Output:
The square root of zero
Code:
Output:
Explanation: As seen above, we are demonstrating the python square root of Zero by utilizing the square root function in Python we familiarized ourselves with earlier. We imported the math module to access this square root function in Python, highlighting its utility for such mathematical operations.
Square root of negative numbers
Code:
Output:
Explanation: As studied above for any number less than 0 or a negative number, the square root function in python for that number comes out to be an ERROR as we got for the above code where we were trying to sort import the math module to use the square root of the number -9. And once the code was executed we get a ValueError: math domain error .
sqrt() Function Error
When using the sqrt() function in Python to calculate the square root of a negative number, it results in a ValueError because square roots of negative numbers are not defined within the real number system. Here's an example demonstrating this error:
Output:
This output indicates that the operation failed due to the input being outside the valid domain for the sqrt() function. To avoid this error, ensure the input is always zero or a positive number.
Conclusion
- An sqrt() in python is an inbuilt function that helps to find the square root of any number that is greater than or equal to zero.
- If you use the sqrt in python to find the square root for any negative number you shall encounter a Value Error.
- While if the value is not a number, it will output a TypeError.
- The output returned from the square root of the number is a floating-point value for that number that is being passed in the parameter eg 5.0 not 5.
- Various scenarios covering the concepts around sqrt() in python were covered as learned in the above code examples.